Lab 03: Indexing and Relevance Ranking on Geo-Texts#
In this tutorial, we will experiment on checking/implementing some information retrieval techniques on a geo-text. Most of these techniques like R-tree, TF-IDF, relevance ranking, cosine similarity, etc. have been covered in the lecture. Techniques that you have learnt in Lab 1 and 2 (e.g., geopandas, osm, etc.) will be used/enhanced in this tutoiral. Meanwhile, new techniques such as wordcloud will also be introduced.
A summary of packages you will be using:
Okay, now let’s discuss first in a very general sense what we want to achieve in this tutorial. The goal is to understand the (functional) characteristics of regions in the City of Bristol. To do it, we use the points of interest and their types (e.g., restaurant, school, police office) as a proxy to quantify such a “functional” characteristics. Namely, if a region has a lot of restrants and bars, it might be more likely to be characterized as a food/entertainment region. It is then clearly different from another region where most points of interet are types of, for instance, school and nursery.
More interestingly, we do not only want to qualitatively describe these regions, we also need a more quantitative method so that computers can also understand it and then retrieve information for us more accurately and effectively.
Part 1: Load the local regions (Lower Super Output Areas 2011) for the City of Bristol#
First of all, let’s load the region data about Bristol. There are many ways to delineate the City (e.g., by administrative office or by local citizens’ perception/activity). In this tutorial, we use the data called Lower Super Output Areas 2011. It is created for the collection of census data. “Lower Super Output Areas (LSOAs) are a national geography for collecting, aggregating and reporting statistics. They were designed to improve the reporting of small area statistics and are built up from groups of Output Areas”. You can download the data from the Open Data Bristol portal or find the downloaded file from Blackboard. The code below then loads the data into Python and convert it to a geodataframe
.
Several tips:
replace the directory to where your data is downloaded.
../
in my code means going up one level in the directorysometimes, we can ignore the parameter
delimiter
inpd.read_csv
if the delimiter is by default comma. But here, since it is semicolon, we need to indicate it (you can try to see what kind of errors you will encourter if you do not include it)the raw data provide geomery information in a geojson format like
{"coordinates": [[[-2.5705649134167503, 51.408...
, to convert it to a format as well-know text, which is supported bygeopandas
/shapely
, we write a functionparse_geom
import geopandas as gpd
import pandas as pd
import json
from shapely.geometry import shape
def parse_geom(geom_str):
try:
return shape(json.loads(geom_str))
except (TypeError, AttributeError): # Handle NaN and empty strings
return None
bristol_lsoal_df = pd.read_csv('../../LabData/Lab3/lsoa110.csv', delimiter=';')
bristol_lsoal_df["geom"] = bristol_lsoal_df["geo_shape"].apply(parse_geom)
bristol_lsoal_gdf = gpd.GeoDataFrame(bristol_lsoal_df, geometry="geom")
print(bristol_lsoal_gdf.head())
OBJECTID LSOA11 Code LSOA11 Name LSOA11 Local name MSOA11 Code \
0 7 E01014612 Bristol 052B Fortfield East E02003063
1 8 E01014512 Bristol 016E Somerville Road E02003027
2 18 E01014618 Bristol 011C Henleaze North E02003022
3 36 E01014576 Bristol 019D Rose Green E02003030
4 24 E01033370 Bristol 054E Redcliffe South E02006887
Ward code Area square mteres Perimeter (m) MI_PRINX \
0 E05001989 250084.343750 3010.960449 2
1 E05001975 178468.437500 3093.555908 152
2 E05001990 324102.562500 4205.579590 921
3 E05001984 469484.093750 3586.286133 976
4 E05001995 126227.867188 2103.395020 19
geo_shape \
0 {"coordinates": [[[-2.5705649134167503, 51.408...
1 {"coordinates": [[[-2.5836634052406393, 51.476...
2 {"coordinates": [[[-2.610432616527603, 51.4856...
3 {"coordinates": [[[-2.5511455096576006, 51.463...
4 {"coordinates": [[[-2.5911512584061063, 51.445...
geo_point_2d \
0 51.409649589944884,-2.566230812955203
1 51.47614221648566,-2.588736496528701
2 51.489587737185744,-2.606558179420023
3 51.46845752679203,-2.5464321087549955
4 51.44681337159509,-2.5874479497333067
geom
0 POLYGON ((-2.57056 51.40811, -2.57048 51.40829...
1 POLYGON ((-2.58366 51.47624, -2.58344 51.47609...
2 POLYGON ((-2.61043 51.48565, -2.61012 51.48600...
3 POLYGON ((-2.55115 51.46378, -2.55102 51.46396...
4 POLYGON ((-2.59115 51.44567, -2.59098 51.44604...
Alternatively, you can load shapefiles (or geojson, geopackages formated data) using the read_file()
function of geopandas
, which will directly load the geo-referenced file as a geodataframe
. Note that you can download the LSOA_2011 of Bristol in different formats from the Open Data Bristol portal too.
bristol_shp_dir = '../../LabData/Lab3/LSOA_2011_(simplified)/LSOA_2011_(simplified).shp'
bristol_lsoal_gdf2 = gpd.read_file(bristol_shp_dir)
bristol_lsoal_gdf2.head()
OBJECTID | LSOA11CD | LSOA11NM | LSOA11LN | MSOA11CD | WARDCD | WARDNM | AREA_M2 | PERIMETER_ | Shape__Are | Shape__Len | geometry | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 1 | E01014485 | Bristol 023A | Mina Road | E02003034 | E05001972 | Ashley | 274649.437500 | 3500.999023 | 271234.427200 | 3345.873087 | POLYGON ((360647.016 174587.134, 360638.381 17... |
1 | 2 | E01033366 | Bristol 023H | St Pauls Grosvenor Road | E02003034 | E05001972 | Ashley | 92561.281250 | 1667.071045 | 91912.916122 | 1590.649592 | POLYGON ((359458.000 173935.000, 359464.000 17... |
2 | 3 | E01014486 | Bristol 023B | St Agnes | E02003034 | E05001972 | Ashley | 276066.531250 | 4337.872559 | 276957.510246 | 4153.567496 | POLYGON ((360249.713 174716.608, 360224.497 17... |
3 | 4 | E01014489 | Bristol 023D | Lower Montpelier | E02003034 | E05001972 | Ashley | 171743.171875 | 2755.843506 | 171822.423092 | 2655.816875 | POLYGON ((359628.089 174397.518, 359611.263 17... |
4 | 5 | E01014488 | Bristol 023C | Upper Montpelier | E02003034 | E05001972 | Ashley | 188000.250000 | 2753.252197 | 188759.246117 | 2636.044692 | POLYGON ((359628.089 174397.518, 359604.571 17... |
Now, even though bristol_lsoal_gdf
is created as a geodataframe
, we still want to check if the crs is set up already because sometimes we can easily forgot it. Then, .crs
can be used here (crs
is an attribute of geodataframe
):
bristol_lsoal_gdf.crs is None
True
Since there is no crs being set up in this example, we will assign a crs by using:
bristol_lsoal_gdf.crs = 'epsg:4326'
bristol_lsoal_gdf.crs
<Geographic 2D CRS: EPSG:4326>
Name: WGS 84
Axis Info [ellipsoidal]:
- Lat[north]: Geodetic latitude (degree)
- Lon[east]: Geodetic longitude (degree)
Area of Use:
- name: World.
- bounds: (-180.0, -90.0, 180.0, 90.0)
Datum: World Geodetic System 1984 ensemble
- Ellipsoid: WGS 84
- Prime Meridian: Greenwich
So, how do I know it is epsg:4326
?
As usual, we can easily plot the regions:
bristol_lsoal_gdf.plot()
<Axes: >
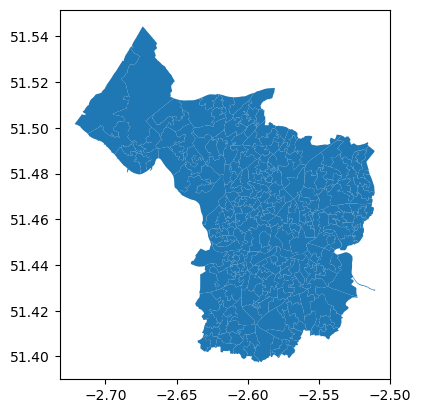
Part 2: Load in the Points of Interest (POI) from OSM#
Alright, now we have the regions in the City of Bristol. We next want to get the POIs in the City. There are many ways to get the POIs, many of which have been introduced in the lecture (e.g., Yelp and Foursquare). Since we have already used OSM, why not playing with the POIs provided from OSM?
As you may recall there are also many ways to load OSM data into Python. For example, you can readily use pyrosm
to get the POIs in Bristol:
from pyrosm import OSM, get_data
bristol = get_data("bristol")
osm = OSM(bristol)
bristol_pois = osm.get_pois()
However, some of you have encourtered issues of using this package. The main reason is that this package is sometimes out of date and its dependencies are not updated so that your system might have conflicts with it. The data extracted by this approach is sometimes also the very raw one without any preprocessing.
The second way, which is recommended in this tutorial, is to directly download the data from https://download.geofabrik.de/, and then load it to Python:
## Option 2 (Recommended): directly download it from the website and load it into python
bristol_pois_file = "../../LabData/Lab3/gis_osm_pois_a_free_1.shp"
bristol_pois = gpd.read_file(bristol_pois_file)
bristol_pois.head()
osm_id | code | fclass | name | geometry | |
---|---|---|---|---|---|
0 | 3894324 | 2204 | park | Portland Square | POLYGON ((-2.58600 51.46103, -2.58600 51.46110... |
1 | 4297151 | 2204 | park | Gainsborough Square | POLYGON ((-2.56426 51.49027, -2.56422 51.49031... |
2 | 4309554 | 2008 | town_hall | Bristol City Hall | POLYGON ((-2.60242 51.45242, -2.60241 51.45244... |
3 | 4313536 | 2204 | park | None | POLYGON ((-2.57615 51.49791, -2.57609 51.49803... |
4 | 4313541 | 2204 | park | Poets Park | POLYGON ((-2.57429 51.49952, -2.57419 51.49972... |
As can be seen, there is a fclass
column indicating the place type of the poi. We wil regard it as a token/term of a document, which will be explained in more detail later.
Again, let’s double check the crs of this data:
bristol_pois.crs
<Geographic 2D CRS: EPSG:4326>
Name: WGS 84
Axis Info [ellipsoidal]:
- Lat[north]: Geodetic latitude (degree)
- Lon[east]: Geodetic longitude (degree)
Area of Use:
- name: World.
- bounds: (-180.0, -90.0, 180.0, 90.0)
Datum: World Geodetic System 1984 ensemble
- Ellipsoid: WGS 84
- Prime Meridian: Greenwich
Let’s also check the number of place types we have in this dataset:
len(set(bristol_pois['fclass']))
104
Don’t forget we can also use .info()
to summary the basic info about such a dataset. For example, from the info below, we know that there are 5370 pois in this dataset.
bristol_pois.info() # you can also use list(bristol_pois.columns) to check the list of the columns
<class 'geopandas.geodataframe.GeoDataFrame'>
RangeIndex: 5370 entries, 0 to 5369
Data columns (total 5 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 osm_id 5370 non-null object
1 code 5370 non-null int64
2 fclass 5370 non-null object
3 name 4567 non-null object
4 geometry 5370 non-null geometry
dtypes: geometry(1), int64(1), object(3)
memory usage: 209.9+ KB
And, we can also simply plot these pois:
bristol_pois.plot()
<Axes: >
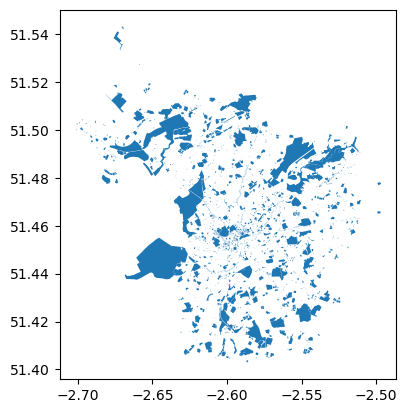
Part 3: Use WordCloud to Characterize Regions#
For natural languages like the place types, a popular way to visulize it is to use a technique called WordCloud. It draws a “cloud” of words, where the position and size of the word is determined by its frequency in the document. In Python, we can use the package wordcloud
to implement it. To do it, we need a document that contains words/tokens.
However, what is the document in our case study here?
There can be many ways and you are encouraged to be creative in answering this question. One way we will experiment here is to regard the whole City of Bristol as a document. Its words/tokens are those place types whose georeferenced POIs are within the City of Bristol.
Let’s next see how we implement it. We first need to convert the column fclass
in geodataframe
to a string (document). Then we can use the function WordCloud
provided by the package wordcloud
to build the cloud map. Note that the example showing below can be modified to other versions. You can check the wordcloud gallaries for some inspirations.
## use word cloud to visualize the frequency of poi types mentioned in Bristol
# first you need to install the package of wordcloud
from wordcloud import WordCloud, STOPWORDS
import matplotlib.pyplot as plt
bristol_pois_doc = " ".join(list(bristol_pois['fclass']))
wordcloud = WordCloud(width = 800, height = 800,
background_color ='white',
min_font_size = 10).generate(bristol_pois_doc)
# plot the WordCloud image
plt.figure(figsize = (8, 8), facecolor = None)
plt.imshow(wordcloud)
plt.axis("off")
plt.tight_layout(pad = 0)
plt.show()
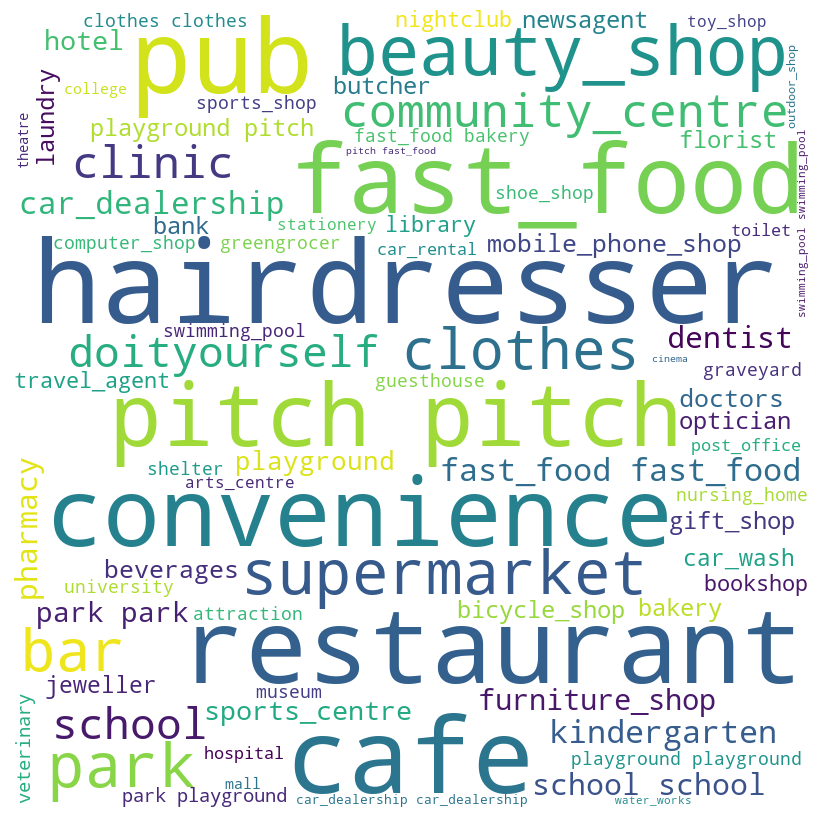
So, we here regarded the whole City as a document. Can you try to implement it by regarding each LSOA11 region as the document? What other approaches can you think of?
Part 4: Preprocessing the data for GIR#
After exploring some new techniques of visualizing regions using natural language processing techniques, next let’s come back to the implemention of techniques we have learnt in the lecture.
First, we would like to create a dataset where place types of POIs are assigned to each LSOA11 regions, as in later experiment, we will regard the LSOA11 regions as documents and place types will be the words/tokens.
To do it, we can use sjoin()
, which we have learnt before. Note the meaning of the parameters in the function, e.g., how
and op
.
bristol_lsoal_pois = gpd.sjoin(bristol_lsoal_gdf, bristol_pois , how="left", op='intersects')
bristol_lsoal_pois.head()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/IPython/core/interactiveshell.py:3493: FutureWarning: The `op` parameter is deprecated and will be removed in a future release. Please use the `predicate` parameter instead.
if await self.run_code(code, result, async_=asy):
OBJECTID | LSOA11 Code | LSOA11 Name | LSOA11 Local name | MSOA11 Code | Ward code | Area square mteres | Perimeter (m) | MI_PRINX | geo_shape | geo_point_2d | geom | index_right | osm_id | code | fclass | name | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | 1172.0 | 211278259 | 2204.0 | park | Briery Leaze Road Open Space |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | 4126.0 | 436088974 | 2205.0 | playground | None |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | 5114.0 | 750955626 | 2253.0 | swimming_pool | None |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | 5113.0 | 750955625 | 2253.0 | swimming_pool | None |
1 | 8 | E01014512 | Bristol 016E | Somerville Road | E02003027 | E05001975 | 178468.43750 | 3093.555908 | 152 | {"coordinates": [[[-2.5836634052406393, 51.476... | 51.47614221648566,-2.588736496528701 | POLYGON ((-2.58366 51.47624, -2.58344 51.47609... | 447.0 | 121690642 | 2013.0 | nursing_home | Sycamore Court |
There are many columns from OSM that we might not use. Here we can remove them by using codes like:
drop_cols = ['index_right', 'osm_id', 'code', 'name']
bristol_lsoal_pois_dropped = bristol_lsoal_pois.drop(drop_cols, axis=1)
bristol_lsoal_pois_dropped.head()
OBJECTID | LSOA11 Code | LSOA11 Name | LSOA11 Local name | MSOA11 Code | Ward code | Area square mteres | Perimeter (m) | MI_PRINX | geo_shape | geo_point_2d | geom | fclass | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | park |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | playground |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | swimming_pool |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | swimming_pool |
1 | 8 | E01014512 | Bristol 016E | Somerville Road | E02003027 | E05001975 | 178468.43750 | 3093.555908 | 152 | {"coordinates": [[[-2.5836634052406393, 51.476... | 51.47614221648566,-2.588736496528701 | POLYGON ((-2.58366 51.47624, -2.58344 51.47609... | nursing_home |
There are still many things we need to process before this data can be used for our later study. One thing is that the column fclass
might not be recorded as a str
(natural language based string) as we expect. To ensure it is, we can use the code:
bristol_lsoal_pois_dropped['fclass'] = bristol_lsoal_pois_dropped['fclass'].astype('str')
bristol_lsoal_pois_dropped.head()
OBJECTID | LSOA11 Code | LSOA11 Name | LSOA11 Local name | MSOA11 Code | Ward code | Area square mteres | Perimeter (m) | MI_PRINX | geo_shape | geo_point_2d | geom | fclass | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | park |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | playground |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | swimming_pool |
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.34375 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | swimming_pool |
1 | 8 | E01014512 | Bristol 016E | Somerville Road | E02003027 | E05001975 | 178468.43750 | 3093.555908 | 152 | {"coordinates": [[[-2.5836634052406393, 51.476... | 51.47614221648566,-2.588736496528701 | POLYGON ((-2.58366 51.47624, -2.58344 51.47609... | nursing_home |
More importantly, we need to group the place types (words/terms) to LSOA11 regions (documents). To do so, we can use the groupby()
function provided in pandas
. Here is how it looks like:
bristol_lsoal_pois_dropped.groupby('LSOA11 Code')['fclass'].apply(list)
LSOA11 Code
E01014485 [sports_centre, sports_centre, recycling_metal...
