Lab 04: Knowledge Graphs and Neural Network Embedding for Places#
Part 0: Retrieving from a Knowledge Graph (optional)#
First of all, let’s experiment on playing with ontology and knowledge graph (speficically RDF graph here). As we learnt from the lecture, RDF graph is the fundemental graph structure to organize data. It is in the format of <subject, predicate, object>, each of which can be referred to a URI (Uniform Resource Identifier) on the Web. In this practical, we play with GeoNames, which we have discussed when talking about gazetteers. It is so far the largest world-scale gazetteer. Not suprisingly, the data is stored as a graph databse. Its endpoint can be found at: https://www.geonames.org/v3/. Note that all the information rendered in these kinds of web pages are fundementally stored as a graph in the back end.
To experiment it, we use the following library. Make sure you install it.
Our task here is to get all triples (statements) related to the node of “City of Bristol” (it is represented as “https://sws.geonames.org/3333134/” in the GeoNames graph.) To do so, we first need to create a graph using Graph()
.
from rdflib import Graph
# Create a Wien Graph
g = Graph()
Next, we pass in the target node of “City of Bristol” using the function parse()
to the graph. It is represented as a web source identified using a URI:
g.parse("https://sws.geonames.org/3333134/")
<Graph identifier=N264e3878683c4bd3b3b5783a513f2124 (<class 'rdflib.graph.Graph'>)>
Now we have a graph called g
, which is comprised of triples that related to “City of Bristol”. We can check the numbber of triples in such a graph:
print(f"The City of Bristol graph has {len(g)} triples.")
The City of Bristol graph has 65 triples.
To show all the triples in the graph, we can use the function called serialization()
and pass the format of turtle
to it. turtle
here is a serialization format for RDF graphs defined by OGC. It is like the csv
file for relational databases.
print(g.serialize(format="turtle"))
@prefix cc: <http://creativecommons.org/ns#> .
@prefix dcterms: <http://purl.org/dc/terms/> .
@prefix foaf: <http://xmlns.com/foaf/0.1/> .
@prefix gn: <http://www.geonames.org/ontology#> .
@prefix owl: <http://www.w3.org/2002/07/owl#> .
@prefix rdfs: <http://www.w3.org/2000/01/rdf-schema#> .
@prefix wgs84_pos: <http://www.w3.org/2003/01/geo/wgs84_pos#> .
<https://sws.geonames.org/3333134/> a gn:Feature ;
gn:alternateName "Bristol",
"Bristón"@an,
"Bricgstōw"@ang,
"بريستول"@ar,
"Горад Брысталь"@be,
"Бристъл"@bg,
"Bryste"@cy,
"Μπρίστολ"@el,
"Bristolo"@eo,
"Brístol"@es,
"بریستول"@fa,
"Briostó"@ga,
"בריסטול"@he,
"ब्रिस्टल"@hi,
"Բրիստոլ"@hy,
"ブリストル"@ja,
"ბრისტოლი"@ka,
"ಬ್ರಿಸ್ಟಲ್"@kn,
"브리스틀"@ko,
"Bristolium"@la,
"Bristolis"@lt,
"Bristole"@lv,
"Бристол"@mk,
"ब्रिस्टल"@mr,
"بریستول"@mzn,
"Bristol"@nb,
"Bristol"@nn,
"Bristo"@nrm,
"Бристоль"@os,
"ਬਰਿਸਟਲ"@pa,
"برسٹل نگر"@pnb,
"Бристоль"@ru,
"Bristullu"@scn,
"Бристол"@sr,
"பிரிஸ்டல்"@ta,
"บริสตอล"@th,
"Бристоль"@uk,
"בריסטאל"@yi,
"布里斯托尔"@zh ;
gn:childrenFeatures <https://sws.geonames.org/3333134/contains.rdf> ;
gn:countryCode "GB" ;
gn:featureClass <https://www.geonames.org/ontology#A> ;
gn:featureCode <https://www.geonames.org/ontology#A.ADM2> ;
gn:locationMap <https://www.geonames.org/3333134/city-of-bristol.html> ;
gn:name "Bristol" ;
gn:officialName "City of Bristol",
"Bristol"@en ;
gn:parentADM1 <https://sws.geonames.org/6269131/> ;
gn:parentCountry <https://sws.geonames.org/2635167/> ;
gn:parentFeature <https://sws.geonames.org/11609016/> ;
gn:population "454213" ;
gn:wikipediaArticle <https://en.wikipedia.org/wiki/Bristol> ;
rdfs:isDefinedBy <https://sws.geonames.org/3333134/about.rdf> ;
rdfs:seeAlso <https://dbpedia.org/resource/Bristol> ;
owl:sameAs <http://www.ordnancesurvey.co.uk/ontology/v1/AdministrativeGeography.rdf#city_of_bristol_00hb> ;
wgs84_pos:lat "51.45" ;
wgs84_pos:long "-2.6" .
<https://sws.geonames.org/3333134/about.rdf> a foaf:Document ;
cc:attributionName "GeoNames"^^<https://www.w3.org/2001/XMLSchema#string> ;
cc:attributionURL <https://www.geonames.org> ;
cc:license <https://creativecommons.org/licenses/by/4.0/> ;
dcterms:created "2006-01-15"^^<https://www.w3.org/2001/XMLSchema#date> ;
dcterms:modified "2019-03-22"^^<https://www.w3.org/2001/XMLSchema#date> ;
foaf:primaryTopic <https://sws.geonames.org/3333134/> .
From the graph, we can see triples (statements) that can be read by machine and humans, such as: “Bristol is a Feature; it has alternative names including Bristol, Briston, and so on; it has a country code of ‘GB’; it has a population of 454,213; and so on” (note that the city of Bristol is represented as ‘https://sws.geonames.org/3333134/>’ in this graph).
The beauty of knowlege graph is that everything connected to everything else. You can just use the link (or called predicate) here to keep searching. For instance, if we wanted to retrieve all related information about the Country (represented as a predicate - parentCountry
in the graph) of the City of Bristol
:
from rdflib import URIRef
bristol = URIRef("https://sws.geonames.org/3333134/") # this is the URI of the Bristol node
predicate_chosen = URIRef("http://www.geonames.org/ontology#parentCountry") #this is the predicate URI of "parent country"
#The code below retrieves all the triples who are of the form <bristol, predicate_chosen, ???>. In this specific case, we only find only one such ???
for s, p, o in g.triples((bristol, predicate_chosen, None)):
print(s, p, o)
https://sws.geonames.org/3333134/ http://www.geonames.org/ontology#parentCountry https://sws.geonames.org/2635167/
From the result, we retrieve the ??? (an object o) as https://sws.geonames.org/2635167/
. In the next two steps, we can further query the graph whose subject node is o:
g = Graph() # we can initialize the graph again. Otherwise, the newly retrieved data will be appended to the old graph
g.parse(o)
print(g.serialize(format="turtle"))
@prefix cc: <http://creativecommons.org/ns#> .