E01014486 [sports_centre, pitch, pitch, pitch, cafe, pit...
E01014487 [hairdresser, clothes, sports_shop, furniture_...
E01014488 [park, ruins, pitch, school, school, school, p...
E01014489 [community_centre, fast_food, convenience, caf...
...
E01033364 [sports_shop, convenience, fast_food, convenie...
E01033366 [convenience, playground, library, cafe, park,...
E01033367 [mall, cafe, park, pub, playground, restaurant...
E01033369 [car_dealership, recycling_glass, stationery, ...
E01033370 [convenience, restaurant, kindergarten, school...
Name: fclass, Length: 263, dtype: object
Once we have the grouped dataframe, we want to join it back to the bristol_lsoal_gdf
dataframe. Here we can use a general join()
:
bristol_lsoal_poi_full = bristol_lsoal_gdf.join(bristol_lsoal_pois_dropped.groupby('LSOA11 Code')['fclass'].apply(list), on='LSOA11 Code')
bristol_lsoal_poi_full.head()
OBJECTID | LSOA11 Code | LSOA11 Name | LSOA11 Local name | MSOA11 Code | Ward code | Area square mteres | Perimeter (m) | MI_PRINX | geo_shape | geo_point_2d | geom | fclass | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 7 | E01014612 | Bristol 052B | Fortfield East | E02003063 | E05001989 | 250084.343750 | 3010.960449 | 2 | {"coordinates": [[[-2.5705649134167503, 51.408... | 51.409649589944884,-2.566230812955203 | POLYGON ((-2.57056 51.40811, -2.57048 51.40829... | [park, playground, swimming_pool, swimming_pool] |
1 | 8 | E01014512 | Bristol 016E | Somerville Road | E02003027 | E05001975 | 178468.437500 | 3093.555908 | 152 | {"coordinates": [[[-2.5836634052406393, 51.476... | 51.47614221648566,-2.588736496528701 | POLYGON ((-2.58366 51.47624, -2.58344 51.47609... | [nursing_home, hairdresser, computer_shop, fas... |
2 | 18 | E01014618 | Bristol 011C | Henleaze North | E02003022 | E05001990 | 324102.562500 | 4205.579590 | 921 | {"coordinates": [[[-2.610432616527603, 51.4856... | 51.489587737185744,-2.606558179420023 | POLYGON ((-2.61043 51.48565, -2.61012 51.48600... | [kindergarten, convenience, hairdresser, fast_... |
3 | 36 | E01014576 | Bristol 019D | Rose Green | E02003030 | E05001984 | 469484.093750 | 3586.286133 | 976 | {"coordinates": [[[-2.5511455096576006, 51.463... | 51.46845752679203,-2.5464321087549955 | POLYGON ((-2.55115 51.46378, -2.55102 51.46396... | [sports_shop, beauty_shop, convenience, fast_f... |
4 | 24 | E01033370 | Bristol 054E | Redcliffe South | E02006887 | E05001995 | 126227.867188 | 2103.395020 | 19 | {"coordinates": [[[-2.5911512584061063, 51.445... | 51.44681337159509,-2.5874479497333067 | POLYGON ((-2.59115 51.44567, -2.59098 51.44604... | [convenience, restaurant, kindergarten, school... |
We can also save the data as a pickle
for future use by applying the following code. pickle
is a module in python that convert python object into a byte stream (called “Pickling”), and vice verse (called “unpickling”).
bristol_lsoal_poi_full.to_pickle("./bristol_lsoal_poi_full.pkl")
Part 5: Compute TF-IDF#
After the preprocessing, now let’s apply some techniques in IR on these unstructured geo-text (i.e., poi’s place types for regions). There are many things we can do here. The first thing we want is to find the most representative place types for the region, in analogy to finding the representative words in a document. So, we can use the TF-IDF we discussed in the lecture to do so.
Before computing TF-IDF, we need to have a vocabulary. Here we simply call it a wordSet
, which includes all the unique terms used in the document:
wordSet = set()
for index, row in bristol_lsoal_poi_full.iterrows():
wordSet = wordSet.union(set(row['fclass']))
wordSet
{'archaeological',
'arts_centre',
'artwork',
'attraction',
'bakery',
'bank',
'bar',
'beauty_shop',
'beverages',
'bicycle_shop',
'bookshop',
'butcher',
'cafe',
'camp_site',
'car_dealership',
'car_rental',
'car_wash',
'caravan_site',
'castle',
'chemist',
'cinema',
'clinic',
'clothes',
'college',
'comms_tower',
'community_centre',
'computer_shop',
'convenience',
'courthouse',
'dentist',
'department_store',
'doctors',
'doityourself',
'fast_food',
'fire_station',
'florist',
'food_court',
'fountain',
'furniture_shop',
'garden_centre',
'gift_shop',
'golf_course',
'graveyard',
'greengrocer',
'guesthouse',
'hairdresser',
'hospital',
'hostel',
'hotel',
'jeweller',
'kindergarten',
'laundry',
'library',
'mall',
'market_place',
'memorial',
'mobile_phone_shop',
'monument',
'museum',
'nan',
'newsagent',
'nightclub',
'nursing_home',
'observation_tower',
'optician',
'outdoor_shop',
'park',
'pharmacy',
'picnic_site',
'pitch',
'playground',
'police',
'post_office',
'prison',
'pub',
'public_building',
'recycling',
'recycling_glass',
'recycling_metal',
'restaurant',
'ruins',
'school',
'shelter',
'shoe_shop',
'sports_centre',
'sports_shop',
'stadium',
'stationery',
'supermarket',
'swimming_pool',
'theatre',
'theme_park',
'toilet',
'tourist_info',
'tower',
'town_hall',
'toy_shop',
'track',
'travel_agent',
'university',
'veterinary',
'video_shop',
'wastewater_plant',
'water_tower',
'water_works'}
Once we have the vocabulary, next we will see how frequently each word/token in the vacabulary occurred in each of my document:
docDic = {}
for index, row in bristol_lsoal_poi_full.iterrows():
doc = row['fclass']
wordDict = dict.fromkeys(wordSet, 0)
for word in doc:
wordDict[word]+=1
docDic[row['LSOA11 Code']] = wordDict
docDic
{'E01014612': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 2,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014512': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 3,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 3,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 3,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 1,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014618': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014576': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 1,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033370': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 2,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014518': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 3,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014731': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 4,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 1,
'university': 0,
'supermarket': 0},
'E01033345': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 3,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 5,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 23,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 13,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 2,
'cinema': 1,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 14,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 9,
'laundry': 0,
'theatre': 2,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 2,
'college': 0,
'police': 0,
'bar': 7,
'hospital': 0,
'florist': 1,
'tourist_info': 1,
'town_hall': 1,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 1,
'hostel': 0,
'swimming_pool': 0,
'fountain': 5,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 2,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 4,
'optician': 0,
'hairdresser': 9,
'gift_shop': 1,
'university': 0,
'supermarket': 2},
'E01014591': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014611': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014550': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 1,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 1,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 5,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014568': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 5,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 1,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 9,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 7,
'graveyard': 0,
'recycling': 0,
'pharmacy': 2,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 2,
'archaeological': 0,
'school': 0,
'clothes': 3,
'cinema': 0,
'butcher': 3,
'hotel': 0,
'garden_centre': 0,
'cafe': 3,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 2,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 4,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 5,
'gift_shop': 1,
'university': 0,
'supermarket': 4},
'E01033359': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 3,
'convenience': 2,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 1,
'bank': 2,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 1,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 9,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 1,
'archaeological': 0,
'school': 0,
'clothes': 2,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 8,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 6,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 7,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 1,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 1,
'jeweller': 1,
'shoe_shop': 1,
'artwork': 0,
'nightclub': 1,
'optician': 2,
'hairdresser': 7,
'gift_shop': 1,
'university': 0,
'supermarket': 1},
'E01014549': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 1,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014554': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 1,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 2,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 2,
'supermarket': 0},
'E01014585': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014600': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014648': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 2,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 1,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 1,
'university': 0,
'supermarket': 0},
'E01014566': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 4,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 5,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 2,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014654': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 6,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 7,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 1,
'archaeological': 0,
'school': 3,
'clothes': 2,
'cinema': 0,
'butcher': 4,
'hotel': 0,
'garden_centre': 0,
'cafe': 7,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 2,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 2,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 2,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014649': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014636': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014569': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 4,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014532': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014560': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014587': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 4,
'supermarket': 0},
'E01014592': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 1,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014706': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 5,
'graveyard': 0,
'recycling': 0,
'pharmacy': 2,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014642': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033366': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014553': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 2,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 3,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 1,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 7,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 1,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 1,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 9,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 2,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 1,
'bar': 3,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 9,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 2,
'hairdresser': 2,
'gift_shop': 1,
'university': 0,
'supermarket': 2},
'E01014632': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014644': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 2,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 6,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014733': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014588': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 4,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 1,
'archaeological': 0,
'school': 2,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 1,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 1,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 2,
'gift_shop': 0,
'university': 1,
'supermarket': 4},
'E01014487': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 1,
'university': 0,
'supermarket': 0},
'E01014633': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 1,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033362': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 1,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 5,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 3,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 3,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 1,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014722': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014687': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014577': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 2,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014725': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014575': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 1,
'toilet': 0,
'library': 0,
'pitch': 7,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014692': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033347': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 3,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 1,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 3,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 1,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 1,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014645': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 2,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 2,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014498': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 2,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 1,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 6,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 2,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 1,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 3,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 1,
'college': 0,
'police': 1,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014617': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014539': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 4,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014670': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014640': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 2,
'sports_centre': 1,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014679': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014653': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 6,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 3,
'travel_agent': 1,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 2,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 1,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014676': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 1,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014601': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014651': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 1,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01032518': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014521': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014664': {'food_court': 0,
'camp_site': 1,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 5,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 1,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014489': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 6,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 4,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 5,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 6,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 5,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014655': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 10,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 9,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 2,
'archaeological': 0,
'school': 0,
'clothes': 1,
'cinema': 0,
'butcher': 3,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 8,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014589': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014683': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 1,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014646': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 3,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 1,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 1,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 2,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033364': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 5,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 4,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 2,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014528': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 2,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014734': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014665': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 1,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 2,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 1,
'pub': 0,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 1,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 3},
'E01014526': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 21,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 3,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 2,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 1,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014499': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 3,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014712': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 2,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014563': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 7,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 1,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014675': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 3,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014511': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014729': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 6,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014641': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 2,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014637': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014525': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01032515': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 1,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014527': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 4,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014520': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014677': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014630': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 2,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014615': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014536': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 2,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 4,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014661': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014602': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014516': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 3,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014671': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 5,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 7,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 6,
'graveyard': 0,
'recycling': 0,
'pharmacy': 2,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 4,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 3,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 4,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 3,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 6,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014562': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014571': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014621': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 3,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 1,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014522': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014622': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 1,
'fire_station': 0,
'market_place': 0,
'fast_food': 7,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 1,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 2,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 6,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014717': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 5,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 2,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014718': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014492': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014501': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 1,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014559': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 3,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 2,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 1,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014581': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 6,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014534': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 2,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014684': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 2,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 1,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 4,
'graveyard': 1,
'recycling': 1,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014507': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014673': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 2,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014699': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014515': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014531': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 6,
'convenience': 4,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 1,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 6,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 1,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 2,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 5,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 7,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014608': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 4,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01033355': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 1,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 4,
'recycling_glass': 0,
'tower': 1,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 1,
'market_place': 0,
'fast_food': 7,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 5,
'garden_centre': 0,
'cafe': 5,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 1,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 7,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 1,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014586': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 1,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 2,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014597': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014582': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 1,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014567': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 6,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 6,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 4,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 6,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014669': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 6,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014510': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 1,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014570': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 6,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033352': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 2,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 1,
'fast_food': 2,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 1,
'doityourself': 3,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 4,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 5,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 1,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014613': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014701': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 3,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014486': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 9,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 5,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 8,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 7,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 6,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 1,
'doityourself': 0,
'playground': 4,
'travel_agent': 2,
'archaeological': 0,
'school': 3,
'clothes': 1,
'cinema': 0,
'butcher': 2,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 1,
'community_centre': 2,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 2,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 6,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014548': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 3,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014506': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 3,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 6,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014508': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 2,
'stadium': 1,
'toy_shop': 1,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 5,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 1,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 4,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 7,
'newsagent': 2,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 5,
'laundry': 2,
'theatre': 1,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 2,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 1,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 4,
'gift_shop': 3,
'university': 0,
'supermarket': 0},
'E01014579': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 1,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 1,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014678': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 4,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033353': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 1,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 1,
'doityourself': 2,
'playground': 0,
'travel_agent': 1,
'archaeological': 0,
'school': 1,
'clothes': 3,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 6,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 3,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 10,
'laundry': 0,
'theatre': 1,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 2,
'dentist': 2,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 4,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014624': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01032514': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014509': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 1,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014726': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014690': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 1,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014702': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014626': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 11,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 1,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014572': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 2,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 4,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 4,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 7,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 2,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 11,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014735': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 4,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 1,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014714': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 1,
'tower': 0,
'toilet': 1,
'library': 0,
'pitch': 6,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 2,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 1,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 1,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 1,
'supermarket': 0},
'E01014715': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 1,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 1,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 3,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 2,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014491': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014555': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014610': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014689': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 6,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 5,
'graveyard': 0,
'recycling': 0,
'pharmacy': 2,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 3,
'newsagent': 0,
'community_centre': 3,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 2,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014631': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014666': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 6,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014502': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 4,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 2,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014523': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014596': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014638': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 6,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 4,
'graveyard': 1,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014682': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014659': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014705': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014493': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 5,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 1,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 1,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 4,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 6,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01033367': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 7,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 1,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 2,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 3,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 1,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 1,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 1,
'university': 0,
'supermarket': 0},
'E01014623': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 4,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014497': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014700': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 1,
'toy_shop': 0,
'bookshop': 1,
'park': 0,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 6,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 4,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 2,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014668': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 2,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 2,