@prefix dcterms: <http://purl.org/dc/terms/> .
@prefix foaf: <http://xmlns.com/foaf/0.1/> .
@prefix gn: <http://www.geonames.org/ontology#> .
@prefix rdfs: <http://www.w3.org/2000/01/rdf-schema#> .
@prefix wgs84_pos: <http://www.w3.org/2003/01/geo/wgs84_pos#> .
<https://sws.geonames.org/2635167/> a gn:Feature ;
gn:alternateName "Britain",
"VK"@af,
"ዩናይትድ ኪንግደም"@am,
"Reino Unito"@an,
"Geāned Cynerīce"@ang,
"ܡܠܟܘܬܐ ܡܚܝܕܬܐ"@arc,
"Reinu Uníu"@ast,
"Reinu Xuníu"@ast,
"BK"@az,
"Vaeinigts Kinireich"@bar,
"Велікабрытанія"@be,
"Обединено кралство"@bg,
"Обединено кралство Великобритания и Северна Ирландия"@bg,
"যুক্তরাজ্য"@bn,
"Rouantelezh Unanet Breizh-Veur ha Norzhiwerzhon"@br,
"Ujedinjeno Kraljevstvo"@bs,
"RU"@ca,
"Ĭng-guók"@cdo,
"شانشینی یەکگرتوو"@ckb,
"Regnu Unitu"@co,
"Velká Británie"@cs,
"DU"@cy,
"Det Forenede Kongerige"@da,
"Det Forenede Kongerige Storbritannien og Nordirland"@da,
"Großbritannien und Nordirland"@de,
"Vereinigte Königreich Großbritannien und Nordirland"@de,
"Britain"@en,
"U.K"@en,
"U.K."@en,
"United Kingdom of Great Britain and Northern Ireland"@en,
"Unuiĝinta Reĝlando de Granda Britio kaj Nord-Irlando"@eo,
"Suurbritannia"@et,
"EB"@eu,
"پادشاهی متحد بریتانیای کبیر و ایرلند شمالی"@fa,
"پادشاهی متحده"@fa,
"Yhdistynyt kuningaskunta"@fi,
"Stóra Bretland"@fo,
"UK"@fo,
"R.-u."@fr,
"Royômo-Uni"@frp,
"Grut-Brittanje"@fy,
"An Rìoghachd Aonaichte"@gd,
"RU"@gl,
"Reino Unido - United Kingdom"@gl,
"Reeriaght Unnaneysit"@gv,
"Ujedinjeno Kraljevstvo"@hbs,
"הממלכה המאוחדת"@he,
"संयुक्त राजशाही"@hi,
"Ujedinjena Kraljevina Velike Britanije i Sjeverne Irske"@hr,
"Ujedinjeno Kraljevstvo"@hr,
"Zjednoćene kralestwo"@hsb,
"Wayòm Ini"@ht,
"Մեծ Բրիտանիա"@hy,
"Միավորված Թագավորություն"@hy,
"Britania Raya"@id,
"Inggris Raya"@id,
"Nagkaykaysa a Pagarian"@ilo,
"Unionita Rejio"@io,
"UK"@it,
"გაერთიანებული სამეფო"@ka,
"დიდი ბრიტანეთი"@ka,
"Keyaniya Yekbûyî"@ku,
"Keyatiya Yekbûyî ya Brîtaniya Mezin û Îrlanda"@ku,
"Britanniarum Regnum"@la,
"Regnum Unitum"@la,
"Groussbritannien an Nordirland"@lb,
"Vereineg Keuninkriek"@li,
"Regno Unïo"@lij,
"JK"@lt,
"Jungtinė Didžiosios Britanijos ir Šiaurės Airijos Karalystė"@lt,
"Lielbritānija"@lv,
"Kīngitanga Kotahi"@mi,
"Обединето Кралство"@mk,
"Renju Unit"@mt,
"Vereenigt Königriek vun Grootbritannien un Noordirland"@nds,
"Groot-Brittannië"@nl,
"Storbritannia"@nn,
"Det forente kongerike Storbritannia og Nord-Irland"@no,
"Rouoyaume Unni"@nrm,
"Reialme Unit"@oc,
"Yhtys Kuningaskundu"@olo,
"Pisanmetung a Ka-arian"@pam,
"Regn Unì"@pms,
"بريتانيا"@ps,
"Hukllachasqa Qhapaq Suyu"@qu,
"Reginavel Unì da la Gronda Britannia ed Irlanda dal Nord"@rm,
"Phandlo Thagaripen la Bare Britaniyako thai le Nordutne Irlandesko"@rmy,
"Regatul Unit al Marii Britanii și al Irlandei de Nord"@ro,
"Соединенное Королевство"@ru,
"Regnu Unitu"@scn,
"Unitit Kinrick"@sco,
"Velika Britanija"@sl,
"Združeno kraljestvo Velike Britanije in Severne Irske"@sl,
"Mbretëria e Bashkuar e Britanisë së Madhe dhe Irlandës së Veriut"@sq,
"Уједињено Краљевство"@sr,
"ஐக்கிய இராச்சியம்"@ta,
"Reinu Naklibur"@tet,
"Подшоҳии Муттаҳида"@tg,
"Nagkakaisang Kaharain"@tl,
"Nagkakaisang Kaharian"@tl,
"Birleşik Krallık"@tr,
"Paratāne"@ty,
"بۈيۈك بېرىتانىيە"@ug,
"Великобританія"@uk,
"برطانیہ"@ur,
"Vương quốc Liên hiệp Anh và Bắc Ireland"@vi,
"פאראייניגטע קעניגרייך"@yi,
"英國"@zh ;
gn:childrenFeatures <https://sws.geonames.org/2635167/contains.rdf> ;
gn:countryCode "GB" ;
gn:featureClass <https://www.geonames.org/ontology#A> ;
gn:featureCode <https://www.geonames.org/ontology#A.PCLI> ;
gn:locationMap <https://www.geonames.org/2635167/united-kingdom-of-great-britain-and-northern-ireland.html> ;
gn:name "United Kingdom" ;
gn:neighbouringFeatures <https://sws.geonames.org/2635167/neighbours.rdf> ;
gn:officialName "United Kingdom"@aa,
"Verenigde Koninkryk"@af,
"Ahendiman Nkabom"@ak,
"ዩኬ"@am,
"المملكة المتحدة"@ar,
"ইউ. কে."@as,
"Böyük Britaniya"@az,
"Вялікабрытанія"@be,
"Вялікая Брытанія"@be,
"Великобритания"@bg,
"Angilɛtɛri"@bm,
"ইউ কে"@bn,
"དབྱིན་ཇི་"@bo,
"UK"@bs,
"Regne Unit de la Gran Bretanya i Irlanda del Nord"@ca,
"Йоккха Британи"@ce,
"Spojené království"@cs,
"Y Deyrnas Unedig"@cy,
"Storbritannien"@da,
"Vereinigtes Königreich"@de,
"ཡུ་ནཱའི་ཊེཌ་ ཀིང་ཌམ"@dz,
"United Kingdom nutome"@ee,
"ΗΒ"@el,
"United Kingdom"@en,
"Unuiĝinta Reĝlando"@eo,
"Reino Unido"@es,
"ÜK"@et,
"Erresuma Batua"@eu,
"انگلستان"@fa,
"بریتانیا"@fa,
"Laamateeri Rentundi"@ff,
"Britannia"@fi,
"Royaume-Uni"@fr,
"VK"@fy,
"RA"@ga,
"RA"@gd,
"યુ.કે."@gu,
"Rywvaneth Unys"@gv,
"Biritaniya"@ha,
"בריטניה"@he,
"यू॰के॰"@hi,
"Ujedinjena Kraljevina"@hr,
"Egyesült Királyság"@hu,
"Միացյալ Թագավորություն"@hy,
"Regno Unite"@ia,
"Kerajaan Inggris"@id,
"UK"@ig,
"ꑱꇩ"@ii,
"Bretland"@is,
"Stóra-Bretland"@is,
"Regno Unito"@it,
"英国"@ja,
"KM"@jv,
"გაერთ.სამ."@ka,
"Ngeretha"@ki,
"Ұлыбритания"@kk,
"Tuluit Nunaat"@kl,
"ច.អ."@km,
"ಯು.ಕೆ."@kn,
"영국"@ko,
"یُنایٹِڑ کِنگڈَم"@ks,
"KY"@ku,
"Rywvaneth Unys"@kw,
"УБ"@ky,
"Groussbritannien"@lb,
"Bungereza"@lg,
"Angɛlɛtɛ́lɛ"@ln,
"ສະຫະລາດຊະອະນາຈັກ"@lo,
"Jungtinė Karalystė"@lt,
"Angeletele"@lu,
"Apvienotā Karaliste"@lv,
"Lielbritānijas un Ziemeļīrijas Apvienotā Karaliste"@lv,
"Angletera"@mg,
"Hononga o Piritene"@mi,
"ОК"@mk,
"യുണൈറ്റഡ് കിംഗ്ഡം"@ml,
"ИБ"@mn,
"यू.के."@mr,
"UK"@ms,
"UK"@mt,
"ယူကေ"@my,
"Storbritannia"@nb,
"United Kingdom"@nd,
"युके"@ne,
"Verenigd Koninkrijk"@nl,
"Storbritannia"@no,
"Reiaume Unit"@oc,
"United Kingdom"@om,
"ୟୁକେ"@or,
"Стыр Британи"@os,
"ਯੂ.ਕੇ."@pa,
"Zjednoczone Królestwo Wielkiej Brytanii"@pl,
"برتانیه"@ps,
"Reino Unido"@pt,
"Reino Unido"@qu,
"Reginavel Unì"@rm,
"Ubwongereza"@rn,
"Regatul Unit"@ro,
"Британия"@ru,
"संयुक्त राष्ट्र:"@sa,
"برطانيه"@sd,
"Stuorra-Británnia"@se,
"Ködörögbïä--Ôko"@sg,
"එ.රා"@si,
"UK"@sk,
"Združeno kraljestvo (V. Britanija in S. Irska)"@sl,
"United Kingdom"@sn,
"UK"@so,
"MB"@sq,
"УК"@sr,
"Britania Raya"@su,
"Storbritannien"@sv,
"Ufalme wa Muungano"@sw,
"யூகே"@ta,
"యు.కె."@te,
"Шоҳигарии Муттаҳида"@tg,
"สหราชอาณาจักร"@th,
"ዩኬይ"@ti,
"Birleşen Patyşalyk"@tk,
"Pilitānia"@to,
"İngiltere"@tr,
"Берләшкән Корольлек"@tt,
"ئەنگلىيە"@ug,
"Велика Британія"@uk,
"Сполучене Королівство"@uk,
"یو کے"@ur,
"Britaniya"@uz,
"Vương quốc Anh"@vi,
"Ruwaayom Ini"@wo,
"פֿאַראייניגטע קעניגרייך"@yi,
"Gẹ̀ẹ́sì"@yo,
"英国"@zh,
"i-U.K."@zu ;
gn:parentFeature <https://sws.geonames.org/11812257/>,
<https://sws.geonames.org/7729883/> ;
gn:population "66488991" ;
gn:shortName "RU"@br,
"UK"@de,
"Great Britain"@en,
"UK"@en,
"RU"@es,
"VK"@nl,
"Wielka Brytania"@pl ;
gn:wikipediaArticle <https://en.wikipedia.org/wiki/United_Kingdom>,
<https://ru.wikipedia.org/wiki/%D0%92%D0%B5%D0%BB%D0%B8%D0%BA%D0%BE%D0%B1%D1%80%D0%B8%D1%82%D0%B0%D0%BD%D0%B8%D1%8F> ;
rdfs:isDefinedBy <https://sws.geonames.org/2635167/about.rdf> ;
rdfs:seeAlso <https://dbpedia.org/resource/United_Kingdom> ;
wgs84_pos:lat "54.75844" ;
wgs84_pos:long "-2.69531" .
<https://sws.geonames.org/2635167/about.rdf> a foaf:Document ;
cc:attributionName "GeoNames"^^<https://www.w3.org/2001/XMLSchema#string> ;
cc:attributionURL <https://www.geonames.org> ;
cc:license <https://creativecommons.org/licenses/by/4.0/> ;
dcterms:created "2006-01-15"^^<https://www.w3.org/2001/XMLSchema#date> ;
dcterms:modified "2023-12-16"^^<https://www.w3.org/2001/XMLSchema#date> ;
foaf:primaryTopic <https://sws.geonames.org/2635167/> .
As can be seen, we now retrieved all the information about the parent country of City of Bristol
– the UK
that are provided in GeoNames. Likewise, you can further search the graph by building similar queries. Here, I only showed you to create a graph and do some simple query of getting information about a specific node. In fact, rdflib
is way more powerful than these simple queries. It supports the full functionality of SPARQL query (the graph version of SQL). Check its documentation to learn more.
Part 1: Word2Vec to Learn Semantic Similarity between Place Types#
In following parts, we will go through experiments of computing word embedding, document embedding, topic modeling, and embedding visualization. It involves the implementaton of several state-of-the-art techniques, including word2vec, TSNS, and LDA. We will use the same data as Lab 03. Similarities and differences between Lab 3 and Lab 4 will also be discussed.