'bookshop': 1,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 4,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 5,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 2,
'castle': 0,
'bakery': 2,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 4,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 4,
'university': 0,
'supermarket': 3},
'E01033356': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014584': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014561': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 6,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 5,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 2,
'sports_centre': 0,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014694': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014556': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 1,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014732': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 1,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 4,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 1,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014627': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 2,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014708': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 14,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014709': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 2,
'library': 0,
'pitch': 20,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 1,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 1,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 6,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014544': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 1,
'theatre': 1,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 3,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 2,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 2,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033361': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 3,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 1,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 2,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 5,
'graveyard': 1,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 2,
'cinema': 0,
'butcher': 1,
'hotel': 1,
'garden_centre': 0,
'cafe': 5,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 4,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 2,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 2,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 1,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 9,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014691': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 9,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 4,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 2,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014658': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 6,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 7,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 1,
'butcher': 0,
'hotel': 0,
'garden_centre': 1,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 1,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 1,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 3},
'E01014524': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014546': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 4,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 3,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 15,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 2,
'recycling': 0,
'pharmacy': 2,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 1,
'archaeological': 0,
'school': 0,
'clothes': 13,
'cinema': 0,
'butcher': 1,
'hotel': 2,
'garden_centre': 0,
'cafe': 9,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 8,
'laundry': 2,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 2,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 1,
'florist': 2,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 8,
'shoe_shop': 1,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 7,
'gift_shop': 2,
'university': 0,
'supermarket': 1},
'E01014629': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014519': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014672': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 7,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 4,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 4,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014500': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 2,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014517': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014686': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014639': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014616': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 1,
'doityourself': 1,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 1,
'cafe': 3,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 2,
'hairdresser': 9,
'gift_shop': 1,
'university': 0,
'supermarket': 1},
'E01014713': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 1,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 2,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014593': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014681': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 3,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 3,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 5,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014547': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033358': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 1,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 2,
'garden_centre': 0,
'cafe': 3,
'newsagent': 1,
'community_centre': 2,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 1,
'pub': 4,
'laundry': 0,
'theatre': 1,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 1,
'bar': 3,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 3,
'public_building': 0,
'arts_centre': 1,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014696': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 1,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014540': {'food_court': 1,
'camp_site': 0,
'theme_park': 0,
'beverages': 2,
'sports_shop': 0,
'memorial': 1,
'car_rental': 0,
'nan': 0,
'beauty_shop': 7,
'convenience': 7,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 5,
'stadium': 0,
'toy_shop': 2,
'bookshop': 6,
'park': 4,
'outdoor_shop': 2,
'bank': 8,
'restaurant': 52,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 2,
'fire_station': 0,
'market_place': 1,
'fast_food': 27,
'graveyard': 0,
'recycling': 0,
'pharmacy': 2,
'mall': 8,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 6,
'archaeological': 0,
'school': 1,
'clothes': 25,
'cinema': 2,
'butcher': 1,
'hotel': 10,
'garden_centre': 0,
'cafe': 37,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 2,
'kindergarten': 1,
'courthouse': 1,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 5,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 32,
'laundry': 0,
'theatre': 1,
'track': 0,
'monument': 1,
'mobile_phone_shop': 9,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 3,
'museum': 1,
'college': 2,
'police': 0,
'bar': 21,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 3,
'water_works': 0,
'computer_shop': 0,
'ruins': 3,
'observation_tower': 2,
'chemist': 0,
'doctors': 3,
'attraction': 5,
'hostel': 3,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 2,
'jeweller': 5,
'shoe_shop': 6,
'artwork': 0,
'nightclub': 5,
'optician': 2,
'hairdresser': 18,
'gift_shop': 7,
'university': 0,
'supermarket': 1},
'E01014634': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 3,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01033350': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 2,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 3,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 3,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 1,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 4,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 1,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 4,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 2,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014723': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014530': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014635': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014496': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 1,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 2,
'market_place': 0,
'fast_food': 6,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 2,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 1,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014573': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 2,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014598': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 2,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 2,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014551': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 1,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 1,
'butcher': 1,
'hotel': 1,
'garden_centre': 1,
'cafe': 3,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 1,
'theatre': 1,
'track': 0,
'monument': 0,
'mobile_phone_shop': 2,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 6,
'water_works': 1,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 1,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 0,
'gift_shop': 1,
'university': 1,
'supermarket': 2},
'E01014603': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014680': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 1,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014542': {'food_court': 1,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 1,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 4,
'convenience': 2,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 3,
'stadium': 0,
'toy_shop': 0,
'bookshop': 3,
'park': 4,
'outdoor_shop': 2,
'bank': 4,
'restaurant': 20,
'recycling_glass': 0,
'tower': 2,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 13,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 4,
'doityourself': 1,
'playground': 0,
'travel_agent': 1,
'archaeological': 0,
'school': 3,
'clothes': 15,
'cinema': 0,
'butcher': 0,
'hotel': 3,
'garden_centre': 0,
'cafe': 25,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 1,
'department_store': 1,
'pub': 12,
'laundry': 0,
'theatre': 2,
'track': 0,
'monument': 0,
'mobile_phone_shop': 2,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 0,
'dentist': 1,
'museum': 3,
'college': 1,
'police': 1,
'bar': 16,
'hospital': 5,
'florist': 2,
'tourist_info': 0,
'town_hall': 0,
'clinic': 3,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 3,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 4,
'jeweller': 1,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 7,
'optician': 3,
'hairdresser': 15,
'gift_shop': 2,
'university': 4,
'supermarket': 2},
'E01014594': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014716': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014695': {'food_court': 0,
'camp_site': 0,
'theme_park': 1,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 1,
'toy_shop': 0,
'bookshop': 0,
'park': 5,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 16,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 1,
'doityourself': 1,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 1,
'cafe': 3,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 2,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 4,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 1,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 2,
'supermarket': 0},
'E01032517': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014703': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014537': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 5,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014533': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 1,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 3,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014647': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014711': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 4,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014565': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 5,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01032516': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 3,
'travel_agent': 0,
'archaeological': 0,
'school': 4,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 1,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 1,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014574': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 1,
'archaeological': 0,
'school': 1,
'clothes': 1,
'cinema': 0,
'butcher': 3,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 1,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014529': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014514': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 3,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 10,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 1,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 7,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 1,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 2,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 11,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 2,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 1,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 1,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 1,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 12,
'gift_shop': 0,
'university': 0,
'supermarket': 3},
'E01014662': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014607': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 1,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 2,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 1,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014620': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014505': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014495': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 2,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014663': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 1,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 1,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014535': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 6,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014667': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 1,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014513': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 1,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014595': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014580': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014552': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 2,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 1,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 1,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 2,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 3,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01032519': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014688': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 3,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 7,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014719': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 4,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 1,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 5,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 4,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 4,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 3,
'water_works': 0,
'computer_shop': 2,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 2,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 1,
'shoe_shop': 1,
'artwork': 0,
'nightclub': 0,
'optician': 2,
'hairdresser': 7,
'gift_shop': 1,
'university': 0,
'supermarket': 3},
'E01014557': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 6,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 1,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014710': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 1,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014693': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 3,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014578': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 4,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014707': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014650': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014730': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 5,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014698': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 1,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 5,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 1,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 2,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033369': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 1,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 1,
'doityourself': 0,
'playground': 4,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014599': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014674': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014619': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 2,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 3,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 1,
'archaeological': 0,
'school': 1,
'clothes': 1,
'cinema': 1,
'butcher': 1,
'hotel': 1,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 3,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 1,
'shelter': 1,
'dentist': 3,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 3,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014538': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 1,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 4,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 2,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 5,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 3,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 1,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 6,
'supermarket': 1},
'E01014736': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033342': {'food_court': 0,
'camp_site': 0,
'theme_park': 1,
'beverages': 0,
'sports_shop': 1,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 8,
'convenience': 4,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 1,
'bookshop': 2,
'park': 3,
'outdoor_shop': 3,
'bank': 4,
'restaurant': 4,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 1,
'fire_station': 0,
'market_place': 0,
'fast_food': 16,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 2,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 1,
'archaeological': 0,
'school': 0,
'clothes': 23,
'cinema': 1,
'butcher': 0,
'hotel': 1,
'garden_centre': 0,
'cafe': 11,
'newsagent': 1,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 1,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 2,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 2,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 11,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 1,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 2,
'public_building': 0,
'arts_centre': 0,
'jeweller': 1,
'shoe_shop': 4,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 8,
'gift_shop': 2,
'university': 0,
'supermarket': 0},
'E01033344': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 1,
'park': 3,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 6,
'recycling_glass': 0,
'tower': 0,
'toilet': 1,
'library': 1,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 6,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 1,
'archaeological': 0,
'school': 6,
'clothes': 3,
'cinema': 0,
'butcher': 0,
'hotel': 3,
'garden_centre': 0,
'cafe': 7,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 6,
'laundry': 0,
'theatre': 1,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 2,
'college': 1,
'police': 0,
'bar': 2,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 2,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 1,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 1,
'optician': 0,
'hairdresser': 4,
'gift_shop': 0,
'university': 1,
'supermarket': 1},
'E01014494': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 1,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 1,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01033348': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 6,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 1,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 6,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 4,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 1,
'archaeological': 0,
'school': 0,
'clothes': 1,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 1,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014720': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 2,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014564': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 1,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014605': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 1,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 6,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 1,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 4,
'gift_shop': 0,
'university': 0,
'supermarket': 3},
'E01014504': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014488': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 3,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 1,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014583': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 1,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014643': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 0,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 2,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 5,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014727': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 1,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 1,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 2},
'E01014609': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 0,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014545': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 1,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 1,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 4,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 1,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 3,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 5,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 2,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 1,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 3,
'shoe_shop': 1,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 2,
'university': 0,
'supermarket': 0},
'E01014721': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 0,
'car_wash': 1,
'golf_course': 1,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 2,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 3,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 1,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014590': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 1,
'convenience': 3,
'car_wash': 1,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 6,
'outdoor_shop': 0,
'bank': 2,
'restaurant': 4,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 8,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 1,
'doityourself': 0,
'playground': 1,
'travel_agent': 1,
'archaeological': 0,
'school': 1,
'clothes': 0,
'cinema': 0,
'butcher': 1,
'hotel': 0,
'garden_centre': 0,
'cafe': 2,
'newsagent': 1,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 3,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 1,
'museum': 0,
'college': 0,
'police': 0,
'bar': 1,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 1,
'doctors': 2,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 2,
'hairdresser': 5,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014614': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 2,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 1,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 1,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 4,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 1,
'tourist_info': 0,
'town_hall': 0,
'clinic': 1,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 2,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014485': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 3,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 1,
'doityourself': 0,
'playground': 2,
'travel_agent': 0,
'archaeological': 0,
'school': 2,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 1,
'community_centre': 2,
'sports_centre': 2,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 1,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 2,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 1,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 1},
'E01014558': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 3,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 5,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 1,
'park': 0,
'outdoor_shop': 0,
'bank': 1,
'restaurant': 14,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 7,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 2,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 1,
'clothes': 3,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 8,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 1,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 1,
'castle': 0,
'bakery': 1,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 5,
'laundry': 1,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 1,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 5,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 2,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 1,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 1,
'hairdresser': 9,
'gift_shop': 1,
'university': 0,
'supermarket': 2},
'E01014660': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 3,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 2,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 2,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 2,
'graveyard': 0,
'recycling': 0,
'pharmacy': 1,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 4,
'travel_agent': 0,
'archaeological': 2,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 1,
'newsagent': 0,
'community_centre': 3,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 6,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 1,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 1,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014704': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 1,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 0,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 0,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 0,
'sports_centre': 0,
'kindergarten': 0,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 1,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0},
'E01014685': {'food_court': 0,
'camp_site': 0,
'theme_park': 0,
'beverages': 0,
'sports_shop': 0,
'memorial': 0,
'car_rental': 0,
'nan': 0,
'beauty_shop': 0,
'convenience': 1,
'car_wash': 0,
'golf_course': 0,
'bicycle_shop': 0,
'stadium': 0,
'toy_shop': 0,
'bookshop': 0,
'park': 0,
'outdoor_shop': 0,
'bank': 0,
'restaurant': 0,
'recycling_glass': 0,
'tower': 0,
'toilet': 0,
'library': 0,
'pitch': 3,
'prison': 0,
'video_shop': 0,
'fire_station': 0,
'market_place': 0,
'fast_food': 1,
'graveyard': 0,
'recycling': 0,
'pharmacy': 0,
'mall': 0,
'post_office': 0,
'water_tower': 0,
'veterinary': 0,
'stationery': 0,
'doityourself': 0,
'playground': 0,
'travel_agent': 0,
'archaeological': 0,
'school': 0,
'clothes': 0,
'cinema': 0,
'butcher': 0,
'hotel': 0,
'garden_centre': 0,
'cafe': 0,
'newsagent': 0,
'community_centre': 1,
'sports_centre': 0,
'kindergarten': 1,
'courthouse': 0,
'comms_tower': 0,
'greengrocer': 0,
'castle': 0,
'bakery': 0,
'recycling_metal': 0,
'caravan_site': 0,
'wastewater_plant': 0,
'department_store': 0,
'pub': 0,
'laundry': 0,
'theatre': 0,
'track': 0,
'monument': 0,
'mobile_phone_shop': 0,
'nursing_home': 0,
'car_dealership': 0,
'guesthouse': 0,
'shelter': 0,
'dentist': 0,
'museum': 0,
'college': 0,
'police': 0,
'bar': 0,
'hospital': 0,
'florist': 0,
'tourist_info': 0,
'town_hall': 0,
'clinic': 0,
'water_works': 0,
'computer_shop': 0,
'ruins': 0,
'observation_tower': 0,
'chemist': 0,
'doctors': 0,
'attraction': 0,
'hostel': 0,
'swimming_pool': 0,
'fountain': 0,
'picnic_site': 0,
'furniture_shop': 0,
'public_building': 0,
'arts_centre': 0,
'jeweller': 0,
'shoe_shop': 0,
'artwork': 0,
'nightclub': 0,
'optician': 0,
'hairdresser': 0,
'gift_shop': 0,
'university': 0,
'supermarket': 0}}
docDic
here is a dictionary of dictionary. The inner dictionary is the frequency of each word occurred in the document. The outer dictionary is about different documents. We next can convert such a dictionary into a dataframe
:
doc2wordMatrix = pd.DataFrame.from_dict(docDic, orient='index').rename_axis('LSOA11 Code').reset_index()
doc2wordMatrix.head()
LSOA11 Code | food_court | camp_site | theme_park | beverages | sports_shop | memorial | car_rental | nan | beauty_shop | ... | arts_centre | jeweller | shoe_shop | artwork | nightclub | optician | hairdresser | gift_shop | university | supermarket | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | E01014612 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
1 | E01014512 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 0 | 0 | 0 |
2 | E01014618 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 0 | 0 | 0 |
3 | E01014576 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 1 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
4 | E01033370 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | ... | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
5 rows × 106 columns
Note here such a data sometimes is referred as a document matrix, where each row is a document, and each column is a word/token from the vocabulary. The value in the matrix represents the frequency of the word/token in the correspoding document. For example, we can see that place types car_dealership
and college
both occurred once in the document with LSOA11 code “E01014512” (remember that it is actually a region in our example).
Now, we can start computing the term frequency-inverse document frequency (TF-IDF), which will be used later for us to determine the relevance ranking of documents in relation to a query. The functions are written below. We first compute TF, and then IDF. Multiplying both, we will have TF-IDF. Please read it first and try to think if you can understand it:
## Compute term frequency (TF)
def computeTF(wordDict):
tfDict = {}
bowCount = 0
for word, count in wordDict.items():
if count >0:
bowCount +=1
for word, count in wordDict.items():
tfDict[word] = count/float(bowCount)
return tfDict
We can use the computeTF()
function on one example document (region with code ‘E01014612’). From the result, you can see the frequency of “swimming_pool” in this region is 0.666666666, frequency of “park” is 0.3333333, and the frequency of seeing “playground” is also 0.33333333.
computeTF(docDic['E01014612'])
{'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.3333333333333333,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.3333333333333333,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.6666666666666666,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0}
Next, we have the function computeIDF()
to compute inverse document frequency. Note the use of math.log10()
in the last step is to avoid the situation where the number of documents N is too large making N/float(count)
become too large as well. This logarithm is to scale the inversed document frequency to a relatively small value (e.g., a dampen effect).
## Compute inverse document frequency (IDF)
def computeIDF(docDic):
import math