Used packages include:
Yes! You have already used most of these libraries.
We will learn two more new libraries here: gensim
and pyLDAvis
. Particularly for gensim
, it is an important library in modern natural language processing. Make sure your installed gensim
is in the version of 4.2.0 or later.
import gensim
print(gensim.__version__)
4.3.2
The first part is about to train a Word2Vec model using the library gensim
. Similar to Lab 03, we want to represent place types (unstructured words representing the category of places/POIs) using a numeric vector. Different from Lab 03, we want to capture more semantics (e.g., how words are used together in the document) than simply counting the frequency of terms occurred in the document. As discussed in the lecture, Wrod2Vec is the fundemental for most state-of-the-art natural language processing techniques, such as BERT, which is developed by Google and has been used in their search engine, and the famous ChatGPT, developed by OpenAI.
Before we use the model, we must load the data. Here in the tutorial, we will use exactly the same data you created from Lab 03, i.e., the bristol_lsoal_poi_full
dataframe. Recall that it is a dataframe with each row represent a LSOA11 region, and it contains a column named fclass
that includes POI types that have been seen in this region. Like Lab 03, we will regard each LSOA11 region as a document and the POI types from the fclass
column as words composing the document. However, you should note that such a setup is simply for us to do the experiment as the vocabulary here (all unique POI types) is relatively in small size and the number of documents (regions) is also small. Data-driven techniques (e.g. Neural Network), such as Word2Vec, usually require a large data/corpus. In your future projects, you’d better not limit yourself to only small datasets (even though starting from a relatively smaller data is always a good idea).
In case you have not saved the bristol_lsoal_poi_full
dataframe, I have prepared it for you and you can download it from the Blackboard. Once you get the data, you can use the code below to load it. Remember to assign the right crs to the dataframe. Also worth noting that the parameter wkt.loads
is to tell the dataframe that the coloumn geom
is as the WKT format. The converters = {}
parameter is to tell pandas
that while loading the data, delete the list bracket []
and then split the string using ,
. Try to see what you load if you don’t use such a converters = {}
.
# load the data
import geopandas as gpd
import pandas as pd
import json
from shapely.geometry import shape
from shapely import wkt
import gensim # make sure it is in the version of 4.2.0 or later
bristol_lsoal_poi_full = pd.read_csv('bristol_lsoal_poi_full.csv', sep = '\t',converters={"fclass": lambda x: x.strip("[]").split(", ")})
bristol_lsoal_poi_full['geom'] = bristol_lsoal_poi_full['geom'].apply(wkt.loads)
bristol_lsoal_poi_full = gpd.GeoDataFrame(bristol_lsoal_poi_full,crs="EPSG:4326", geometry= "geom")
After loading the data, we need to prepare a document list (called corpus
here) as the input to the model. This corpus
is a list of list, the outter is a document list, and the inner list is a word list that composing the document. See how it looks like below:
corpus = list(bristol_lsoal_poi_full['fclass'])
corpus
[["'swimming_pool'", "'swimming_pool'", "'playground'", "'park'"],
["'convenience'",
"'convenience'",
"'nursing_home'",
"'college'",
"'hairdresser'",
"'computer_shop'",
"'fast_food'",
"'restaurant'",
"'doityourself'",
"'car_wash'",
"'convenience'",
"'restaurant'",
"'sports_centre'",
"'clinic'",
"'hairdresser'",
"'sports_centre'",
"'doityourself'",
"'car_dealership'",
"'restaurant'",
"'dentist'",
"'kindergarten'",
"'community_centre'",
"'cafe'",
"'doityourself'",
"'butcher'",
"'theatre'",
"'sports_centre'"],
["'kindergarten'",
"'community_centre'",
"'kindergarten'",
"'convenience'",
"'hairdresser'",
"'fast_food'",
"'playground'",
"'park'",
"'park'",
"'convenience'",
"'cafe'",
"'fast_food'",
"'fast_food'",
"'hairdresser'",
"'beauty_shop'"],
["'sports_shop'",
"'beauty_shop'",
"'convenience'",
"'fast_food'",
"'pub'",
"'car_dealership'",
"'playground'",
"'pitch'",
"'pitch'",
"'toy_shop'",
"'playground'"],
["'college'",
"'pub'",
"'restaurant'",
"'pitch'",
"'school'",
"'pitch'",
"'park'",
"'car_dealership'",
"'pitch'",
"'playground'",
"'furniture_shop'",
"'hotel'",
"'pub'",
"'hotel'",
"'kindergarten'",
"'convenience'",
"'fast_food'",
"'fast_food'"],
["'convenience'",
"'beauty_shop'",
"'fast_food'",
"'pharmacy'",
"'hairdresser'",
"'pub'",
"'fast_food'",
"'park'",
"'doityourself'",
"'car_dealership'",
"'doityourself'",
"'car_wash'",
"'doityourself'"],
["'bakery'",
"'pub'",
"'pub'",
"'pub'",
"'restaurant'",
"'sports_centre'",
"'gift_shop'",
"'pub'",
"'convenience'",
"'greengrocer'"],
["'museum'",
"'restaurant'",
"'convenience'",
"'clothes'",
"'restaurant'",
"'nightclub'",
"'beverages'",
"'hairdresser'",
"'fast_food'",
"'pub'",
"'hairdresser'",
"'restaurant'",