idfDict = {}
N = len(docDic)
idfDict = dict.fromkeys(wordSet, 0)
for doc, wordDic in docDic.items():
for word, count in wordDic.items():
if count > 0:
idfDict[word] += 1
for word, count in idfDict.items(): ## this count is the number of document containing the word
idfDict[word] = math.log10(N / float(count))
return idfDict
With this function, we can then input all the documents (represented using the docDoc
variable) to it so as to obtain the inverse document frequency (idfs), which is also represented as a dictionary. The keys of the dictionary are the words/tokens from the vocabulary. The values are the computed IDF.
idfs = computeIDF(docDic)
idfs
{'food_court': 2.1189257528257768,
'camp_site': 2.419955748489758,
'theme_park': 2.1189257528257768,
'beverages': 0.8517540244227628,
'sports_shop': 1.1189257528257768,
'memorial': 2.1189257528257768,
'car_rental': 1.306012396182921,
'nan': 2.419955748489758,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 1.3407745024421331,
'bicycle_shop': 1.058227912472165,
'stadium': 1.6418044981061142,
'toy_shop': 1.378563063331533,
'bookshop': 1.2738277128115199,
'park': 0.17198248212795123,
'outdoor_shop': 1.574857708475501,
'bank': 1.141202147536929,
'restaurant': 0.4905368227754652,
'recycling_glass': 1.9428344937700954,
'tower': 1.720985744153739,
'toilet': 1.2738277128115199,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 1.9428344937700954,
'video_shop': 1.9428344937700954,
'fire_station': 1.8178957571617955,
'market_place': 1.8178957571617955,
'fast_food': 0.26461971102469606,
'graveyard': 0.9885919843307706,
'recycling': 2.419955748489758,
'pharmacy': 0.7039524048549587,
'mall': 1.4199557484897578,
'post_office': 1.215835765833833,
'water_tower': 2.1189257528257768,
'veterinary': 1.058227912472165,
'stationery': 1.378563063331533,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 1.141202147536929,
'archaeological': 1.9428344937700954,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 1.574857708475501,
'butcher': 1.0049824005189398,
'hotel': 1.0220157398177203,
'garden_centre': 1.720985744153739,
'cafe': 0.428729672797263,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.679593058995514,
'courthouse': 1.9428344937700954,
'comms_tower': 2.1189257528257768,
'greengrocer': 1.1189257528257768,
'castle': 2.1189257528257768,
'bakery': 0.7478578905540404,
'recycling_metal': 2.419955748489758,
'caravan_site': 2.419955748489758,
'wastewater_plant': 2.1189257528257768,
'department_store': 1.8178957571617955,
'pub': 0.2961041075226721,
'laundry': 0.9575577505908017,
'theatre': 1.378563063331533,
'track': 1.306012396182921,
'monument': 2.1189257528257768,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.6491037368476137,
'guesthouse': 1.215835765833833,
'shelter': 1.189506827111484,
'dentist': 0.7297596684612442,
'museum': 1.3407745024421331,
'college': 1.215835765833833,
'police': 1.574857708475501,
'bar': 0.8517540244227628,
'hospital': 1.189506827111484,
'florist': 0.9575577505908017,
'tourist_info': 2.1189257528257768,
'town_hall': 2.1189257528257768,
'clinic': 0.6640808928172665,
'water_works': 1.378563063331533,
'computer_shop': 1.189506827111484,
'ruins': 1.5168657614978143,
'observation_tower': 2.419955748489758,
'chemist': 2.1189257528257768,
'doctors': 0.8288911414632587,
'attraction': 1.3407745024421331,
'hostel': 1.9428344937700954,
'swimming_pool': 1.058227912472165,
'fountain': 2.419955748489758,
'picnic_site': 2.419955748489758,
'furniture_shop': 0.8636532477224705,
'public_building': 2.1189257528257768,
'arts_centre': 1.4199557484897578,
'jeweller': 1.2738277128115199,
'shoe_shop': 1.465713239050433,
'artwork': 2.419955748489758,
'nightclub': 1.465713239050433,
'optician': 0.9885919843307706,
'hairdresser': 0.2864168401195404,
'gift_shop': 1.141202147536929,
'university': 1.465713239050433,
'supermarket': 0.5168657614978143}
By multiplying tf
and idf
, we can finally have the TF-IDF using the function:
def computeTFIDF(tf, idfs):
tfidf = {}
for word, freq in tf.items():
tfidf[word] = freq*idfs[word]
return tfidf
Here is an example on how we use the function:
tfidfDic = {}
for doc, wordDic in docDic.items():
doc_tfidf = computeTFIDF(wordDic, idfs)
tfidfDic[doc] = doc_tfidf
tfidfDic
{'E01014612': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 2.11645582494433,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014512': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.7757632387643489,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 2.359461878730514,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 2.3295092160107114,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014618': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014576': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 1.378563063331533,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033370': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 2.0440314796354406,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014518': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 2.359461878730514,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014731': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.1844164300906883,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 0.0},
'E01033345': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 2.5552620732682882,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.8599124106397561,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 11.2823469238357,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 3.440056243321049,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 1.6803443037458954,
'cinema': 1.574857708475501,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 6.002215419161682,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 2.664936967704049,
'laundry': 0.0,
'theatre': 2.757126126663066,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 2.6815490048842663,
'college': 0.0,
'police': 0.0,
'bar': 5.9622781709593395,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 2.1189257528257768,
'town_hall': 2.1189257528257768,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 1.3407745024421331,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 12.099778742448791,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 2.8399114969795156,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 5.862852956201732,
'optician': 0.0,
'hairdresser': 2.5777515610758632,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014591': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014611': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014550': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 1.574857708475501,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.4805205376133603,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014568': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 1.292938731273915,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 1.378563063331533,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 4.414831404979187,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.8523379771728723,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 1.4079048097099174,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 2.282404295073858,
'archaeological': 0.0,
'school': 0.0,
'clothes': 2.520516455618843,
'cinema': 0.0,
'butcher': 3.0149472015568195,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 1.4957157811080808,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.1844164300906883,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.432084200597702,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 2.067463045991257},
'E01033359': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 1.7433199732575078,
'convenience': 0.517175492509566,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 1.574857708475501,
'bank': 2.282404295073858,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 2.3815773992222646,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.0,
'clothes': 1.6803443037458954,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 3.429837382378104,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.7766246451360326,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 7.988415032758502,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 2.1189257528257768,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 1.4199557484897578,
'jeweller': 1.2738277128115199,
'shoe_shop': 1.465713239050433,
'artwork': 0.0,
'nightclub': 1.465713239050433,
'optician': 1.9771839686615411,
'hairdresser': 2.004917880836783,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014549': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 1.720985744153739,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014554': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 1.2738277128115199,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 2.0440314796354406,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 2.931426478100866,
'supermarket': 0.0},
'E01014585': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014600': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014648': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 2.282404295073858,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 1.4199557484897578,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 0.0},
'E01014566': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 3.4070160976910513,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.3230985551234804,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 1.4957157811080808,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014654': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 1.5515264775286979,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.8523379771728723,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 1.6803443037458954,
'cinema': 0.0,
'butcher': 4.019929602075759,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 3.001107709580841,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 1.553006144007141,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 2.282404295073858,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 1.7035080488455256,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014649': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014636': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014569': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 2.5964149473904548,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014532': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014560': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014587': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 5.862852956201732,
'supermarket': 0.0},
'E01014592': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 1.574857708475501,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014706': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.3230985551234804,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 1.4079048097099174,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014642': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033366': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014553': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 2.2378515056515536,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.7433199732575078,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 1.2738277128115199,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 3.4337577594282562,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 1.4199557484897578,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 3.858567055175367,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 2.379013654222968,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 1.574857708475501,
'bar': 2.5552620732682882,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 7.772879229502235,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 1.9771839686615411,
'hairdresser': 0.5728336802390808,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014632': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014644': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 1.0318948927677074,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014733': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014588': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.0584788440987842,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 1.3407745024421331,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 1.2738277128115199,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 1.465713239050433,
'supermarket': 2.067463045991257},
'E01014487': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 0.0},
'E01014633': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 1.8178957571617955,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033362': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 1.2738277128115199,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.3230985551234804,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 2.359461878730514,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 1.2738277128115199,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014722': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014687': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014577': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 2.11645582494433,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014725': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014575': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 1.720985744153739,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.8311527947615573,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014692': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033347': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 3.0660472194531607,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 2.5552620732682882,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 1.9428344937700954,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 1.465713239050433,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014645': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 1.1373947995413651,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 2.5476554256230397,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014498': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 2.612024792365842,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 1.9428344937700954,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.569559538367049,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 3.885668987540191,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 2.1189257528257768,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.947311210542841,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 1.3407745024421331,
'college': 0.0,
'police': 1.574857708475501,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014617': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014539': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.1844164300906883,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014670': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014640': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 1.1373947995413651,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014679': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014653': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 1.5515264775286979,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 1.0332263612916814,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 2.0099648010378797,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 1.2738277128115199,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014676': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 2.419955748489758,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014601': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014651': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 2.1189257528257768,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01032518': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014521': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014664': {'food_court': 0.0,
'camp_site': 2.419955748489758,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.8599124106397561,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 1.3407745024421331,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014489': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 1.5515264775286979,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 1.9621472911018607,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.3230985551234804,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 2.572378036783578,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.4805205376133603,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014655': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 2.58587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 2.3815773992222646,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 2.282404295073858,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 3.0149472015568195,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 2.291334720956323,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014589': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014683': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 1.378563063331533,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014646': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.7433199732575078,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 1.4199557484897578,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 1.6577822829265174,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033364': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.8599124106397561,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.0584788440987842,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 1.7035080488455256,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014528': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 1.9455954342950772,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014734': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014665': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 1.8178957571617955,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 1.1373947995413651,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 1.8178957571617955,
'pub': 0.0,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 1.3407745024421331,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.5505972844934428},
'E01014526': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 5.493458384284672,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 1.553006144007141,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 1.5168657614978143,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014499': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 2.359461878730514,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014712': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 1.4595193369224884,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014563': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 3.4337577594282562,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 1.465713239050433,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014675': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.947311210542841,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014511': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014729': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 1.0318948927677074,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014641': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 2.11645582494433,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014637': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014525': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01032515': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 1.3407745024421331,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014527': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.0584788440987842,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014520': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014677': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014630': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 1.1373947995413651,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014615': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014536': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 1.6803443037458954,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 5.224049584731684,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014661': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014602': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014516': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 1.0332263612916814,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014671': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 1.292938731273915,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 3.4337577594282562,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 1.4079048097099174,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 3.3606886074917908,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.1844164300906883,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 2.5552620732682882,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 1.7185010407172423,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014562': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014571': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014621': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.7433199732575078,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 1.2738277128115199,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014522': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014622': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 1.9428344937700954,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.8523379771728723,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 1.4957157811080808,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 1.7185010407172423,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014717': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.9992383022485138,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 2.11645582494433,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014718': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014492': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014501': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 1.720985744153739,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014559': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.7433199732575078,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 2.11645582494433,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 1.465713239050433,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014581': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.569559538367049,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014534': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 1.9771839686615411,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014684': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 2.757126126663066,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.9885919843307706,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.0584788440987842,
'graveyard': 0.9885919843307706,
'recycling': 2.419955748489758,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014507': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014673': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 1.4595193369224884,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014699': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014515': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014531': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 3.4866399465150155,
'convenience': 1.034350985019132,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 1.574857708475501,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 2.1189257528257768,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 1.9151155011816035,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 3.2455186842380686,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 2.004917880836783,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014608': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.799390641798811,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01033355': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 2.1189257528257768,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 1.9621472911018607,
'recycling_glass': 0.0,
'tower': 1.720985744153739,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 1.8178957571617955,
'market_place': 0.0,
'fast_food': 1.8523379771728723,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 5.110078699088602,
'garden_centre': 0.0,
'cafe': 2.143648363986315,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 1.9428344937700954,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 2.0727287526587044,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 1.5168657614978143,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014586': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 1.3407745024421331,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 2.11645582494433,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014597': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014582': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 1.574857708475501,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014567': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.569559538367049,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 3.1459491716406855,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.7185010407172423,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014669': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.569559538367049,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014510': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 1.6418044981061142,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014570': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.569559538367049,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033352': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 2.612024792365842,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 1.8178957571617955,
'fast_food': 0.5292394220493921,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 1.378563063331533,
'doityourself': 2.359461878730514,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 1.714918691189052,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 3.2455186842380686,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 1.5168657614978143,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014613': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014701': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 3.647507297501499,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014486': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 2.3272897162930466,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.8599124106397561,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 3.9242945822037214,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.8311527947615573,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 1.378563063331533,
'doityourself': 0.0,
'playground': 1.3776351483889087,
'travel_agent': 2.282404295073858,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 2.0099648010378797,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.8401721518729477,
'community_centre': 1.1373947995413651,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 1.9151155011816035,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.7185010407172423,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014548': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 1.0332263612916814,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014506': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 2.359461878730514,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 3.894622421085682,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014508': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 2.11645582494433,
'stadium': 1.6418044981061142,
'toy_shop': 1.378563063331533,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 2.452684113877326,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 1.9428344937700954,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.0584788440987842,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 3.001107709580841,
'newsagent': 1.6803443037458954,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.4805205376133603,
'laundry': 1.9151155011816035,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 1.9151155011816035,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 1.465713239050433,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 1.1456673604781615,
'gift_shop': 3.4236064426107866,
'university': 0.0,
'supermarket': 0.0},
'E01014579': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 1.8178957571617955,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014678': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.0584788440987842,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033353': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 1.8178957571617955,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 1.378563063331533,
'doityourself': 1.5729745858203428,
'playground': 0.0,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 2.520516455618843,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 2.572378036783578,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 2.243573671662121,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 2.9610410752267207,
'laundry': 0.0,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 2.379013654222968,
'dentist': 1.4595193369224884,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 5.862852956201732,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014624': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01032514': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014509': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 1.6418044981061142,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014726': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014690': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 2.1189257528257768,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014702': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014626': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 2.8775258203395904,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 1.465713239050433,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014572': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 2.2378515056515536,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 2.3244266310100103,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.9621472911018607,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.8523379771728723,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 2.431671531667666,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 3.1505852413149444,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014735': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 1.3776351483889087,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 2.419955748489758,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014714': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 1.9428344937700954,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.0,
'pitch': 1.569559538367049,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 2.5476554256230397,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 1.3407745024421331,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 2.1189257528257768,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 1.465713239050433,
'supermarket': 0.0},
'E01014715': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 1.378563063331533,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 2.038779176986542,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 1.9151155011816035,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014491': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014555': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014610': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014689': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 1.0318948927677074,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.3230985551234804,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 1.4079048097099174,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.0,
'community_centre': 1.7060921993120477,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 4.2378515056515536,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014631': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014666': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.569559538367049,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014502': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 1.034350985019132,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 2.11645582494433,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014523': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014596': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014638': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 1.5515264775286979,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.0584788440987842,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014682': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014659': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014705': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014493': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 1.292938731273915,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.714918691189052,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 1.7185010407172423,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01033367': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 3.4337577594282562,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 1.4199557484897578,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 1.6803443037458954,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 1.3407745024421331,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 1.3407745024421331,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 0.0},
'E01014623': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.799390641798811,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014497': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014700': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 1.6418044981061142,
'toy_shop': 0.0,
'bookshop': 1.2738277128115199,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 2.943220936652791,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.714918691189052,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 1.4957157811080808,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014668': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 1.7035080488455256,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 2.757126126663066,
'bookshop': 1.2738277128115199,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.9621472911018607,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 2.143648363986315,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 2.2378515056515536,
'castle': 0.0,
'bakery': 1.4957157811080808,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.1844164300906883,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 4.564808590147716,
'university': 0.0,
'supermarket': 1.5505972844934428},
'E01033356': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014584': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014561': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 1.0318948927677074,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 1.7220439354861359,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 1.1373947995413651,
'sports_centre': 0.0,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014694': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014556': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 1.5168657614978143,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014732': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.714918691189052,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 1.465713239050433,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014627': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 1.6577822829265174,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014708': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 3.6623055895231147,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014709': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 2.5476554256230397,
'library': 0.0,
'pitch': 5.231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 2.1189257528257768,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 1.5168657614978143,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 6.34936747483299,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014544': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.9575577505908017,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 3.5685204813344518,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 2.6815490048842663,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 2.5476554256230397,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033361': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 2.918393151442616,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 1.378563063331533,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 2.282404295073858,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.3230985551234804,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 1.6803443037458954,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 2.143648363986315,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.1844164300906883,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 1.4595193369224884,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 1.6577822829265174,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 1.4199557484897578,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 2.5777515610758632,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014691': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 2.354339307550574,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.799390641798811,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 1.553006144007141,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014658': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 5.505411050371199,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 1.574857708475501,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 1.720985744153739,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 2.1189257528257768,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 1.574857708475501,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.5505972844934428},
'E01014524': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014546': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 2.3244266310100103,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 3.8214831384345596,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 7.358052341631978,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 1.9771839686615411,
'recycling': 0.0,
'pharmacy': 1.4079048097099174,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.0,
'clothes': 10.92223797434832,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 2.0440314796354406,
'garden_centre': 0.0,
'cafe': 3.858567055175367,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 2.3688328601813766,
'laundry': 1.9151155011816035,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 1.4595193369224884,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 1.189506827111484,
'florist': 1.9151155011816035,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 10.190621702492159,
'shoe_shop': 1.465713239050433,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 2.004917880836783,
'gift_shop': 2.282404295073858,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014629': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014519': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014672': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.8311527947615573,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.799390641798811,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 5.095310851246079,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014500': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.517175492509566,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014517': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014686': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014639': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014616': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 1.378563063331533,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 1.720985744153739,
'cafe': 1.286189018391789,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 1.9771839686615411,
'hairdresser': 2.5777515610758632,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014713': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 1.9428344937700954,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 2.11645582494433,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014593': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014681': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.7433199732575078,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.432084200597702,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014547': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033358': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 1.4199557484897578,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 2.0440314796354406,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.8401721518729477,
'community_centre': 1.1373947995413651,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 1.8178957571617955,
'pub': 1.1844164300906883,
'laundry': 0.0,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 1.574857708475501,
'bar': 2.5552620732682882,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 2.590959743167412,
'public_building': 0.0,
'arts_centre': 1.4199557484897578,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014696': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 1.2738277128115199,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014540': {'food_court': 2.1189257528257768,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 1.7035080488455256,
'sports_shop': 0.0,
'memorial': 2.1189257528257768,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 4.067746604267518,
'convenience': 1.8101142237834809,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 5.291139562360825,
'stadium': 0.0,
'toy_shop': 2.757126126663066,
'bookshop': 7.642966276869119,
'park': 0.6879299285118049,
'outdoor_shop': 3.149715416951002,
'bank': 9.129617180295432,
'restaurant': 25.50791478432419,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 3.885668987540191,
'fire_station': 0.0,
'market_place': 1.8178957571617955,
'fast_food': 7.1447321976667935,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 1.4079048097099174,
'mall': 11.359645987918062,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 6.847212885221573,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 21.00430379682369,
'cinema': 3.149715416951002,
'butcher': 1.0049824005189398,
'hotel': 10.220157398177204,
'garden_centre': 0.0,
'cafe': 15.862997893498731,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 1.553006144007141,
'kindergarten': 0.679593058995514,
'courthouse': 1.9428344937700954,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 3.739289452770202,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 9.475331440725506,
'laundry': 0.0,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 2.1189257528257768,
'mobile_phone_shop': 10.27081932783236,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 2.1892790053837325,
'museum': 1.3407745024421331,
'college': 2.431671531667666,
'police': 0.0,
'bar': 17.886834512878018,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.9922426784517993,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 4.550597284493443,
'observation_tower': 4.839911496979516,
'chemist': 0.0,
'doctors': 2.4866734243897763,
'attraction': 6.7038725122106655,
'hostel': 5.828503481310286,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 2.8399114969795156,
'jeweller': 6.369138564057599,
'shoe_shop': 8.794279434302599,
'artwork': 0.0,
'nightclub': 7.328566195252165,
'optician': 1.9771839686615411,
'hairdresser': 5.1555031221517265,
'gift_shop': 7.988415032758502,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014634': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 2.359461878730514,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01033350': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 2.11645582494433,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.286189018391789,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 2.3295092160107114,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 2.419955748489758,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 5.363098009768533,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 2.1189257528257768,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 5.363098009768533,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 2.8399114969795156,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014723': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014530': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014635': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014496': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 1.720985744153739,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 3.635791514323591,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 2.0440314796354406,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014573': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 1.9771839686615411,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014598': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 1.6803443037458954,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 1.2982074736952274,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014551': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 1.4199557484897578,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 1.574857708475501,
'butcher': 1.0049824005189398,
'hotel': 1.0220157398177203,
'garden_centre': 1.720985744153739,
'cafe': 1.286189018391789,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.9575577505908017,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 2.282404295073858,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 3.9844853569035985,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 1.3407745024421331,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.0,
'gift_shop': 1.141202147536929,
'university': 1.465713239050433,
'supermarket': 1.0337315229956285},
'E01014603': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014680': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 2.419955748489758,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014542': {'food_court': 2.1189257528257768,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 2.3244266310100103,
'convenience': 0.517175492509566,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 3.174683737416495,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 3.8214831384345596,
'park': 0.6879299285118049,
'outdoor_shop': 3.149715416951002,
'bank': 4.564808590147716,
'restaurant': 9.810736455509304,
'recycling_glass': 0.0,
'tower': 3.441971488307478,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 3.440056243321049,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 5.514252253326132,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 12.602582278094216,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 3.0660472194531607,
'garden_centre': 0.0,
'cafe': 10.718241819931574,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 2.1189257528257768,
'department_store': 1.8178957571617955,
'pub': 3.553249290272065,
'laundry': 0.0,
'theatre': 2.757126126663066,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 2.282404295073858,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 4.0223235073264,
'college': 1.215835765833833,
'police': 1.574857708475501,
'bar': 13.628064390764205,
'hospital': 5.94753413555742,
'florist': 1.9151155011816035,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.9922426784517993,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 5.828503481310286,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 5.679822993959031,
'jeweller': 1.2738277128115199,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 10.25999267335303,
'optician': 2.9657759529923116,
'hairdresser': 4.296252601793106,
'gift_shop': 2.282404295073858,
'university': 5.862852956201732,
'supermarket': 1.0337315229956285},
'E01014594': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014716': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014695': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 2.1189257528257768,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 1.6418044981061142,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.8599124106397561,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 4.185492102312131,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 1.378563063331533,
'doityourself': 0.7864872929101714,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 1.720985744153739,
'cafe': 1.286189018391789,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 1.4957157811080808,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.1844164300906883,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 2.931426478100866,
'supermarket': 0.0},
'E01032517': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014703': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014537': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 3.2455186842380686,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014533': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 1.574857708475501,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 3.647507297501499,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014647': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014711': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 4.23291164988866,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014565': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 3.2455186842380686,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01032516': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 1.0332263612916814,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.799390641798811,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 2.1189257528257768,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 1.3407745024421331,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014574': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 3.0149472015568195,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 2.1189257528257768,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014529': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014514': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.7433199732575078,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 4.905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 1.9428344937700954,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.8523379771728723,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.7864872929101714,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 1.6803443037458954,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 4.716026400769893,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 1.553006144007141,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 1.141202147536929,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 1.189506827111484,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 1.2738277128115199,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 3.4370020814344846,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.5505972844934428},
'E01014662': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014607': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 1.574857708475501,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 1.553006144007141,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 1.306012396182921,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014620': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014505': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014495': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 1.4595193369224884,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014663': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 2.1189257528257768,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 1.3407745024421331,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014535': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014667': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014513': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 1.9428344937700954,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014595': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014580': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014552': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 1.1622133155050052,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 1.4199557484897578,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 1.4595193369224884,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.9922426784517993,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01032519': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014688': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.7757632387643489,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.8523379771728723,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014719': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 2.3244266310100103,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 1.378563063331533,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 5.706010737684645,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 1.714918691189052,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.1844164300906883,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.9922426784517993,
'water_works': 0.0,
'computer_shop': 2.379013654222968,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 1.6577822829265174,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 1.2738277128115199,
'shoe_shop': 1.465713239050433,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 1.9771839686615411,
'hairdresser': 2.004917880836783,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 1.5505972844934428},
'E01014557': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.569559538367049,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 1.3407745024421331,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014710': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 1.215835765833833,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014693': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.7938591330740882,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014578': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.6879299285118049,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014707': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014650': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014730': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.8599124106397561,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014698': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 1.378563063331533,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 2.143648363986315,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 1.215835765833833,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 2.8399114969795156,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033369': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 1.9428344937700954,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 1.378563063331533,
'doityourself': 0.0,
'playground': 1.3776351483889087,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014599': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014674': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014619': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 2.5476554256230397,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 1.4716104683263955,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.8401721518729477,
'cinema': 1.574857708475501,
'butcher': 1.0049824005189398,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 2.038779176986542,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 1.215835765833833,
'shelter': 1.189506827111484,
'dentist': 2.1892790053837325,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.8592505203586212,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014538': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 1.378563063331533,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 1.714918691189052,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 1.553006144007141,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.4805205376133603,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.9922426784517993,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 1.4199557484897578,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 8.794279434302599,
'supermarket': 0.5168657614978143},
'E01014736': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033342': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 2.1189257528257768,
'beverages': 0.0,
'sports_shop': 1.1189257528257768,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 4.648853262020021,
'convenience': 1.034350985019132,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 1.378563063331533,
'bookshop': 2.5476554256230397,
'park': 0.5159474463838537,
'outdoor_shop': 4.724573125426503,
'bank': 4.564808590147716,
'restaurant': 1.9621472911018607,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 1.9428344937700954,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 4.233915376395137,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 2.8399114969795156,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.0,
'clothes': 19.323959493077798,
'cinema': 1.574857708475501,
'butcher': 0.0,
'hotel': 1.0220157398177203,
'garden_centre': 0.0,
'cafe': 4.716026400769893,
'newsagent': 0.8401721518729477,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 1.9428344937700954,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 1.4957157811080808,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 3.635791514323591,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 12.553223622906218,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 1.189506827111484,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 1.727306495444941,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 1.2738277128115199,
'shoe_shop': 5.862852956201732,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 2.291334720956323,
'gift_shop': 2.282404295073858,
'university': 0.0,
'supermarket': 0.0},
'E01033344': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 1.2738277128115199,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 2.943220936652791,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 1.2738277128115199,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 1.1990859626982164,
'clothes': 2.520516455618843,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 3.0660472194531607,
'garden_centre': 0.0,
'cafe': 3.001107709580841,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.7766246451360326,
'laundry': 0.0,
'theatre': 1.378563063331533,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 2.6815490048842663,
'college': 1.215835765833833,
'police': 0.0,
'bar': 1.7035080488455256,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 2.6815490048842663,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 1.2738277128115199,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 1.465713239050433,
'optician': 0.0,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 1.465713239050433,
'supermarket': 0.5168657614978143},
'E01014494': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.9885919843307706,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 1.189506827111484,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01033348': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 1.5515264775286979,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 1.058227912472165,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 2.943220936652791,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.0584788440987842,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.8401721518729477,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 1.4199557484897578,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014720': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 1.359186117991028,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014564': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.4905368227754652,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014605': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 1.306012396182921,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.5877182661481763,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.6491037368476137,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 1.1456673604781615,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.5505972844934428},
'E01014504': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014488': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.5995429813491082,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 1.5168657614978143,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014583': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014643': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 3.2836089962122283,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.307966281972541,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014727': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.9885919843307706,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 1.215835765833833,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014609': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.0,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014545': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.8517540244227628,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 1.2738277128115199,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 1.0463730255780328,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 1.4199557484897578,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 2.520516455618843,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 2.143648363986315,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 2.2378515056515536,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 1.058227912472165,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 3.8214831384345596,
'shoe_shop': 1.465713239050433,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 2.282404295073858,
'university': 0.0,
'supermarket': 0.0},
'E01014721': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.0,
'car_wash': 0.9727977171475386,
'golf_course': 1.3407745024421331,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.9810736455509304,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 3.8214831384345596,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 1.378563063331533,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014590': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.5811066577525026,
'convenience': 0.7757632387643489,
'car_wash': 0.9727977171475386,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 1.0318948927677074,
'outdoor_shop': 0.0,
'bank': 2.282404295073858,
'restaurant': 1.9621472911018607,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 2.1169576881975685,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 1.378563063331533,
'doityourself': 0.0,
'playground': 0.34440878709722716,
'travel_agent': 1.141202147536929,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 1.0049824005189398,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.857459345594526,
'newsagent': 0.8401721518729477,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.8883123225680163,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.7297596684612442,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.8517540244227628,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 2.1189257528257768,
'doctors': 1.6577822829265174,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 1.9771839686615411,