"'bar'",
"'nightclub'",
"'theatre'",
"'hairdresser'",
"'arts_centre'",
"'park'",
"'park'",
"'fast_food'",
"'pub'",
"'park'",
"'fast_food'",
"'park'",
"'fast_food'",
"'beauty_shop'",
"'supermarket'",
"'furniture_shop'",
"'fast_food'",
"'restaurant'",
"'bar'",
"'hairdresser'",
"'convenience'",
"'fast_food'",
"'park'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'cafe'",
"'cafe'",
"'fast_food'",
"'restaurant'",
"'restaurant'",
"'beauty_shop'",
"'pub'",
"'fountain'",
"'restaurant'",
"'cafe'",
"'fountain'",
"'hairdresser'",
"'clothes'",
"'fast_food'",
"'fountain'",
"'cafe'",
"'bar'",
"'restaurant'",
"'theatre'",
"'fast_food'",
"'supermarket'",
"'pub'",
"'restaurant'",
"'bar'",
"'hairdresser'",
"'pharmacy'",
"'nightclub'",
"'toilet'",
"'cafe'",
"'restaurant'",
"'community_centre'",
"'hairdresser'",
"'sports_centre'",
"'cafe'",
"'restaurant'",
"'cafe'",
"'hairdresser'",
"'cafe'",
"'clinic'",
"'cafe'",
"'library'",
"'fountain'",
"'fountain'",
"'town_hall'",
"'attraction'",
"'cafe'",
"'arts_centre'",
"'bar'",
"'pub'",
"'restaurant'",
"'pub'",
"'restaurant'",
"'nightclub'",
"'bar'",
"'restaurant'",
"'museum'",
"'restaurant'",
"'restaurant'",
"'pub'",
"'pub'",
"'school'",
"'pub'",
"'cafe'",
"'cinema'",
"'hotel'",
"'bar'",
"'tourist_info'",
"'restaurant'",
"'cafe'",
"'cafe'",
"'restaurant'",
"'gift_shop'",
"'florist'",
"'restaurant'",
"'fast_food'",
"'restaurant'",
"'beverages'",
"'hairdresser'",
"'beverages'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'cafe'"],
["'nursing_home'",
"'convenience'",
"'bakery'",
"'school'",
"'car_dealership'",
"'hairdresser'",
"'park'",
"'playground'",
"'school'",
"'car_dealership'",
"'convenience'",
"'doityourself'",
"'hairdresser'",
"'convenience'",
"'doityourself'"],
["'college'",
"'park'",
"'school'",
"'pitch'",
"'park'",
"'sports_centre'",
"'park'",
"'track'",
"'fast_food'"],
["'pub'",
"'cafe'",
"'restaurant'",
"'doityourself'",
"'pub'",
"'clinic'",
"'sports_shop'",
"'fast_food'",
"'fast_food'",
"'outdoor_shop'",
"'pub'",
"'convenience'",
"'fast_food'",
"'restaurant'",
"'pub'",
"'convenience'",
"'veterinary'",
"'sports_centre'",
"'clinic'",
"'dentist'",
"'playground'",
"'pub'"],
["'convenience'",
"'butcher'",
"'gift_shop'",
"'mobile_phone_shop'",
"'pub'",
"'fast_food'",
"'fast_food'",
"'clothes'",
"'cafe'",
"'park'",
"'playground'",
"'park'",
"'pub'",
"'convenience'",
"'restaurant'",
"'restaurant'",
"'fast_food'",
"'hairdresser'",
"'restaurant'",
"'hairdresser'",
"'pub'",
"'restaurant'",
"'convenience'",
"'supermarket'",
"'restaurant'",
"'supermarket'",
"'pharmacy'",
"'butcher'",
"'restaurant'",
"'restaurant'",
"'cafe'",
"'beauty_shop'",
"'fast_food'",
"'travel_agent'",
"'travel_agent'",
"'pharmacy'",
"'bakery'",
"'supermarket'",
"'cafe'",
"'restaurant'",
"'hairdresser'",
"'clothes'",
"'fast_food'",
"'restaurant'",
"'fast_food'",
"'beauty_shop'",
"'hairdresser'",
"'supermarket'",
"'toy_shop'",
"'hairdresser'",
"'convenience'",
"'pub'",
"'fast_food'",
"'car_wash'",
"'clothes'",
"'playground'",
"'bakery'",
"'butcher'",
"'convenience'"],
["'sports_centre'",
"'convenience'",
"'car_rental'",
"'arts_centre'",
"'fast_food'",
"'fast_food'",
"'shoe_shop'",
"'clothes'",
"'mobile_phone_shop'",
"'fast_food'",
"'beauty_shop'",
"'fast_food'",
"'chemist'",
"'cafe'",
"'beverages'",
"'cafe'",
"'hairdresser'",
"'mobile_phone_shop'",
"'hairdresser'",
"'mobile_phone_shop'",
"'hairdresser'",
"'jeweller'",
"'optician'",
"'beauty_shop'",
"'mobile_phone_shop'",
"'beauty_shop'",
"'post_office'",
"'clothes'",
"'optician'",
"'pub'",
"'car_dealership'",
"'supermarket'",
"'car_wash'",
"'park'",
"'pub'",
"'cafe'",
"'mobile_phone_shop'",
"'hairdresser'",
"'bank'",
"'mobile_phone_shop'",
"'bank'",
"'mobile_phone_shop'",
"'hairdresser'",
"'gift_shop'",
"'travel_agent'",
"'cafe'",
"'fast_food'",
"'fast_food'",
"'greengrocer'",
"'cafe'",
"'butcher'",
"'toilet'",
"'pitch'",
"'playground'",
"'nightclub'",
"'pub'",
"'fast_food'",
"'pub'",
"'hairdresser'",
"'fast_food'",
"'cafe'",
"'furniture_shop'",
"'pharmacy'",
"'doctors'",
"'restaurant'",
"'library'",
"'clinic'",
"'hairdresser'",
"'convenience'",
"'cafe'",
"'outdoor_shop'",
"'furniture_shop'",
"'pub'",
"'cafe'",
"'fast_food'",
"'pub'"],
["'playground'", "'tower'", "'pitch'"],
["'hairdresser'",
"'fast_food'",
"'hairdresser'",
"'dentist'",
"'university'",
"'hotel'",
"'pub'",
"'hotel'",
"'clinic'",
"'restaurant'",
"'bookshop'",
"'park'",
"'restaurant'",
"'laundry'",
"'convenience'",
"'pub'",
"'bar'",
"'pub'",
"'hairdresser'",
"'university'",
"'school'",
"'swimming_pool'",
"'kindergarten'",
"'restaurant'",
"'cafe'",
"'convenience'",
"'fast_food'"],
["'school'",
"'pitch'",
"'park'",
"'convenience'",
"'pitch'",
"'community_centre'",
"'park'"],
["'school'", "'post_office'"],
["'cafe'",
"'toilet'",
"'pitch'",
"'shelter'",
"'park'",
"'mall'",
"'playground'",
"'pitch'",
"'fast_food'",
"'hairdresser'",
"'florist'",
"'bakery'",
"'hairdresser'",
"'car_wash'",
"'community_centre'",
"'bank'",
"'bank'",
"'fast_food'",
"'gift_shop'",
"'dentist'",
"'kindergarten'",
"'veterinary'",