'hairdresser': 1.432084200597702,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014614': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.517175492509566,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.2615932563945082,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 1.058227912472165,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.799390641798811,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.9575577505908017,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.6640808928172665,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.5728336802390808,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014485': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.5159474463838537,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 1.378563063331533,
'doityourself': 0.0,
'playground': 0.6888175741944543,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.3996953208994055,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.8401721518729477,
'community_centre': 1.1373947995413651,
'sports_centre': 1.553006144007141,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 2.419955748489758,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.5922082150453442,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.8636532477224705,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.5168657614978143},
'E01014558': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 2.5552620732682882,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 2.9055332887625127,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 1.2738277128115199,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 1.141202147536929,
'restaurant': 6.8675155188565125,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 1.8523379771728723,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 1.5729745858203428,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.19984766044970276,
'clothes': 2.520516455618843,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 3.429837382378104,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.7765030720035705,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 1.1189257528257768,
'castle': 0.0,
'bakery': 0.7478578905540404,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 1.4805205376133603,
'laundry': 0.9575577505908017,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 1.2738277128115199,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 4.258770122113814,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 1.328161785634533,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.8288911414632587,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.9885919843307706,
'hairdresser': 2.5777515610758632,
'gift_shop': 1.141202147536929,
'university': 0.0,
'supermarket': 1.0337315229956285},
'E01014660': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.7757632387643489,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.34396496425590245,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.5231865127890164,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.5292394220493921,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.7039524048549587,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 1.3776351483889087,
'travel_agent': 0.0,
'archaeological': 3.885668987540191,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.428729672797263,
'newsagent': 0.0,
'community_centre': 1.7060921993120477,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 9.101194568986886,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 1.3407745024421331,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.2864168401195404,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014704': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.17198248212795123,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.0,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.0,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.0,
'sports_centre': 0.0,
'kindergarten': 0.0,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.2961041075226721,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0},
'E01014685': {'food_court': 0.0,
'camp_site': 0.0,
'theme_park': 0.0,
'beverages': 0.0,
'sports_shop': 0.0,
'memorial': 0.0,
'car_rental': 0.0,
'nan': 0.0,
'beauty_shop': 0.0,
'convenience': 0.258587746254783,
'car_wash': 0.0,
'golf_course': 0.0,
'bicycle_shop': 0.0,
'stadium': 0.0,
'toy_shop': 0.0,
'bookshop': 0.0,
'park': 0.0,
'outdoor_shop': 0.0,
'bank': 0.0,
'restaurant': 0.0,
'recycling_glass': 0.0,
'tower': 0.0,
'toilet': 0.0,
'library': 0.0,
'pitch': 0.7847797691835245,
'prison': 0.0,
'video_shop': 0.0,
'fire_station': 0.0,
'market_place': 0.0,
'fast_food': 0.26461971102469606,
'graveyard': 0.0,
'recycling': 0.0,
'pharmacy': 0.0,
'mall': 0.0,
'post_office': 0.0,
'water_tower': 0.0,
'veterinary': 0.0,
'stationery': 0.0,
'doityourself': 0.0,
'playground': 0.0,
'travel_agent': 0.0,
'archaeological': 0.0,
'school': 0.0,
'clothes': 0.0,
'cinema': 0.0,
'butcher': 0.0,
'hotel': 0.0,
'garden_centre': 0.0,
'cafe': 0.0,
'newsagent': 0.0,
'community_centre': 0.5686973997706826,
'sports_centre': 0.0,
'kindergarten': 0.679593058995514,
'courthouse': 0.0,
'comms_tower': 0.0,
'greengrocer': 0.0,
'castle': 0.0,
'bakery': 0.0,
'recycling_metal': 0.0,
'caravan_site': 0.0,
'wastewater_plant': 0.0,
'department_store': 0.0,
'pub': 0.0,
'laundry': 0.0,
'theatre': 0.0,
'track': 0.0,
'monument': 0.0,
'mobile_phone_shop': 0.0,
'nursing_home': 0.0,
'car_dealership': 0.0,
'guesthouse': 0.0,
'shelter': 0.0,
'dentist': 0.0,
'museum': 0.0,
'college': 0.0,
'police': 0.0,
'bar': 0.0,
'hospital': 0.0,
'florist': 0.0,
'tourist_info': 0.0,
'town_hall': 0.0,
'clinic': 0.0,
'water_works': 0.0,
'computer_shop': 0.0,
'ruins': 0.0,
'observation_tower': 0.0,
'chemist': 0.0,
'doctors': 0.0,
'attraction': 0.0,
'hostel': 0.0,
'swimming_pool': 0.0,
'fountain': 0.0,
'picnic_site': 0.0,
'furniture_shop': 0.0,
'public_building': 0.0,
'arts_centre': 0.0,
'jeweller': 0.0,
'shoe_shop': 0.0,
'artwork': 0.0,
'nightclub': 0.0,
'optician': 0.0,
'hairdresser': 0.0,
'gift_shop': 0.0,
'university': 0.0,
'supermarket': 0.0}}
tfidfDic
is a dictionary of dictionary. The inner is a dictionary of words with their tfidf scores, and the outer is the document dictionary.
Again, we can convert the tfidf dictionary to a dataframe
by using code:
doc2wordTFIDFMatrix = pd.DataFrame.from_dict(tfidfDic, orient='index').rename_axis('LSOA11 Code').reset_index()
doc2wordTFIDFMatrix.head()
LSOA11 Code | food_court | camp_site | theme_park | beverages | sports_shop | memorial | car_rental | nan | beauty_shop | ... | arts_centre | jeweller | shoe_shop | artwork | nightclub | optician | hairdresser | gift_shop | university | supermarket | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | E01014612 | 0.0 | 0.0 | 0.0 | 0.0 | 0.000000 | 0.0 | 0.0 | 0.0 | 0.000000 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.000000 | 0.0 | 0.0 | 0.0 |
1 | E01014512 | 0.0 | 0.0 | 0.0 | 0.0 | 0.000000 | 0.0 | 0.0 | 0.0 | 0.000000 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.572834 | 0.0 | 0.0 | 0.0 |
2 | E01014618 | 0.0 | 0.0 | 0.0 | 0.0 | 0.000000 | 0.0 | 0.0 | 0.0 | 0.581107 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.572834 | 0.0 | 0.0 | 0.0 |
3 | E01014576 | 0.0 | 0.0 | 0.0 | 0.0 | 1.118926 | 0.0 | 0.0 | 0.0 | 0.581107 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.000000 | 0.0 | 0.0 | 0.0 |
4 | E01033370 | 0.0 | 0.0 | 0.0 | 0.0 | 0.000000 | 0.0 | 0.0 | 0.0 | 0.000000 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.000000 | 0.0 | 0.0 | 0.0 |
5 rows × 106 columns
Different from doc2wordMatrix
where the term frequency is recorded, this doc2wordTFIDFMatrix
records the TF-IDF values. As we have discussed in the lecture, the TF-IDF can better represent the uniqueness of terms in a document.
So far, we have mannually implemented the TF-IDF computation. In fact, we can also achieve the same using existing packages:
## automatically compute the IF-IDF using packages sklearn
from sklearn.feature_extraction.text import TfidfVectorizer
tfidf = TfidfVectorizer()
TfidfVectorizer()
here is to initialize a Tfidf vector, which we will use in the next steps to fit the TF-IDF scores from the documents. Before that, we also have to prepare a list of string: docList
as the input for the next step:
docList = list(bristol_lsoal_poi_full['fclass'].apply(' '.join))
docList
['park playground swimming_pool swimming_pool',
'nursing_home hairdresser computer_shop fast_food restaurant doityourself restaurant sports_centre hairdresser sports_centre doityourself car_dealership restaurant dentist kindergarten community_centre cafe doityourself butcher theatre sports_centre convenience convenience college car_wash convenience clinic',
'kindergarten convenience hairdresser fast_food community_centre playground park park convenience cafe fast_food fast_food hairdresser beauty_shop kindergarten',
'sports_shop beauty_shop convenience fast_food pub car_dealership playground pitch pitch toy_shop playground',
'convenience restaurant kindergarten school pitch park pitch playground pub hotel fast_food fast_food college pitch car_dealership furniture_shop pub hotel',
'car_dealership doityourself car_wash doityourself doityourself convenience beauty_shop fast_food park pharmacy hairdresser pub fast_food',
'sports_centre greengrocer bakery gift_shop pub convenience pub pub pub restaurant',
'restaurant cafe cafe restaurant gift_shop florist restaurant fast_food restaurant beverages hairdresser beverages restaurant restaurant cafe cafe arts_centre attraction bar pub restaurant pub restaurant nightclub bar restaurant restaurant pub pub restaurant pub cafe cinema hotel tourist_info pub park fast_food fast_food beauty_shop bar fast_food supermarket furniture_shop fast_food restaurant bar hairdresser convenience fast_food fast_food fast_food fast_food cafe fast_food restaurant restaurant beauty_shop pub restaurant cafe hairdresser fountain fountain clothes fast_food bar restaurant theatre supermarket pub pharmacy convenience restaurant hairdresser fast_food hairdresser bar theatre hairdresser arts_centre museum hairdresser sports_centre cafe restaurant toilet cafe restaurant community_centre hairdresser cafe restaurant cafe clinic museum school cafe library park fountain park park fountain town_hall park cafe fountain cafe fast_food restaurant bar hairdresser nightclub restaurant clothes nightclub beverages pub restaurant nightclub',
'car_dealership convenience doityourself hairdresser convenience doityourself nursing_home convenience bakery school car_dealership hairdresser park playground school',
'college park park track fast_food sports_centre park school pitch',
'cafe restaurant doityourself pub fast_food fast_food pub clinic sports_shop outdoor_shop convenience fast_food restaurant pub veterinary sports_centre pub convenience playground pub clinic dentist',
'hairdresser convenience pub fast_food car_wash clothes playground bakery butcher convenience restaurant restaurant fast_food hairdresser restaurant hairdresser park playground pub park restaurant convenience supermarket beauty_shop restaurant pub convenience supermarket pharmacy butcher restaurant restaurant fast_food travel_agent supermarket restaurant hairdresser clothes cafe travel_agent pharmacy bakery cafe hairdresser convenience butcher gift_shop mobile_phone_shop fast_food fast_food clothes fast_food restaurant fast_food beauty_shop supermarket toy_shop pub cafe',
'car_wash pitch park mobile_phone_shop bank mobile_phone_shop hairdresser gift_shop travel_agent cafe pub cafe mobile_phone_shop hairdresser bank cafe toilet nightclub convenience outdoor_shop furniture_shop pub cafe fast_food pub fast_food fast_food greengrocer cafe butcher arts_centre fast_food fast_food shoe_shop clothes mobile_phone_shop fast_food beauty_shop fast_food chemist cafe beverages cafe hairdresser mobile_phone_shop playground mobile_phone_shop hairdresser jeweller beauty_shop beauty_shop pub optician pub fast_food pub hairdresser fast_food cafe furniture_shop pharmacy doctors restaurant library clinic hairdresser optician mobile_phone_shop post_office clothes car_dealership supermarket hairdresser convenience sports_centre car_rental',
'playground tower pitch',
'restaurant bookshop restaurant hairdresser park laundry pub kindergarten pub university swimming_pool restaurant school fast_food hairdresser fast_food hairdresser dentist university clinic hotel hotel cafe convenience pub convenience bar',
'community_centre school pitch park convenience pitch park',
'school post_office',
'toilet pitch shelter park playground pitch pitch pitch mall fast_food hairdresser florist bakery hairdresser car_wash community_centre bank bank fast_food gift_shop dentist kindergarten veterinary school kindergarten fast_food newsagent clinic pub park graveyard cafe',
'pitch pitch pub school doityourself convenience beauty_shop beverages fast_food car_dealership optician convenience post_office fast_food beverages restaurant pub bakery fast_food restaurant beverages bakery beauty_shop hairdresser fast_food pharmacy fast_food pub furniture_shop newsagent beverages hairdresser convenience dentist pitch',
'bar bar cafe school sports_centre fast_food school fast_food cafe community_centre cafe convenience furniture_shop cafe clothes optician hairdresser convenience computer_shop fast_food greengrocer restaurant fast_food hairdresser butcher cafe cafe butcher fast_food mobile_phone_shop convenience travel_agent fast_food mobile_phone_shop furniture_shop butcher pharmacy cafe park pitch pitch hairdresser butcher restaurant fast_food convenience restaurant playground park pitch car_dealership park convenience convenience school playground park clothes clinic library sports_centre bicycle_shop',
'doctors fast_food restaurant restaurant hairdresser hairdresser community_centre veterinary pub graveyard',
'pub convenience playground pitch school cafe fast_food',
'pub greengrocer cafe beverages convenience park community_centre car_dealership pharmacy hairdresser car_dealership car_dealership car_dealership pitch',
'hairdresser newsagent hairdresser',
'dentist beauty_shop bank restaurant hairdresser hairdresser convenience fast_food fast_food restaurant hairdresser bakery clinic swimming_pool clinic park doctors',
'university hospital school pitch pharmacy nursing_home university university university cafe park fast_food restaurant hairdresser pitch park playground pitch park hairdresser convenience convenience',
'park fast_food fast_food pub police cafe hairdresser supermarket clinic guesthouse park beauty_shop kindergarten community_centre park park',
'hairdresser cafe convenience optician fast_food pharmacy pharmacy veterinary fast_food convenience hairdresser convenience fast_food fast_food fast_food library school school kindergarten',
'park hairdresser hairdresser convenience convenience fast_food fast_food florist school park',
'convenience playground library cafe park park pitch playground community_centre park',
'pitch sports_centre supermarket mall laundry optician newsagent cafe pub bar doctors furniture_shop convenience cafe cafe hairdresser beauty_shop cafe bar restaurant beauty_shop library furniture_shop furniture_shop restaurant cafe furniture_shop furniture_shop furniture_shop clinic supermarket community_centre furniture_shop restaurant restaurant doityourself furniture_shop furniture_shop police cafe kindergarten shelter cafe cafe school sports_shop fast_food pub pub optician beauty_shop restaurant kindergarten greengrocer hairdresser convenience cafe gift_shop bookshop restaurant fast_food pharmacy restaurant bicycle_shop bar sports_shop clothes toilet shelter',
'playground pitch sports_centre hospital laundry pharmacy hairdresser fast_food hairdresser hairdresser supermarket fast_food convenience doityourself dentist community_centre pitch doctors pitch school pitch',
'park pitch pitch convenience beauty_shop hairdresser park park beauty_shop fast_food cafe park park dentist park playground convenience golf_course library community_centre swimming_pool school',
'park doityourself playground pub convenience pub fast_food park',
'library car_dealership supermarket fast_food fast_food fast_food supermarket clothes hairdresser supermarket pub museum university bank pub school beauty_shop hairdresser pitch restaurant beauty_shop jeweller mobile_phone_shop optician supermarket fast_food travel_agent playground park park hospital school park',
'hairdresser clothes sports_shop furniture_shop cafe clinic gift_shop pitch school kindergarten fast_food restaurant greengrocer mobile_phone_shop park playground shelter park',
'playground pitch school pub car_rental car_dealership convenience beauty_shop hospital fire_station',
'doityourself florist fast_food hairdresser fast_food beverages restaurant fast_food hairdresser restaurant convenience newsagent pub doityourself supermarket bicycle_shop fast_food car_dealership pub cafe furniture_shop hairdresser hairdresser car_dealership pub park bar bookshop clothes doityourself furniture_shop cafe fast_food cafe beauty_shop beauty_shop jeweller clinic',
'park',
'school doctors convenience car_dealership car_rental fast_food supermarket pitch pitch',
'park park school pitch swimming_pool swimming_pool cafe park school hairdresser playground convenience pitch nursing_home pub convenience park',
'pub park',
'pitch graveyard kindergarten beverages pub hairdresser pitch convenience pitch pitch park pitch pitch playground pitch school tower',
'convenience hairdresser playground pitch fast_food beverages hairdresser fast_food beauty_shop doityourself pitch car_wash school pitch park',
'hotel bar convenience park theatre playground park cafe playground school school college hotel bar pub hostel nightclub hotel park bar cafe hairdresser graveyard restaurant restaurant convenience hairdresser',
'clinic nursing_home nursing_home hairdresser hairdresser park fast_food newsagent hairdresser community_centre community_centre hairdresser computer_shop fast_food beverages pitch pitch pitch',
'park playground park convenience museum archaeological car_dealership school track pitch pitch car_rental car_rental bakery fast_food fast_food car_dealership archaeological wastewater_plant recycling_glass pitch pitch pitch pitch police car_dealership park shelter',
'car_dealership pitch pitch pitch swimming_pool car_dealership clinic hairdresser doityourself clinic',
'bakery supermarket playground pub pub park convenience sports_centre fast_food dentist cafe pub pub convenience fast_food restaurant',
'beauty_shop fast_food beauty_shop restaurant hairdresser convenience veterinary dentist',
'playground kindergarten pitch pitch sports_centre community_centre pitch pitch school community_centre school pitch',
'car_dealership pub fast_food hairdresser',
'post_office convenience butcher fast_food travel_agent restaurant furniture_shop convenience laundry cafe butcher school mobile_phone_shop beauty_shop cafe convenience supermarket convenience fast_food pub park playground playground park convenience playground park restaurant pub community_centre park pitch pitch school jeweller convenience',
'nan',
'park park park hospital school doctors community_centre park',
'community_centre doctors swimming_pool water_tower kindergarten pub park',
'school convenience library',
'library pitch pitch school pitch pitch park school',
'camp_site school pitch car_rental supermarket park pitch park hospital park park attraction park supermarket',
'community_centre fast_food convenience cafe restaurant bank convenience butcher convenience convenience cafe supermarket pub restaurant hairdresser fast_food cafe bar cafe convenience fast_food convenience bakery hairdresser cafe restaurant restaurant fast_food pub pitch cafe school doctors fast_food clinic pitch school pub park playground pitch playground park pub pub',
'playground pitch pub convenience fast_food hairdresser fast_food fast_food convenience cafe restaurant fast_food pharmacy hairdresser bicycle_shop convenience convenience fast_food hairdresser beauty_shop restaurant community_centre hairdresser park butcher dentist mobile_phone_shop clothes convenience hairdresser convenience butcher travel_agent restaurant florist laundry fast_food travel_agent hairdresser convenience car_wash clinic fast_food convenience pub greengrocer fast_food fast_food hairdresser butcher convenience convenience hairdresser park park clinic',
'pitch community_centre convenience fast_food fast_food pub park park',
'park bakery hairdresser toy_shop cafe school school newsagent pub',
'park school convenience community_centre cafe optician newsagent fast_food hairdresser beverages travel_agent doctors doctors mall fast_food hairdresser bicycle_shop bar convenience florist beauty_shop bakery beauty_shop beauty_shop',
'sports_shop convenience fast_food convenience fast_food car_dealership car_dealership fast_food hairdresser restaurant school doctors pub restaurant fast_food park park playground park community_centre park beverages bakery park bar bar',
'pitch pitch pitch pitch park playground pub park cafe park pharmacy fast_food fast_food doctors cafe water_works car_wash car_dealership car_wash supermarket',
'pub cafe toilet playground pitch pitch park pitch school community_centre playground pitch shelter',
'community_centre supermarket market_place furniture_shop fast_food supermarket pharmacy cafe department_store doctors furniture_shop bicycle_shop hairdresser school community_centre school supermarket laundry car_wash playground fast_food convenience veterinary hairdresser school park park attraction',
'pitch pitch school fast_food swimming_pool pitch pitch hotel pitch pitch kindergarten pitch pitch pitch sports_centre pitch pitch pitch cafe school car_dealership pitch pitch pitch cafe doityourself doityourself sports_centre car_dealership pitch ruins water_works pitch pitch pitch pitch playground park pitch cafe',
'park nursing_home hairdresser community_centre pub convenience car_dealership school pitch park park doityourself convenience dentist doityourself doityourself fast_food fast_food',
'beauty_shop bicycle_shop beverages restaurant supermarket restaurant fast_food dentist beauty_shop hairdresser hairdresser swimming_pool pitch school dentist playground college',
'furniture_shop hotel shoe_shop pub hairdresser fast_food restaurant pub clothes convenience bar restaurant fast_food laundry restaurant beauty_shop restaurant restaurant restaurant furniture_shop restaurant',
'school computer_shop hairdresser pub beauty_shop car_dealership fast_food convenience convenience hairdresser hairdresser car_dealership car_dealership pub pub',
'supermarket furniture_shop school',
'park shelter playground park pitch park park pitch pitch park doityourself pub doityourself school park',
'park school pitch kindergarten swimming_pool swimming_pool park',
'car_wash convenience pharmacy supermarket dentist park doctors community_centre',
'pub school fast_food cafe veterinary library',
'attraction school school sports_centre playground park',
'fast_food clinic convenience beauty_shop fast_food butcher hairdresser fast_food fast_food convenience pub pitch pitch pub pitch graveyard park school playground',
'pub hairdresser fast_food park convenience graveyard school',
'pub kindergarten community_centre car_dealership park graveyard',
'convenience beverages hairdresser fast_food beauty_shop hairdresser fast_food supermarket supermarket fast_food greengrocer park pitch school school school pitch community_centre park playground community_centre',
'hairdresser supermarket pitch pitch swimming_pool pitch pitch pitch school school school',
'track track golf_course pitch track school track playground park community_centre park playground car_dealership furniture_shop clothes furniture_shop sports_shop clothes fast_food',
'car_wash doctors pitch playground school nursing_home convenience doityourself convenience hairdresser car_dealership',
'pitch school school pharmacy doctors supermarket community_centre doityourself',
'park school playground playground pitch playground park',
'pharmacy restaurant fast_food hairdresser hairdresser restaurant clothes clothes bakery pharmacy hairdresser mobile_phone_shop clothes pub fast_food laundry convenience florist restaurant restaurant pub furniture_shop optician hairdresser clothes clinic bar restaurant cafe convenience restaurant convenience greengrocer fast_food hairdresser fast_food bar fast_food pub hairdresser computer_shop supermarket convenience fast_food beverages convenience restaurant dentist cafe cafe bar pitch supermarket pub swimming_pool park playground',
'community_centre hairdresser pub fast_food pub bakery fast_food pub restaurant restaurant convenience',
'park pitch pub pitch pitch school',
'hairdresser beauty_shop hairdresser fast_food furniture_shop beauty_shop jeweller library florist pub hairdresser beauty_shop supermarket clothes',
'playground pitch school',
'supermarket pharmacy beverages hairdresser convenience bakery cafe video_shop convenience fast_food beauty_shop fast_food fast_food clothes hairdresser car_dealership cafe florist butcher hairdresser fast_food fast_food optician bakery convenience newsagent post_office hairdresser fast_food beauty_shop fast_food hairdresser hairdresser school',
'park swimming_pool swimming_pool school kindergarten park sports_centre school school pitch pitch school school veterinary',
'school golf_course park',
'hairdresser pub golf_course convenience pitch school bar kindergarten water_works playground park',
'garden_centre doityourself pitch pitch community_centre convenience school pitch car_dealership sports_centre',
'restaurant bicycle_shop restaurant convenience cafe hairdresser cafe supermarket clinic fast_food beauty_shop hairdresser bicycle_shop nightclub pub school dentist school pub bakery beauty_shop restaurant sports_centre kindergarten convenience pub kindergarten convenience hairdresser beauty_shop',
'park pitch school pitch furniture_shop playground park car_wash pitch pitch pitch convenience pitch',
'park park hospital pitch park pitch playground track graveyard guesthouse hotel supermarket graveyard furniture_shop',
'car_dealership graveyard beauty_shop fast_food hairdresser hairdresser fast_food hairdresser recycling toy_shop restaurant beauty_shop hairdresser supermarket convenience restaurant toy_shop fast_food toilet fast_food community_centre bar car_dealership cafe library playground cafe park pitch pitch pitch',
'hairdresser school bank kindergarten pub car_wash',
'park graveyard veterinary pub dentist car_wash car_dealership cafe hairdresser hairdresser newsagent restaurant supermarket dentist convenience',
'pharmacy butcher dentist guesthouse kindergarten fast_food hairdresser supermarket school convenience pub hairdresser convenience',
'playground park pitch pub pub',
'school newsagent beauty_shop beauty_shop hairdresser convenience beauty_shop outdoor_shop laundry fast_food restaurant butcher hairdresser fast_food hairdresser cafe laundry beauty_shop hairdresser fast_food greengrocer hairdresser convenience bakery convenience shelter post_office restaurant fast_food fast_food hairdresser fast_food convenience supermarket graveyard pub beverages pub beauty_shop hairdresser beauty_shop bicycle_shop pub restaurant car_dealership castle car_dealership car_dealership car_dealership car_dealership',
'park library fast_food school supermarket pub convenience school school school',
'school hotel college memorial tower park pub restaurant supermarket restaurant park hotel fast_food bank hairdresser pub restaurant bakery fast_food laundry fast_food cafe hairdresser convenience pub fast_food pub cafe supermarket fast_food cafe pub pub courthouse kindergarten cafe newsagent clinic fast_food bar cafe fast_food hotel restaurant pub hotel hotel park ruins fire_station',
'park pitch pitch school pitch toilet playground park school park playground pitch community_centre park swimming_pool restaurant convenience car_rental swimming_pool museum pitch',
'pitch school',
'school park fast_food fast_food convenience police',
'pitch pitch pitch park pitch playground playground pitch pitch fast_food convenience fast_food fast_food school hairdresser hairdresser hairdresser hairdresser hairdresser cafe doityourself supermarket doityourself beauty_shop doityourself mobile_phone_shop fast_food fast_food pub fast_food hairdresser car_dealership car_dealership doityourself school',
'pitch school pitch pitch pitch pitch shelter school pitch',
'pitch stadium hairdresser fast_food convenience',
'pitch school track pitch beverages pitch fast_food pitch pitch pitch',
'market_place car_dealership cafe cafe car_dealership car_dealership cafe hotel newsagent hairdresser car_rental ruins park playground pitch stationery park graveyard pub playground pitch school cafe car_dealership doityourself doityourself bar doityourself car_rental car_dealership restaurant fast_food hairdresser fast_food',
'computer_shop beauty_shop sports_shop hairdresser convenience',
'hairdresser hairdresser doityourself guesthouse guesthouse guesthouse',
'sports_centre pitch pitch pitch cafe pitch school laundry hairdresser playground convenience butcher bicycle_shop park restaurant stationery fast_food hairdresser convenience car_wash convenience community_centre park community_centre clothes clinic computer_shop pub playground park hairdresser hairdresser cafe fast_food convenience convenience fast_food dentist convenience travel_agent convenience pub travel_agent restaurant restaurant restaurant hairdresser supermarket restaurant pitch park pitch playground hairdresser restaurant restaurant convenience pitch park convenience pub laundry post_office fast_food fast_food fast_food butcher newsagent restaurant furniture_shop school playground furniture_shop school',
'clinic pub playground playground pub restaurant school clinic dentist furniture_shop fast_food playground pub',
'car_wash fast_food sports_shop doityourself car_dealership car_dealership car_dealership fast_food beverages newsagent hairdresser mobile_phone_shop park bakery supermarket pub car_dealership doityourself doityourself car_dealership car_dealership',
'pitch school pub pub veterinary restaurant kindergarten restaurant butcher theatre beauty_shop hairdresser sports_centre restaurant florist greengrocer car_dealership furniture_shop gift_shop pub newsagent cafe shoe_shop gift_shop beauty_shop hairdresser cafe bicycle_shop furniture_shop hairdresser florist toy_shop bar fast_food fast_food bakery pub convenience restaurant optician cafe doityourself cafe fast_food fast_food restaurant cafe newsagent cafe laundry laundry bicycle_shop gift_shop hairdresser cafe college stadium prison bank kindergarten pub car_wash',
'fire_station car_rental park car_dealership school pitch pitch park kindergarten convenience fast_food playground kindergarten clinic park clinic park convenience fast_food beauty_shop florist convenience fast_food hairdresser supermarket',
'fast_food convenience hairdresser florist fast_food convenience hairdresser beverages car_dealership pub butcher pub fast_food cafe fast_food laundry',
'school market_place veterinary car_dealership restaurant computer_shop bakery shelter shelter cafe bar cafe cafe nightclub restaurant clinic cafe pub nightclub car_wash hotel car_rental doityourself doityourself sports_centre park pub nightclub pub cafe restaurant pub fast_food clothes pub cafe dentist pub hairdresser dentist pub stationery convenience fast_food bicycle_shop bakery pub hairdresser fast_food travel_agent nightclub bakery pub florist clothes theatre pub pitch park clothes',
'doctors hospital swimming_pool water_works hairdresser fast_food clothes school pitch park playground pitch track',
'park pitch park park',
'pitch school convenience fast_food pharmacy fast_food hairdresser fast_food pub pub college stadium park playground convenience',
'pub pitch school kindergarten doctors pitch park pitch supermarket playground',
'park pitch pitch public_building park park dentist',
'park',
'convenience pitch pitch school pitch pitch pitch pitch pitch nightclub fast_food supermarket pitch clinic pitch pitch pitch playground school convenience',
'hairdresser guesthouse fast_food hairdresser convenience pub guesthouse car_dealership fast_food car_dealership hairdresser fast_food supermarket beauty_shop hairdresser hairdresser fast_food restaurant hairdresser hairdresser restaurant hairdresser restaurant beauty_shop beauty_shop fast_food hairdresser convenience restaurant convenience pub hairdresser cafe sports_shop beauty_shop sports_shop pitch hairdresser laundry fast_food fast_food pitch pitch school',