"'school'",
"'kindergarten'",
"'fast_food'",
"'newsagent'",
"'clinic'",
"'pub'",
"'park'",
"'graveyard'",
"'pitch'",
"'pitch'"],
["'dentist'",
"'pub'",
"'pitch'",
"'bakery'",
"'beauty_shop'",
"'hairdresser'",
"'fast_food'",
"'pharmacy'",
"'fast_food'",
"'pub'",
"'furniture_shop'",
"'newsagent'",
"'beverages'",
"'hairdresser'",
"'convenience'",
"'pitch'",
"'pitch'",
"'school'",
"'doityourself'",
"'convenience'",
"'beauty_shop'",
"'beverages'",
"'fast_food'",
"'car_dealership'",
"'optician'",
"'convenience'",
"'post_office'",
"'fast_food'",
"'beverages'",
"'restaurant'",
"'pub'",
"'fast_food'",
"'bakery'",
"'restaurant'",
"'beverages'"],
["'school'",
"'playground'",
"'park'",
"'park'",
"'car_dealership'",
"'clothes'",
"'convenience'",
"'convenience'",
"'clinic'",
"'library'",
"'sports_centre'",
"'bicycle_shop'",
"'pitch'",
"'school'",
"'sports_centre'",
"'fast_food'",
"'school'",
"'fast_food'",
"'cafe'",
"'community_centre'",
"'cafe'",
"'convenience'",
"'furniture_shop'",
"'cafe'",
"'clothes'",
"'optician'",
"'hairdresser'",
"'convenience'",
"'computer_shop'",
"'fast_food'",
"'greengrocer'",
"'restaurant'",
"'fast_food'",
"'hairdresser'",
"'butcher'",
"'cafe'",
"'cafe'",
"'butcher'",
"'fast_food'",
"'mobile_phone_shop'",
"'convenience'",
"'travel_agent'",
"'fast_food'",
"'mobile_phone_shop'",
"'furniture_shop'",
"'butcher'",
"'pharmacy'",
"'cafe'",
"'park'",
"'hairdresser'",
"'butcher'",
"'restaurant'",
"'fast_food'",
"'convenience'",
"'restaurant'",
"'playground'",
"'park'",
"'pitch'",
"'pitch'",
"'bar'",
"'bar'",
"'cafe'"],
["'veterinary'",
"'doctors'",
"'pub'",
"'fast_food'",
"'restaurant'",
"'restaurant'",
"'hairdresser'",
"'hairdresser'",
"'community_centre'",
"'graveyard'"],
["'pitch'",
"'school'",
"'pub'",
"'convenience'",
"'cafe'",
"'fast_food'",
"'playground'"],
["'community_centre'",
"'car_dealership'",
"'pharmacy'",
"'hairdresser'",
"'car_dealership'",
"'car_dealership'",
"'car_dealership'",
"'pitch'",
"'pub'",
"'beverages'",
"'convenience'",
"'greengrocer'",
"'cafe'",
"'park'"],
["'hairdresser'", "'hairdresser'", "'newsagent'"],
["'hairdresser'",
"'bakery'",
"'clinic'",
"'swimming_pool'",
"'clinic'",
"'park'",
"'doctors'",
"'dentist'",
"'beauty_shop'",
"'restaurant'",
"'hairdresser'",
"'hairdresser'",
"'bank'",
"'convenience'",
"'fast_food'",
"'fast_food'",
"'restaurant'"],
["'park'",
"'university'",
"'university'",
"'hospital'",
"'university'",
"'university'",
"'cafe'",
"'school'",
"'pitch'",
"'park'",
"'fast_food'",
"'restaurant'",
"'hairdresser'",
"'hairdresser'",
"'convenience'",
"'pharmacy'",
"'convenience'",
"'nursing_home'",
"'pitch'",
"'playground'",
"'pitch'",
"'park'"],
["'clinic'",
"'guesthouse'",
"'park'",
"'beauty_shop'",
"'kindergarten'",
"'community_centre'",
"'park'",
"'park'",
"'park'",
"'fast_food'",
"'fast_food'",
"'pub'",
"'police'",
"'cafe'",
"'hairdresser'",
"'supermarket'"],
["'hairdresser'",
"'cafe'",
"'convenience'",
"'optician'",
"'fast_food'",
"'pharmacy'",
"'pharmacy'",
"'veterinary'",
"'fast_food'",
"'convenience'",
"'hairdresser'",
"'convenience'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'library'",
"'kindergarten'",
"'school'",
"'school'"],
["'park'",
"'hairdresser'",
"'hairdresser'",
"'convenience'",
"'convenience'",
"'fast_food'",
"'fast_food'",
"'florist'",
"'school'",
"'park'"],
["'community_centre'",
"'park'",
"'convenience'",
"'playground'",
"'library'",
"'cafe'",
"'park'",
"'park'",
"'pitch'",
"'playground'"],
["'restaurant'",
"'fast_food'",
"'sports_shop'",
"'fast_food'",
"'pub'",
"'pub'",
"'pharmacy'",
"'restaurant'",
"'bicycle_shop'",
"'bar'",
"'sports_shop'",
"'clothes'",
"'toilet'",
"'shelter'",
"'beauty_shop'",
"'cafe'",
"'bar'",
"'restaurant'",
"'beauty_shop'",
"'doctors'",
"'library'",
"'furniture_shop'",
"'furniture_shop'",
"'restaurant'",
"'cafe'",
"'furniture_shop'",
"'furniture_shop'",
"'furniture_shop'",
"'furniture_shop'",
"'clinic'",
"'supermarket'",
"'community_centre'",
"'furniture_shop'",
"'restaurant'",
"'restaurant'",
"'doityourself'",
"'furniture_shop'",
"'furniture_shop'",
"'police'",
"'cafe'",
"'kindergarten'",
"'shelter'",
"'cafe'",
"'cafe'",
"'optician'",
"'beauty_shop'",
"'school'",
"'restaurant'",
"'kindergarten'",
"'greengrocer'",
"'hairdresser'",
"'convenience'",
"'cafe'",
"'gift_shop'",
"'bookshop'",
"'supermarket'",
"'mall'",
"'laundry'",
"'optician'",
"'pitch'",
"'newsagent'",
"'cafe'",
"'pub'",
"'bar'",
"'convenience'",
"'cafe'",
"'cafe'",
"'hairdresser'",
"'sports_centre'"],
["'playground'",
"'pitch'",
"'sports_centre'",
"'pitch'",
"'laundry'",
"'pharmacy'",
"'hairdresser'",
"'fast_food'",
"'hairdresser'",
"'hairdresser'",
"'supermarket'",
"'fast_food'",
"'convenience'",
"'doityourself'",
"'doctors'",
"'dentist'",
"'community_centre'",
"'pitch'",
"'school'",
"'pitch'",
"'hospital'"],
["'golf_course'",
"'library'",
"'community_centre'",
"'swimming_pool'",
"'school'",
"'pitch'",
"'pitch'",
"'park'",
"'convenience'",
"'beauty_shop'",
"'hairdresser'",
"'park'",
"'beauty_shop'",
"'fast_food'",
"'cafe'",
"'park'",
"'park'",
"'dentist'",