'convenience bakery fast_food park playground park convenience pitch greengrocer pitch newsagent playground playground playground park artwork shelter pitch',
'nursing_home toilet university museum pitch park nursing_home pitch pitch pitch recycling_glass pitch pitch town_hall beauty_shop bicycle_shop beverages',
'kindergarten hairdresser kindergarten dentist restaurant pub pub kindergarten supermarket bar hairdresser convenience bakery hairdresser stationery beauty_shop fast_food laundry pharmacy laundry hairdresser pitch pitch pitch graveyard park library playground',
'kindergarten playground cafe pitch pitch school pub school',
'pitch pub beauty_shop cafe graveyard convenience pub kindergarten',
'hairdresser hairdresser convenience community_centre school',
'park public_building park park library convenience public_building park community_centre park hairdresser hairdresser community_centre fast_food supermarket community_centre restaurant fast_food cafe hairdresser cafe pharmacy hairdresser beverages cafe pharmacy convenience fast_food fast_food bakery fast_food pub convenience clinic supermarket park',
'hospital supermarket school playground pitch school pitch pitch park pitch',
'pitch pitch pitch pitch pitch community_centre school pitch',
'library convenience car_dealership hairdresser hairdresser fast_food cafe convenience convenience park park convenience veterinary veterinary car_dealership',
'school hairdresser park pitch kindergarten school',
'pitch playground park pitch school playground school',
'school graveyard pitch pitch pitch doctors clinic dentist convenience fast_food fast_food bakery convenience convenience convenience pharmacy convenience fast_food park fast_food cafe hairdresser hairdresser cafe convenience playground community_centre supermarket pitch school pitch',
'pitch park playground car_dealership school pub bakery pub',
'library hairdresser fast_food cafe dentist pitch pitch playground beauty_shop restaurant',
'school track community_centre pub',
'playground dentist post_office cafe cafe veterinary beverages bakery school graveyard convenience beauty_shop pub doityourself pub convenience hairdresser bank pharmacy fast_food optician butcher cafe florist hairdresser supermarket hairdresser hairdresser convenience fast_food cafe convenience convenience fast_food kindergarten hairdresser hairdresser playground park',
'mall cafe park pub playground restaurant clothes hairdresser clothes pub hairdresser restaurant gift_shop restaurant convenience restaurant restaurant museum attraction fast_food furniture_shop fast_food post_office cafe pub fast_food restaurant restaurant bakery cafe laundry park',
'pitch school pitch pitch pitch playground pitch school school school pub pub pub park library',
'pitch pitch school pitch pitch water_works park playground park pub community_centre school',
'doityourself hairdresser cafe bar beverages beauty_shop hairdresser bookshop restaurant restaurant restaurant computer_shop pharmacy cafe butcher fast_food supermarket doityourself stadium restaurant bakery fast_food bakery fast_food beauty_shop restaurant greengrocer convenience pub restaurant hairdresser clinic kindergarten florist optician cafe cafe hairdresser newsagent bank',
'toy_shop supermarket beauty_shop clothes toy_shop hairdresser supermarket cafe fast_food gift_shop pub beverages bookshop post_office gift_shop beverages bakery hairdresser greengrocer gift_shop mobile_phone_shop kindergarten bar bicycle_shop florist hairdresser beauty_shop cafe greengrocer restaurant pub cafe supermarket pub gift_shop cafe convenience pub bakery newsagent restaurant restaurant restaurant cafe library',
'playground park sports_centre park pitch park playground community_centre pub newsagent fast_food fast_food pub bar',
'clinic pitch park clinic playground pitch park park park',
'school community_centre community_centre pitch pitch pitch school kindergarten park park park park park playground playground playground playground playground park kindergarten clinic clinic',
'park golf_course school community_centre park playground park',
'sports_centre dentist ruins school guesthouse pub sports_shop pub pub convenience cafe college school clinic',
'park cafe restaurant optician pharmacy fast_food school supermarket cafe laundry park bar hairdresser graveyard hairdresser newsagent fast_food cafe shoe_shop florist furniture_shop cafe hairdresser fast_food',
'car_wash pitch doctors doctors pub florist school hospital pitch pitch water_works veterinary community_centre restaurant beauty_shop convenience dentist doityourself supermarket',
'golf_course hairdresser pitch pitch playground park pitch pitch pitch pitch pitch pitch pitch sports_centre pitch pitch pitch pitch pitch',
'park pitch pitch shelter toilet pitch pitch pitch pitch pitch pitch pitch toilet pitch pitch pitch pitch pitch pitch pitch pitch pitch pitch swimming_pool ruins swimming_pool swimming_pool swimming_pool doctors nursing_home swimming_pool swimming_pool college cafe pitch park water_tower water_works park',
'doctors restaurant florist jeweller laundry jeweller pub clinic theatre pitch pitch pitch school attraction shelter shelter pitch toilet playground attraction park shelter school',
'car_wash car_wash doityourself doctors park hairdresser beauty_shop hairdresser car_wash hairdresser butcher bank cafe cafe clothes hairdresser mobile_phone_shop greengrocer bakery cafe hairdresser cafe convenience restaurant fast_food optician newsagent clothes arts_centre pub hotel graveyard doctors clinic supermarket guesthouse dentist fast_food hairdresser beverages post_office restaurant bank pharmacy hairdresser pub fast_food toy_shop beauty_shop cafe fast_food hairdresser hairdresser pub community_centre dentist fast_food sports_centre pitch pitch pitch pub park playground school',
'park park park school sports_centre school pitch sports_centre pitch pitch school swimming_pool pitch convenience veterinary furniture_shop school pitch pitch pitch pitch pitch doctors pub',
'kindergarten bar school park fast_food fast_food fast_food fast_food supermarket fast_food convenience garden_centre supermarket supermarket bakery cafe sports_shop pharmacy cinema doityourself car_dealership doityourself fast_food park car_rental comms_tower car_dealership police doityourself playground doityourself doityourself doityourself doityourself playground park hairdresser',
'school school pub supermarket beverages fast_food car_dealership hairdresser fast_food kindergarten',
'jeweller hairdresser pitch graveyard restaurant restaurant hairdresser cafe pharmacy cafe hospital doityourself fast_food hairdresser beauty_shop pub restaurant convenience clinic hairdresser restaurant restaurant mobile_phone_shop bookshop fast_food dentist hotel cafe park bookshop pub graveyard restaurant cafe hairdresser restaurant car_dealership supermarket restaurant pub shoe_shop laundry optician florist library jeweller pub bookshop cafe jeweller beverages gift_shop clothes computer_shop travel_agent bakery florist cafe restaurant jeweller butcher restaurant pharmacy clothes cafe beauty_shop fast_food clothes pub jeweller restaurant clothes jeweller cafe bar clothes gift_shop clothes restaurant clothes clothes hotel clothes furniture_shop hairdresser pub dentist jeweller restaurant cafe beauty_shop hairdresser clothes restaurant clothes beauty_shop pub restaurant clothes clothes jeweller laundry furniture_shop pub',
'library park playground kindergarten community_centre track beauty_shop pitch school',
'convenience school pitch park toilet graveyard school pitch',
'furniture_shop pub hospital school school nursing_home nursing_home nursing_home nursing_home pitch pitch pitch pitch pitch pitch pitch playground park school park school',
'fast_food fast_food pub cafe convenience hairdresser florist school pub beauty_shop car_dealership park clothes playground convenience car_dealership pub sports_shop car_wash computer_shop fast_food clinic',
'park school',
'school convenience park hairdresser pitch',
'park pitch graveyard doctors park cafe convenience playground sports_centre pitch',
'guesthouse dentist stationery hairdresser hairdresser cafe optician hairdresser pharmacy optician cafe supermarket greengrocer hairdresser laundry bakery beauty_shop butcher gift_shop restaurant convenience garden_centre fast_food post_office hairdresser fast_food hairdresser doityourself hairdresser hairdresser bicycle_shop school beverages bar pitch cafe community_centre pitch school pitch pitch school restaurant hairdresser playground park beauty_shop pub',
'swimming_pool archaeological swimming_pool park',
'pitch track playground pitch park pub park',
'car_dealership park furniture_shop pitch school graveyard kindergarten restaurant pub beauty_shop florist cafe hairdresser pitch pitch park community_centre hairdresser convenience playground beauty_shop pub bicycle_shop laundry cafe mobile_phone_shop hairdresser cafe computer_shop hairdresser hairdresser pub convenience beauty_shop fast_food dentist supermarket fast_food',
'pub hairdresser restaurant pub dentist pub hotel restaurant park',
'furniture_shop fast_food florist hairdresser bakery fast_food convenience clothes school park pub computer_shop restaurant police community_centre park bar hairdresser hairdresser cafe restaurant kindergarten park playground restaurant mall hotel bar newsagent pub community_centre cafe pub theatre pitch doityourself arts_centre bar supermarket furniture_shop furniture_shop cafe school hotel pub department_store car_rental',
'convenience furniture_shop fast_food supermarket beverages pub bookshop restaurant beauty_shop bicycle_shop pitch school',
'playground pitch park pub beauty_shop hotel clinic pub restaurant sports_centre pub bar restaurant ruins park gift_shop bookshop beauty_shop restaurant restaurant restaurant restaurant attraction pub nightclub attraction restaurant restaurant pub restaurant cafe restaurant jeweller clothes cafe restaurant restaurant fast_food restaurant bicycle_shop clothes pub fast_food cafe bicycle_shop arts_centre bank pub restaurant nightclub bakery beverages fast_food butcher museum attraction attraction attraction hostel cafe convenience cafe pub hotel restaurant bar college pub dentist park cafe doctors dentist college restaurant pub pub pub pub hotel kindergarten pub restaurant restaurant pub pub theatre doctors restaurant pub bar hotel fast_food hotel convenience restaurant convenience fast_food convenience restaurant fast_food hairdresser restaurant hairdresser bakery hairdresser restaurant fast_food restaurant newsagent restaurant nightclub restaurant cafe florist hairdresser restaurant fast_food cafe convenience nightclub fast_food fast_food bar fast_food restaurant hairdresser restaurant fast_food hairdresser fast_food fast_food optician fast_food restaurant bar cafe restaurant pub restaurant bar bicycle_shop hairdresser hairdresser cafe pub clothes bar pub restaurant pub bar gift_shop gift_shop cafe restaurant dentist beauty_shop clinic restaurant restaurant restaurant bar restaurant mobile_phone_shop bar guesthouse cafe cafe bookshop bar bookshop jeweller mall bookshop bar cafe food_court market_place travel_agent mall hotel pub bar restaurant memorial hotel restaurant travel_agent pub fast_food restaurant park bank clothes cafe clothes clothes clothes clothes gift_shop restaurant pub fast_food clothes ruins travel_agent convenience bank toy_shop ruins pitch cafe bookshop mall mall clothes mall cafe beauty_shop mobile_phone_shop hairdresser bicycle_shop clinic restaurant clothes toy_shop fast_food beauty_shop cafe fast_food cafe supermarket fast_food nightclub jeweller cinema hairdresser mall jeweller gift_shop fast_food bakery shoe_shop gift_shop shoe_shop shoe_shop travel_agent beauty_shop mall cafe hairdresser jeweller cafe school outdoor_shop sports_centre bicycle_shop restaurant bookshop bar bar cafe hostel bar cafe arts_centre bar cafe cafe courthouse monument cafe bar pub restaurant restaurant bar restaurant cafe bar clothes hotel bakery cafe hairdresser clothes cafe hotel hairdresser restaurant pub pub pub restaurant clothes pub hairdresser shoe_shop hairdresser cafe video_shop cinema clothes beverages hairdresser bar cafe hairdresser pub fast_food travel_agent cafe pub clothes clothes shoe_shop clothes cafe clothes clothes bank restaurant optician mobile_phone_shop mall beauty_shop gift_shop pharmacy doctors fast_food mobile_phone_shop clothes mobile_phone_shop convenience community_centre cafe bakery outdoor_shop bank mobile_phone_shop cafe pharmacy clothes restaurant travel_agent shoe_shop restaurant bank fast_food mobile_phone_shop cafe mobile_phone_shop clothes mobile_phone_shop cafe bank video_shop bank clothes observation_tower observation_tower hairdresser fast_food pub fast_food fast_food hotel clothes hostel',
'doityourself doityourself supermarket beauty_shop restaurant beauty_shop computer_shop cafe restaurant optician car_dealership convenience doityourself pub',
'bicycle_shop attraction restaurant park bicycle_shop sports_centre cafe park cafe museum cafe tourist_info attraction sports_centre attraction sports_centre museum museum pub pub restaurant convenience caravan_site arts_centre arts_centre pub toilet museum attraction',
'pub school',
'park fast_food hairdresser cafe park post_office convenience convenience park park',
'hairdresser convenience hotel beauty_shop pub kindergarten',
'school park laundry hotel hairdresser pub pub doctors supermarket sports_shop hairdresser fast_food pharmacy bakery fast_food guesthouse fast_food library hotel playground park cafe cafe fast_food community_centre playground park fire_station clinic fast_food fast_food track fire_station tower shelter',
'convenience pitch pitch guesthouse pitch hairdresser restaurant playground graveyard graveyard park park',
'pharmacy car_dealership park pub clothes fast_food furniture_shop supermarket sports_shop park car_dealership supermarket clothes doityourself school',
'university clinic clinic hotel veterinary clinic pub kindergarten swimming_pool attraction doityourself clinic theatre kindergarten water_works dentist clinic supermarket cinema butcher cafe restaurant garden_centre bank mobile_phone_shop pharmacy supermarket mall clinic fast_food laundry optician gift_shop florist fast_food newsagent mobile_phone_shop cafe cafe',
'playground school',
'park picnic_site pitch pitch playground',
'arts_centre pub hairdresser hairdresser hairdresser clinic hairdresser pub clinic hotel pub food_court fast_food stationery bookshop park hairdresser convenience florist arts_centre hotel fast_food cafe pub cafe hairdresser doityourself school florist restaurant sports_shop bicycle_shop restaurant pub cafe clothes jeweller hostel cafe guesthouse hospital restaurant cafe gift_shop clothes bar beauty_shop cafe cafe beauty_shop beverages clothes travel_agent cafe fast_food nightclub outdoor_shop clothes bar bar supermarket clothes clothes restaurant restaurant bookshop stationery restaurant restaurant restaurant bar nightclub arts_centre cafe nightclub car_wash convenience theatre museum bar fast_food bar optician department_store clothes restaurant bar cafe hairdresser bar restaurant hairdresser cafe pub restaurant fast_food bar clothes hairdresser hairdresser hairdresser restaurant beauty_shop restaurant nightclub cafe hairdresser cafe bakery clothes fast_food cafe clothes bar bar dentist clothes cafe restaurant optician newsagent mobile_phone_shop bookshop cafe cafe bar pub restaurant fast_food cafe gift_shop hairdresser fast_food clothes stationery cafe bar post_office cafe clothes restaurant restaurant nightclub bank restaurant hairdresser bar stationery clothes bar cafe optician hairdresser bank supermarket mobile_phone_shop outdoor_shop clothes nightclub pharmacy hostel fast_food fast_food cafe fast_food tower restaurant museum museum sports_centre school fast_food university nightclub restaurant bank cafe bicycle_shop university beauty_shop cafe theatre university park tower wastewater_plant hospital cafe bar fast_food university arts_centre college school bank park car_rental bicycle_shop police pub hotel park hostel pub pub pub hospital pub clinic hospital hospital',
'car_dealership school pitch pitch pitch pitch pitch hairdresser supermarket doityourself',
'pitch pitch pitch pitch pitch graveyard school golf_course park',
'pitch fast_food community_centre car_dealership pitch pitch pitch pitch pitch pitch school pitch park pitch track college pitch stadium pitch pub doityourself newsagent cafe park playground pitch university university pitch park theme_park playground kindergarten pitch park garden_centre park water_works greengrocer cafe pub beauty_shop pub stationery cafe bakery computer_shop sports_centre pitch restaurant restaurant bakery fast_food school pitch school guesthouse pub convenience',
'convenience laundry school nursing_home park park clinic dentist',
'school track playground golf_course school park',
'hairdresser fast_food car_dealership park school pharmacy convenience school car_dealership car_dealership car_dealership car_dealership',
'hospital optician convenience park guesthouse pub guesthouse guesthouse outdoor_shop',
'school school school',
'school pitch pitch park pitch supermarket hairdresser hairdresser supermarket restaurant sports_centre fast_food fast_food fast_food beauty_shop veterinary dentist pub swimming_pool swimming_pool park swimming_pool swimming_pool',
'car_dealership hairdresser pub cafe hairdresser hairdresser fast_food playground car_wash car_dealership car_dealership car_dealership car_dealership school laundry bar hairdresser pub',
'park castle graveyard cafe toilet school playground pitch playground pitch playground museum pub sports_centre community_centre school pub hotel supermarket veterinary pitch school school park',
'monument playground park graveyard car_dealership clinic convenience cafe pitch butcher fast_food cafe pitch school butcher butcher supermarket beverages pitch restaurant clothes hairdresser travel_agent convenience fast_food',
'school park fast_food park',
'pub convenience hairdresser supermarket hairdresser cafe cafe hairdresser restaurant restaurant clinic butcher jeweller hairdresser fast_food clinic restaurant cafe clothes restaurant fast_food fast_food cafe beauty_shop hairdresser pub computer_shop supermarket convenience shelter hairdresser beauty_shop cafe school prison pitch pitch sports_centre pitch park hairdresser fast_food cafe sports_centre cafe supermarket doityourself restaurant restaurant mobile_phone_shop cafe hairdresser hairdresser cafe fast_food furniture_shop furniture_shop cafe hairdresser hairdresser cafe sports_shop restaurant beauty_shop beverages veterinary restaurant fast_food fast_food restaurant convenience clothes hairdresser dentist restaurant',
'park pitch pitch pitch sports_centre pitch pitch supermarket',
'hospital sports_centre pitch cinema playground restaurant hotel park track school sports_centre pitch park pitch restaurant fast_food fast_food pub',
'doctors cafe playground',
'school pub hairdresser park restaurant hairdresser hairdresser beauty_shop fast_food hairdresser',
'pitch pitch pitch sports_centre fast_food newsagent convenience community_centre hairdresser hairdresser school dentist fast_food car_wash restaurant dentist post_office doctors',
'convenience fast_food comms_tower pitch pitch pitch pitch school playground community_centre park attraction',
'golf_course fast_food supermarket fast_food doityourself fast_food newsagent doityourself dentist fast_food fast_food fast_food school playground pitch hospital supermarket',
'pub nursing_home park community_centre clinic fast_food fast_food sports_centre clinic hairdresser convenience pharmacy cafe restaurant bakery hairdresser fast_food travel_agent convenience kindergarten hairdresser cafe',
'school fast_food doityourself doityourself community_centre school pub school pitch pitch cafe playground hairdresser prison pitch pitch sports_centre pitch park',
'pitch school school pitch pitch pitch pitch playground school',
'park convenience fast_food school park park park playground school',
'college kindergarten clinic pub hairdresser furniture_shop clinic beauty_shop optician car_dealership community_centre pitch sports_centre dentist dentist beauty_shop cafe supermarket mall pub hairdresser furniture_shop clinic clothes restaurant cafe convenience pub',
'hairdresser cafe fast_food fast_food clinic',
'dentist clothes fast_food pub fast_food fast_food fast_food fast_food cafe fast_food hairdresser convenience convenience convenience car_dealership fast_food sports_shop park car_wash',
'doctors clinic pub pub clinic doityourself computer_shop beauty_shop hairdresser pub hairdresser supermarket cafe cafe computer_shop gift_shop toy_shop hairdresser convenience supermarket sports_centre bank beauty_shop greengrocer florist hairdresser restaurant jeweller supermarket bar bank cafe fast_food optician bank bakery doityourself bank hairdresser optician bank dentist pharmacy doctors shoe_shop beauty_shop newsagent pub fast_food restaurant laundry convenience clinic restaurant cafe hairdresser fast_food beauty_shop hairdresser',
'clinic doctors car_dealership pitch kindergarten museum school pitch pitch pitch school pitch pitch shelter',
'park swimming_pool college park',
'park clinic hairdresser fast_food fast_food fast_food convenience hairdresser golf_course park',
'community_centre park convenience fast_food convenience park park park convenience fast_food',
'community_centre school doctors pub school',
'newsagent fast_food convenience park park',
'park water_works convenience beauty_shop bar park hairdresser supermarket restaurant fast_food fast_food newsagent park kindergarten pub park car_dealership park',
'pharmacy arts_centre laundry convenience hairdresser beverages fast_food cafe arts_centre clothes hairdresser hairdresser newsagent cafe fast_food cafe toy_shop cafe cafe pitch park pub car_dealership restaurant school community_centre playground school guesthouse pub',
'car_dealership recycling_glass stationery playground pub playground school convenience clothes playground park park doctors pharmacy park playground',
'park playground pitch butcher hairdresser fast_food fast_food convenience pharmacy school hairdresser convenience',
'beauty_shop restaurant newsagent car_wash cafe hairdresser fast_food',
'kindergarten restaurant kindergarten hairdresser park park shelter kindergarten guesthouse hairdresser beauty_shop clothes dentist sports_shop cafe dentist restaurant veterinary pharmacy butcher travel_agent dentist bookshop hairdresser bookshop supermarket cinema restaurant school hotel',
'sports_centre school cafe university university library kindergarten university arts_centre school hotel clinic restaurant fast_food pub cafe cafe bakery pharmacy hairdresser restaurant sports_centre university university university pub doctors hairdresser hairdresser stationery pub hairdresser supermarket pub kindergarten cafe bar furniture_shop clinic sports_shop clinic pub',
'pitch doityourself doityourself school pharmacy school park beverages hairdresser fast_food fast_food hairdresser',
'clothes mobile_phone_shop convenience mobile_phone_shop convenience cafe bookshop clothes newsagent restaurant restaurant mobile_phone_shop cafe beauty_shop mobile_phone_shop clothes jeweller mobile_phone_shop travel_agent bank gift_shop sports_shop bank optician toy_shop mobile_phone_shop video_shop bank fast_food mobile_phone_shop clothes beauty_shop clothes hairdresser fast_food fast_food beauty_shop hairdresser clothes fast_food fast_food cafe shoe_shop clothes department_store outdoor_shop shoe_shop bank cafe fast_food fast_food beauty_shop furniture_shop hairdresser cafe fast_food bakery cafe gift_shop fast_food shoe_shop fast_food beauty_shop theme_park cafe shoe_shop mobile_phone_shop clothes restaurant clothes department_store clothes clothes clothes clothes clothes clothes clothes clothes hairdresser convenience mall fast_food park clothes cafe cafe fast_food bakery hotel cafe restaurant clothes clothes outdoor_shop clothes beauty_shop mobile_phone_shop beauty_shop clothes hairdresser fast_food mobile_phone_shop bicycle_shop beauty_shop hairdresser fast_food outdoor_shop hairdresser bookshop clinic cafe clothes hairdresser furniture_shop mobile_phone_shop mall park park courthouse hospital convenience fast_food fast_food playground cinema',
'restaurant cafe pub clinic supermarket hotel school college school pub school cafe pub bar restaurant restaurant hotel attraction cafe museum hairdresser bookshop pub bicycle_shop hairdresser pub fast_food cafe clothes library convenience school bank pub cafe museum cafe nightclub cafe school pitch playground park park toilet attraction university hotel jeweller clothes fast_food furniture_shop park bar restaurant hairdresser clinic restaurant fast_food fast_food travel_agent fast_food restaurant fast_food clothes hairdresser sports_centre school theatre',
'pitch pitch sports_centre park playground pub playground school graveyard doctors shelter',
'travel_agent fast_food hairdresser convenience community_centre park restaurant convenience restaurant fast_food restaurant convenience clothes convenience pharmacy cafe restaurant convenience fast_food fast_food restaurant bicycle_shop cafe restaurant hairdresser convenience bar arts_centre',
'pharmacy kindergarten clinic dentist kindergarten playground',
'playground car_dealership pitch pitch school kindergarten pitch pub community_centre playground park pitch restaurant convenience pub',
'park fast_food fast_food hairdresser park kindergarten dentist pub doctors fast_food supermarket hairdresser newsagent supermarket convenience cafe beverages optician hairdresser supermarket hairdresser fast_food bakery fast_food fast_food library community_centre pitch car_rental beauty_shop car_dealership',
'clinic pub convenience hairdresser veterinary school pitch playground',
'park ruins pitch school school school playground playground convenience',
'school playground park',
'pitch pitch stadium park swimming_pool stadium school pitch pharmacy doctors pitch pitch beauty_shop hairdresser kindergarten park golf_course park',
'library fast_food bakery post_office pharmacy supermarket supermarket',
'park',
'convenience dentist clothes pub cafe greengrocer clothes shoe_shop hairdresser greengrocer cafe bookshop restaurant cafe gift_shop cafe furniture_shop beverages jeweller jeweller cafe jeweller clothes mall florist bar gift_shop restaurant hairdresser beauty_shop pitch pitch clinic pitch pitch kindergarten clinic swimming_pool school school',
'school community_centre water_works car_wash supermarket golf_course pitch pitch restaurant beauty_shop park nursing_home restaurant pub nursing_home nursing_home',
'convenience car_wash doctors community_centre hairdresser clinic restaurant hairdresser fast_food bakery fast_food optician bank travel_agent convenience pub fast_food beauty_shop stationery pharmacy fast_food cafe cafe pub chemist bank fast_food convenience hairdresser pub restaurant restaurant restaurant park dentist hairdresser fast_food newsagent fast_food fast_food laundry veterinary hairdresser kindergarten butcher playground park bar optician doctors school park park pitch park park',
'park school pitch school convenience school school clinic veterinary florist fast_food convenience hairdresser fast_food pharmacy hairdresser',
'sports_centre sports_centre recycling_metal park community_centre convenience stationery supermarket furniture_shop community_centre newsagent school pub school playground kindergarten pub park playground park',
'pub bar restaurant restaurant restaurant restaurant pub hairdresser supermarket restaurant fast_food fast_food bar beauty_shop hairdresser restaurant doityourself restaurant hairdresser bar pharmacy pub hairdresser bank fast_food clinic beverages cafe bakery restaurant fast_food fast_food beauty_shop cafe doityourself cafe hairdresser fast_food bar beauty_shop beauty_shop doctors school pub fast_food cafe nursing_home clothes hairdresser bookshop beverages sports_centre optician cafe restaurant clinic laundry clothes hairdresser cafe cafe supermarket gift_shop clothes convenience restaurant restaurant restaurant bar pub restaurant restaurant hairdresser beauty_shop cafe hairdresser greengrocer beverages',
'ruins ruins ruins ruins archaeological ruins ruins playground community_centre park attraction playground playground park community_centre pitch cafe convenience pharmacy fast_food community_centre hairdresser fast_food convenience convenience archaeological pitch playground',
'park pub convenience',
'pitch pitch fast_food convenience community_centre pitch kindergarten']
Then, we can use the fit_transform()
function provided by TfidfVectorizer
to get the tfidf
scores:
tfidf_response = tfidf.fit_transform(docList)
We can also convert the result of a specific document into a dataframe
as well:
tfidf_pd = pd.DataFrame(tfidf_response[0].T.todense(), index=tfidf.get_feature_names(), columns=["TF-IDF"])
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
Cell In[37], line 1
----> 1 tfidf_pd = pd.DataFrame(tfidf_response[0].T.todense(), index=tfidf.get_feature_names(), columns=["TF-IDF"])
AttributeError: 'TfidfVectorizer' object has no attribute 'get_feature_names'
tfidf_pd
TF-IDF | |
---|---|
archaeological | 0.0 |
arts_centre | 0.0 |
artwork | 0.0 |
attraction | 0.0 |
bakery | 0.0 |
... | ... |
veterinary | 0.0 |
video_shop | 0.0 |
wastewater_plant | 0.0 |
water_tower | 0.0 |
water_works | 0.0 |
105 rows × 1 columns
As can be seen here, since the list of words is long, many of the rows are not showing here. A trick to show the full table with all rows being listed is to use the code below:
pd.set_option('display.max_rows', None)
tfidf_pd
TF-IDF | |
---|---|
archaeological | 0.000000 |
arts_centre | 0.000000 |
artwork | 0.000000 |
attraction | 0.000000 |
bakery | 0.000000 |
bank | 0.000000 |
bar | 0.000000 |
beauty_shop | 0.000000 |
beverages | 0.000000 |
bicycle_shop | 0.000000 |
bookshop | 0.000000 |
butcher | 0.000000 |
cafe | 0.000000 |
camp_site | 0.000000 |
car_dealership | 0.000000 |
car_rental | 0.000000 |
car_wash | 0.000000 |
caravan_site | 0.000000 |
castle | 0.000000 |
chemist | 0.000000 |
cinema | 0.000000 |
clinic | 0.000000 |
clothes | 0.000000 |
college | 0.000000 |
comms_tower | 0.000000 |
community_centre | 0.000000 |
computer_shop | 0.000000 |
convenience | 0.000000 |
courthouse | 0.000000 |
dentist | 0.000000 |
department_store | 0.000000 |
doctors | 0.000000 |
doityourself | 0.000000 |
fast_food | 0.000000 |
fire_station | 0.000000 |
florist | 0.000000 |
food_court | 0.000000 |
fountain | 0.000000 |
furniture_shop | 0.000000 |
garden_centre | 0.000000 |
gift_shop | 0.000000 |
golf_course | 0.000000 |
graveyard | 0.000000 |
greengrocer | 0.000000 |
guesthouse | 0.000000 |
hairdresser | 0.000000 |
hospital | 0.000000 |
hostel | 0.000000 |
hotel | 0.000000 |
jeweller | 0.000000 |
kindergarten | 0.000000 |
laundry | 0.000000 |
library | 0.000000 |
mall | 0.000000 |
market_place | 0.000000 |
memorial | 0.000000 |
mobile_phone_shop | 0.000000 |
monument | 0.000000 |
museum | 0.000000 |
nan | 0.000000 |
newsagent | 0.000000 |
nightclub | 0.000000 |
nursing_home | 0.000000 |
observation_tower | 0.000000 |
optician | 0.000000 |
outdoor_shop | 0.000000 |
park | 0.194603 |
pharmacy | 0.000000 |
picnic_site | 0.000000 |
pitch | 0.000000 |
playground | 0.249640 |
police | 0.000000 |
post_office | 0.000000 |
prison | 0.000000 |
pub | 0.000000 |
public_building | 0.000000 |
recycling | 0.000000 |
recycling_glass | 0.000000 |
recycling_metal | 0.000000 |
restaurant | 0.000000 |
ruins | 0.000000 |
school | 0.000000 |
shelter | 0.000000 |
shoe_shop | 0.000000 |
sports_centre | 0.000000 |
sports_shop | 0.000000 |
stadium | 0.000000 |
stationery | 0.000000 |
supermarket | 0.000000 |
swimming_pool | 0.948583 |
theatre | 0.000000 |
theme_park | 0.000000 |
toilet | 0.000000 |
tourist_info | 0.000000 |
tower | 0.000000 |
town_hall | 0.000000 |
toy_shop | 0.000000 |
track | 0.000000 |
travel_agent | 0.000000 |
university | 0.000000 |
veterinary | 0.000000 |
video_shop | 0.000000 |
wastewater_plant | 0.000000 |
water_tower | 0.000000 |
water_works | 0.000000 |
We can also order the tfidf_pd
and only show the top 25 (or other numbers as you wish) records. As we have learnt from the lecture, the greater the TF-IDF, the more relevant/unique the word is to characterize the document.