"'park'",
"'playground'",
"'convenience'",
"'park'"],
["'pub'",
"'fast_food'",
"'playground'",
"'pub'",
"'convenience'",
"'park'",
"'doityourself'",
"'park'"],
["'park'",
"'clothes'",
"'hairdresser'",
"'school'",
"'pitch'",
"'park'",
"'museum'",
"'university'",
"'hospital'",
"'school'",
"'optician'",
"'supermarket'",
"'fast_food'",
"'travel_agent'",
"'playground'",
"'park'",
"'library'",
"'car_dealership'",
"'supermarket'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'supermarket'",
"'supermarket'",
"'pub'",
"'bank'",
"'pub'",
"'beauty_shop'",
"'hairdresser'",
"'restaurant'",
"'beauty_shop'",
"'jeweller'",
"'mobile_phone_shop'"],
["'furniture_shop'",
"'cafe'",
"'clinic'",
"'gift_shop'",
"'fast_food'",
"'restaurant'",
"'greengrocer'",
"'mobile_phone_shop'",
"'kindergarten'",
"'park'",
"'playground'",
"'shelter'",
"'park'",
"'pitch'",
"'school'",
"'hairdresser'",
"'clothes'",
"'sports_shop'"],
["'pitch'",
"'pub'",
"'school'",
"'car_rental'",
"'car_dealership'",
"'convenience'",
"'beauty_shop'",
"'fire_station'",
"'playground'",
"'hospital'"],
["'newsagent'",
"'pub'",
"'fast_food'",
"'pub'",
"'hairdresser'",
"'furniture_shop'",
"'park'",
"'bar'",
"'bookshop'",
"'clothes'",
"'doityourself'",
"'cafe'",
"'beauty_shop'",
"'beauty_shop'",
"'jeweller'",
"'cafe'",
"'hairdresser'",
"'car_dealership'",
"'pub'",
"'furniture_shop'",
"'cafe'",
"'fast_food'",
"'clinic'",
"'supermarket'",
"'bicycle_shop'",
"'car_dealership'",
"'florist'",
"'fast_food'",
"'hairdresser'",
"'fast_food'",
"'beverages'",
"'restaurant'",
"'doityourself'",
"'fast_food'",
"'hairdresser'",
"'restaurant'",
"'doityourself'",
"'convenience'"],
["'park'"],
["'pitch'",
"'pitch'",
"'car_dealership'",
"'school'",
"'doctors'",
"'convenience'",
"'car_rental'",
"'fast_food'",
"'supermarket'"],
["'school'",
"'pitch'",
"'swimming_pool'",
"'swimming_pool'",
"'cafe'",
"'park'",
"'school'",
"'hairdresser'",
"'playground'",
"'convenience'",
"'park'",
"'pitch'",
"'nursing_home'",
"'pub'",
"'convenience'",
"'park'",
"'park'"],
["'pub'", "'park'"],
["'pitch'",
"'school'",
"'tower'",
"'beverages'",
"'pub'",
"'hairdresser'",
"'pitch'",
"'pitch'",
"'convenience'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'playground'",
"'park'",
"'graveyard'",
"'kindergarten'"],
["'convenience'",
"'hairdresser'",
"'fast_food'",
"'beverages'",
"'hairdresser'",
"'fast_food'",
"'beauty_shop'",
"'doityourself'",
"'car_wash'",
"'school'",
"'playground'",
"'pitch'",
"'pitch'",
"'park'",
"'pitch'"],
["'hotel'",
"'park'",
"'bar'",
"'hotel'",
"'bar'",
"'cafe'",
"'hairdresser'",
"'convenience'",
"'park'",
"'graveyard'",
"'theatre'",
"'playground'",
"'park'",
"'college'",
"'cafe'",
"'playground'",
"'school'",
"'restaurant'",
"'school'",
"'convenience'",
"'hairdresser'",
"'hotel'",
"'bar'",
"'pub'",
"'hostel'",
"'nightclub'",
"'restaurant'"],
["'park'",
"'hairdresser'",
"'hairdresser'",
"'clinic'",
"'nursing_home'",
"'nursing_home'",
"'hairdresser'",
"'computer_shop'",
"'fast_food'",
"'beverages'",
"'fast_food'",
"'newsagent'",
"'hairdresser'",
"'community_centre'",
"'community_centre'",
"'pitch'",
"'pitch'",
"'pitch'"],
["'park'",
"'archaeological'",
"'wastewater_plant'",
"'recycling_glass'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'police'",
"'car_dealership'",
"'park'",
"'shelter'",
"'car_rental'",
"'car_rental'",
"'fast_food'",
"'bakery'",
"'fast_food'",
"'car_dealership'",
"'playground'",
"'park'",
"'convenience'",
"'museum'",
"'archaeological'",
"'car_dealership'",
"'school'",
"'track'",
"'pitch'",
"'pitch'"],
["'pitch'",
"'pitch'",
"'pitch'",
"'swimming_pool'",
"'car_dealership'",
"'clinic'",
"'hairdresser'",
"'car_dealership'",
"'doityourself'",
"'clinic'"],
["'pub'",
"'pub'",
"'park'",
"'bakery'",
"'pub'",
"'playground'",
"'convenience'",
"'sports_centre'",
"'fast_food'",
"'dentist'",
"'cafe'",
"'pub'",
"'convenience'",
"'fast_food'",
"'restaurant'",
"'supermarket'"],
["'fast_food'",
"'beauty_shop'",
"'convenience'",
"'beauty_shop'",
"'veterinary'",
"'dentist'",
"'restaurant'",
"'hairdresser'"],
["'school'",
"'sports_centre'",
"'community_centre'",
"'school'",
"'pitch'",
"'kindergarten'",
"'playground'",
"'pitch'",
"'pitch'",
"'community_centre'",
"'pitch'",
"'pitch'"],
["'car_dealership'", "'pub'", "'fast_food'", "'hairdresser'"],
["'jeweller'",
"'convenience'",
"'pitch'",
"'school'",
"'pitch'",
"'school'",
"'park'",
"'convenience'",
"'fast_food'",
"'pub'",
"'restaurant'",
"'pub'",
"'park'",
"'playground'",
"'playground'",
"'community_centre'",
"'park'",
"'park'",
"'convenience'",
"'playground'",
"'travel_agent'",
"'restaurant'",
"'post_office'",
"'furniture_shop'",
"'convenience'",
"'laundry'",
"'convenience'",
"'cafe'",
"'butcher'",
"'butcher'",
"'fast_food'",
"'mobile_phone_shop'",
"'beauty_shop'",
"'cafe'",
"'convenience'",
"'supermarket'"],
["'nan'"],
["'park'",
"'hospital'",
"'park'",
"'park'",
"'doctors'",
"'school'",
"'community_centre'",
"'park'"],
["'water_tower'",
"'doctors'",
"'pub'",
"'park'",
"'kindergarten'",
"'community_centre'",
"'swimming_pool'"],