tfidf_pd = tfidf_pd.sort_values('TF-IDF', ascending=False)
tfidf_pd.head(25)
TF-IDF | |
---|---|
swimming_pool | 0.948583 |
playground | 0.249640 |
park | 0.194603 |
recycling_metal | 0.000000 |
recycling | 0.000000 |
public_building | 0.000000 |
pub | 0.000000 |
prison | 0.000000 |
post_office | 0.000000 |
police | 0.000000 |
pitch | 0.000000 |
picnic_site | 0.000000 |
pharmacy | 0.000000 |
archaeological | 0.000000 |
outdoor_shop | 0.000000 |
optician | 0.000000 |
observation_tower | 0.000000 |
nursing_home | 0.000000 |
nightclub | 0.000000 |
newsagent | 0.000000 |
nan | 0.000000 |
museum | 0.000000 |
monument | 0.000000 |
mobile_phone_shop | 0.000000 |
memorial | 0.000000 |
Part 6: Relevance Ranking#
With TF-IDF for each word in the document, we can then compute the relevance of a query in respect to the candidate documents. A real-world scenaria can be like this: a user might want to find similar regions that have POIs with place types including “park” and “car dealership” (this is the query). What we can do is to compare the vector of query with the vector of each document, and rank their relevances. The top documents then can be selected to responde to users’ query. The vector here is exactly the TF-IDF vector we have just computed (like the tfidf_pd
).
For the query, we regard it as a single document. Its TF-IDF vector is computed using the same way as what we have done (namely, we can directly multiple the IDF (same as before) with the TF of this specific query document). Below are the codes.
First, we get the term frequency dictionary of the query document:
## rank the relevance score of a query to the documents (region)
query = ["park", "car_dealership"]
# get the vector of the query (same to what we have done before)
query_wordDict = dict.fromkeys(wordSet, 0)
for word in query:
query_wordDict[word]+=1
query_wordDict
{'clothes': 0,
'butcher': 0,
'dentist': 0,
'recycling': 0,
'sports_shop': 0,
'furniture_shop': 0,
'bicycle_shop': 0,
'recycling_glass': 0,
'hospital': 0,
'track': 0,
'chemist': 0,
'garden_centre': 0,
'veterinary': 0,
'beauty_shop': 0,
'car_wash': 0,
'supermarket': 0,
'tower': 0,
'outdoor_shop': 0,
'playground': 0,
'college': 0,
'fountain': 0,
'car_rental': 0,
'park': 1,
'wastewater_plant': 0,
'swimming_pool': 0,
'beverages': 0,
'hostel': 0,
'nursing_home': 0,
'mobile_phone_shop': 0,
'memorial': 0,
'nan': 0,
'newsagent': 0,
'artwork': 0,
'jeweller': 0,
'cafe': 0,
'water_works': 0,
'ruins': 0,
'arts_centre': 0,
'market_place': 0,
'university': 0,
'kindergarten': 0,
'monument': 0,
'convenience': 0,
'community_centre': 0,
'guesthouse': 0,
'mall': 0,
'camp_site': 0,
'greengrocer': 0,
'bank': 0,
'graveyard': 0,
'shoe_shop': 0,
'car_dealership': 1,
'gift_shop': 0,
'stadium': 0,
'hotel': 0,
'bakery': 0,
'restaurant': 0,
'shelter': 0,
'hairdresser': 0,
'post_office': 0,
'public_building': 0,
'department_store': 0,
'comms_tower': 0,
'fire_station': 0,
'stationery': 0,
'library': 0,
'theme_park': 0,
'castle': 0,
'water_tower': 0,
'picnic_site': 0,
'tourist_info': 0,
'golf_course': 0,
'school': 0,
'cinema': 0,
'optician': 0,
'prison': 0,
'florist': 0,
'food_court': 0,
'travel_agent': 0,
'attraction': 0,
'pub': 0,
'fast_food': 0,
'museum': 0,
'video_shop': 0,
'archaeological': 0,
'observation_tower': 0,
'pitch': 0,
'recycling_metal': 0,
'clinic': 0,
'bar': 0,
'computer_shop': 0,
'bookshop': 0,
'nightclub': 0,
'police': 0,
'pharmacy': 0,
'caravan_site': 0,
'doityourself': 0,
'toilet': 0,
'sports_centre': 0,
'laundry': 0,
'courthouse': 0,
'town_hall': 0,
'toy_shop': 0,
'theatre': 0,
'doctors': 0}
query_tf = computeTF(query_wordDict)
query_tf
{'clothes': 0.0,
'butcher': 0.0,
'dentist': 0.0,
'recycling': 0.0,
'sports_shop': 0.0,
'furniture_shop': 0.0,
'bicycle_shop': 0.0,
'recycling_glass': 0.0,
'hospital': 0.0,
'track': 0.0,
'chemist': 0.0,
'garden_centre': 0.0,
'veterinary': 0.0,
'beauty_shop': 0.0,
'car_wash': 0.0,
'supermarket': 0.0,
'tower': 0.0,
'outdoor_shop': 0.0,
'playground': 0.0,
'college': 0.0,
'fountain': 0.0,
'car_rental': 0.0,
'park': 0.5,
'wastewater_plant': 0.0,
'swimming_pool': 0.0,
'beverages': 0.0,
'hostel': 0.0,
'nursing_home': 0.0,
'mobile_phone_shop': 0.0,
'memorial': 0.0,
'nan': 0.0,
'newsagent': 0.0,
'artwork': 0.0,
'jeweller': 0.0,
'cafe': 0.0,
'water_works': 0.0,
'ruins': 0.0,
'arts_centre': 0.0,
'market_place': 0.0,
'university': 0.0,
'kindergarten': 0.0,
'monument': 0.0,
'convenience': 0.0,
'community_centre': 0.0,
'guesthouse': 0.0,
'mall': 0.0,
'camp_site': 0.0,
'greengrocer': 0.0,
'bank': 0.0,
'graveyard': 0.0,
'shoe_shop': 0.0,
'car_dealership': 0.5,
'gift_shop': 0.0,
'stadium': 0.0,
'hotel': 0.0,
'bakery': 0.0,
'restaurant': 0.0,
'shelter': 0.0,
'hairdresser': 0.0,
'post_office': 0.0,
'public_building': 0.0,
'department_store': 0.0,
'comms_tower': 0.0,
'fire_station': 0.0,
'stationery': 0.0,
'library': 0.0,
'theme_park': 0.0,
'castle': 0.0,
'water_tower': 0.0,
'picnic_site': 0.0,
'tourist_info': 0.0,
'golf_course': 0.0,
'school': 0.0,
'cinema': 0.0,
'optician': 0.0,
'prison': 0.0,
'florist': 0.0,
'food_court': 0.0,
'travel_agent': 0.0,
'attraction': 0.0,
'pub': 0.0,
'fast_food': 0.0,
'museum': 0.0,
'video_shop': 0.0,
'archaeological': 0.0,
'observation_tower': 0.0,
'pitch': 0.0,
'recycling_metal': 0.0,
'clinic': 0.0,
'bar': 0.0,
'computer_shop': 0.0,
'bookshop': 0.0,
'nightclub': 0.0,
'police': 0.0,
'pharmacy': 0.0,
'caravan_site': 0.0,
'doityourself': 0.0,
'toilet': 0.0,
'sports_centre': 0.0,
'laundry': 0.0,
'courthouse': 0.0,
'town_hall': 0.0,
'toy_shop': 0.0,
'theatre': 0.0,
'doctors': 0.0}
Then, we get the TF-IDF using the function computeTFIDF()
:
# we often use the same idfs as the documents
query_idf = idfs
query_tfidf = computeTFIDF(query_tf, query_idf)
query_tfidf
{'clothes': 0.0,
'butcher': 0.0,
'dentist': 0.0,
'recycling': 0.0,
'sports_shop': 0.0,
'furniture_shop': 0.0,
'bicycle_shop': 0.0,
'recycling_glass': 0.0,
'hospital': 0.0,
'track': 0.0,
'chemist': 0.0,
'garden_centre': 0.0,
'veterinary': 0.0,
'beauty_shop': 0.0,
'car_wash': 0.0,
'supermarket': 0.0,
'tower': 0.0,
'outdoor_shop': 0.0,
'playground': 0.0,
'college': 0.0,
'fountain': 0.0,
'car_rental': 0.0,
'park': 0.08599124106397561,
'wastewater_plant': 0.0,
'swimming_pool': 0.0,
'beverages': 0.0,
'hostel': 0.0,
'nursing_home': 0.0,
'mobile_phone_shop': 0.0,
'memorial': 0.0,
'nan': 0.0,
'newsagent': 0.0,
'artwork': 0.0,
'jeweller': 0.0,
'cafe': 0.0,
'water_works': 0.0,
'ruins': 0.0,
'arts_centre': 0.0,
'market_place': 0.0,
'university': 0.0,
'kindergarten': 0.0,
'monument': 0.0,
'convenience': 0.0,
'community_centre': 0.0,
'guesthouse': 0.0,
'mall': 0.0,
'camp_site': 0.0,
'greengrocer': 0.0,
'bank': 0.0,
'graveyard': 0.0,
'shoe_shop': 0.0,
'car_dealership': 0.32455186842380684,
'gift_shop': 0.0,
'stadium': 0.0,
'hotel': 0.0,
'bakery': 0.0,
'restaurant': 0.0,
'shelter': 0.0,
'hairdresser': 0.0,
'post_office': 0.0,
'public_building': 0.0,
'department_store': 0.0,
'comms_tower': 0.0,
'fire_station': 0.0,
'stationery': 0.0,
'library': 0.0,
'theme_park': 0.0,
'castle': 0.0,
'water_tower': 0.0,
'picnic_site': 0.0,
'tourist_info': 0.0,
'golf_course': 0.0,
'school': 0.0,
'cinema': 0.0,
'optician': 0.0,
'prison': 0.0,
'florist': 0.0,
'food_court': 0.0,
'travel_agent': 0.0,
'attraction': 0.0,
'pub': 0.0,
'fast_food': 0.0,
'museum': 0.0,
'video_shop': 0.0,
'archaeological': 0.0,
'observation_tower': 0.0,
'pitch': 0.0,
'recycling_metal': 0.0,
'clinic': 0.0,
'bar': 0.0,
'computer_shop': 0.0,
'bookshop': 0.0,
'nightclub': 0.0,
'police': 0.0,
'pharmacy': 0.0,
'caravan_site': 0.0,
'doityourself': 0.0,
'toilet': 0.0,
'sports_centre': 0.0,
'laundry': 0.0,
'courthouse': 0.0,
'town_hall': 0.0,
'toy_shop': 0.0,
'theatre': 0.0,
'doctors': 0.0}
The dictionary can also be converted to a dataframe
:
queryTFIDFMatrix = pd.DataFrame.from_dict(query_tfidf, orient='index').T
queryTFIDFMatrix
clothes | butcher | dentist | recycling | sports_shop | furniture_shop | bicycle_shop | recycling_glass | hospital | track | ... | caravan_site | doityourself | toilet | sports_centre | laundry | courthouse | town_hall | toy_shop | theatre | doctors | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 |
1 rows × 105 columns
Finally, we can write a function to compute the similarity between the TF-IDF vectors of candicate documents (doc_tfidf
) with the TF-IDF vector of the query document (query_tfidf
), and rank it using codes like:
import numpy as np
from numpy.linalg import norm
def rankingTFIDF(query_tfidf, doc_tfidf):
doc_cosine = []
for index, row in doc_tfidf.iterrows():
doc_tfidf_vec = row.to_numpy().flatten()[1:]
query_tfidf_vec = query_tfidf.to_numpy().flatten()
cosine = np.dot(doc_tfidf_vec,query_tfidf_vec)/norm(doc_tfidf_vec)*norm(query_tfidf_vec)
doc_cosine.append([row[0], cosine])
doc_cosine_df = pd.DataFrame(doc_cosine, columns=["LSOA11 Code", "Cosine Similarity"])
doc_cosine_df = doc_cosine_df.sort_values(by=['Cosine Similarity'],ascending=False)
return doc_cosine_df
To apply the function and only show the top 10 results:
doc_cosine_df_test = rankingTFIDF(queryTFIDFMatrix, doc2wordTFIDFMatrix)
doc_cosine_df_test.iloc[0:9]
LSOA11 Code | Cosine Similarity | |
---|---|---|
203 | E01014537 | 0.106043 |
22 | E01014569 | 0.090785 |
207 | E01014565 | 0.090718 |
51 | E01014679 | 0.086994 |
121 | E01014506 | 0.082671 |
72 | E01014675 | 0.077745 |
147 | E01014682 | 0.060071 |
116 | E01033352 | 0.058472 |
47 | E01014617 | 0.058310 |
To evaluate the results, we check the TF-IDF vector of the document who is ranked the top. We can find that this document (region) contains pois with types of “car dealership” and “park” as well (as what our query requests):
pd.set_option('display.max_columns', None)
doc2wordTFIDFMatrix.loc[doc2wordTFIDFMatrix['LSOA11 Code'] == "E01014537"]
LSOA11 Code | clothes | butcher | dentist | recycling | sports_shop | furniture_shop | bicycle_shop | recycling_glass | hospital | track | chemist | garden_centre | veterinary | beauty_shop | car_wash | supermarket | tower | outdoor_shop | playground | college | fountain | car_rental | park | wastewater_plant | swimming_pool | beverages | hostel | nursing_home | mobile_phone_shop | memorial | nan | newsagent | artwork | jeweller | cafe | water_works | ruins | arts_centre | market_place | university | kindergarten | monument | convenience | community_centre | guesthouse | mall | camp_site | greengrocer | bank | graveyard | shoe_shop | car_dealership | gift_shop | stadium | hotel | bakery | restaurant | shelter | hairdresser | post_office | public_building | department_store | comms_tower | fire_station | stationery | library | theme_park | castle | water_tower | picnic_site | tourist_info | golf_course | school | cinema | optician | prison | florist | food_court | travel_agent | attraction | pub | fast_food | museum | video_shop | archaeological | observation_tower | pitch | recycling_metal | clinic | bar | computer_shop | bookshop | nightclub | police | pharmacy | caravan_site | doityourself | toilet | sports_centre | laundry | courthouse | town_hall | toy_shop | theatre | doctors | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
203 | E01014537 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.171982 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.258588 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 3.245519 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.286417 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.399695 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.26462 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.703952 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 |
Great that you have followed the instructions till here. Note that it is just an example to show you how some introduced techniques in the lecture can be implemented in Python. Hope it is interesting and have inspired you on your own projects. Also note that the dataset we are using - place types of POIs - is relatively clean. In most geo-text analysis, you might need to use a lot natural language preprocessing such as stemming, lemmatisation, punctuation, etc. Don’t forget that spaCy
can help you on it (Lab 2).