["'school'", "'convenience'", "'library'"],
["'school'",
"'pitch'",
"'pitch'",
"'park'",
"'library'",
"'school'",
"'pitch'",
"'pitch'"],
["'park'",
"'park'",
"'park'",
"'park'",
"'attraction'",
"'supermarket'",
"'camp_site'",
"'pitch'",
"'school'",
"'park'",
"'pitch'",
"'car_rental'",
"'hospital'",
"'supermarket'"],
["'butcher'",
"'convenience'",
"'convenience'",
"'cafe'",
"'supermarket'",
"'fast_food'",
"'convenience'",
"'bakery'",
"'hairdresser'",
"'cafe'",
"'restaurant'",
"'restaurant'",
"'fast_food'",
"'pub'",
"'community_centre'",
"'pitch'",
"'playground'",
"'park'",
"'pub'",
"'pitch'",
"'park'",
"'playground'",
"'pub'",
"'fast_food'",
"'convenience'",
"'cafe'",
"'restaurant'",
"'bank'",
"'pub'",
"'restaurant'",
"'hairdresser'",
"'fast_food'",
"'cafe'",
"'bar'",
"'cafe'",
"'convenience'",
"'cafe'",
"'school'",
"'doctors'",
"'fast_food'",
"'pub'",
"'clinic'",
"'pitch'",
"'school'",
"'convenience'"],
["'hairdresser'",
"'butcher'",
"'convenience'",
"'convenience'",
"'hairdresser'",
"'park'",
"'park'",
"'pub'",
"'convenience'",
"'fast_food'",
"'hairdresser'",
"'fast_food'",
"'fast_food'",
"'convenience'",
"'cafe'",
"'restaurant'",
"'fast_food'",
"'pharmacy'",
"'hairdresser'",
"'bicycle_shop'",
"'convenience'",
"'convenience'",
"'fast_food'",
"'hairdresser'",
"'beauty_shop'",
"'restaurant'",
"'community_centre'",
"'hairdresser'",
"'park'",
"'butcher'",
"'dentist'",
"'mobile_phone_shop'",
"'clothes'",
"'convenience'",
"'hairdresser'",
"'convenience'",
"'butcher'",
"'travel_agent'",
"'clinic'",
"'restaurant'",
"'florist'",
"'laundry'",
"'fast_food'",
"'travel_agent'",
"'hairdresser'",
"'convenience'",
"'car_wash'",
"'clinic'",
"'fast_food'",
"'convenience'",
"'pub'",
"'greengrocer'",
"'fast_food'",
"'fast_food'",
"'playground'",
"'pitch'"],
["'pitch'",
"'community_centre'",
"'convenience'",
"'fast_food'",
"'fast_food'",
"'pub'",
"'park'",
"'park'"],
["'bakery'",
"'hairdresser'",
"'toy_shop'",
"'cafe'",
"'school'",
"'pub'",
"'school'",
"'newsagent'",
"'park'"],
["'cafe'",
"'optician'",
"'newsagent'",
"'fast_food'",
"'hairdresser'",
"'beverages'",
"'travel_agent'",
"'doctors'",
"'doctors'",
"'mall'",
"'fast_food'",
"'hairdresser'",
"'bicycle_shop'",
"'bar'",
"'convenience'",
"'florist'",
"'beauty_shop'",
"'bakery'",
"'beauty_shop'",
"'beauty_shop'",
"'park'",
"'convenience'",
"'community_centre'",
"'school'"],
["'park'",
"'park'",
"'playground'",
"'park'",
"'fast_food'",
"'community_centre'",
"'park'",
"'doctors'",
"'pub'",
"'beverages'",
"'bakery'",
"'park'",
"'bar'",
"'bar'",
"'car_dealership'",
"'car_dealership'",
"'fast_food'",
"'hairdresser'",
"'sports_shop'",
"'convenience'",
"'restaurant'",
"'fast_food'",
"'convenience'",
"'fast_food'",
"'restaurant'",
"'school'"],
["'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'park'",
"'playground'",
"'pub'",
"'water_works'",
"'car_wash'",
"'park'",
"'cafe'",
"'car_dealership'",
"'car_wash'",
"'park'",
"'pharmacy'",
"'fast_food'",
"'fast_food'",
"'doctors'",
"'cafe'",
"'supermarket'"],
["'pub'",
"'community_centre'",
"'playground'",
"'cafe'",
"'toilet'",
"'pitch'",
"'playground'",
"'pitch'",
"'school'",
"'pitch'",
"'park'",
"'pitch'",
"'shelter'"],
["'park'",
"'attraction'",
"'community_centre'",
"'furniture_shop'",
"'supermarket'",
"'market_place'",
"'supermarket'",
"'fast_food'",
"'supermarket'",
"'pharmacy'",
"'cafe'",
"'laundry'",
"'car_wash'",
"'department_store'",
"'doctors'",
"'playground'",
"'furniture_shop'",
"'bicycle_shop'",
"'fast_food'",
"'convenience'",
"'veterinary'",
"'hairdresser'",
"'hairdresser'",
"'school'",
"'community_centre'",
"'school'",
"'park'",
"'school'"],
["'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'swimming_pool'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'sports_centre'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'water_works'",
"'playground'",
"'park'",
"'pitch'",
"'cafe'",
"'school'",
"'fast_food'",
"'cafe'",
"'school'",
"'hotel'",
"'kindergarten'",
"'car_dealership'",
"'pitch'",
"'cafe'",
"'doityourself'",
"'doityourself'",
"'sports_centre'",
"'car_dealership'",
"'ruins'"],
["'park'",
"'nursing_home'",
"'doityourself'",
"'fast_food'",
"'fast_food'",
"'hairdresser'",
"'community_centre'",
"'pub'",
"'convenience'",
"'car_dealership'",
"'school'",
"'pitch'",
"'park'",
"'park'",
"'doityourself'",
"'convenience'",
"'dentist'",
"'doityourself'"],
["'beauty_shop'",
"'bicycle_shop'",
"'beverages'",
"'restaurant'",
"'supermarket'",
"'restaurant'",
"'fast_food'",
"'dentist'",
"'beauty_shop'",
"'hairdresser'",
"'hairdresser'",
"'swimming_pool'",
"'pitch'",
"'school'",
"'dentist'",
"'playground'",
"'college'"],
["'hotel'",
"'furniture_shop'",
"'shoe_shop'",
"'pub'",
"'hairdresser'",
"'fast_food'",
"'restaurant'",
"'pub'",
"'clothes'",
"'bar'",
"'fast_food'",
"'laundry'",
"'restaurant'",
"'beauty_shop'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'furniture_shop'",
"'restaurant'",
"'convenience'",
"'restaurant'"],
["'beauty_shop'",
"'car_dealership'",
"'fast_food'",
"'convenience'",
"'convenience'",
"'hairdresser'",
"'hairdresser'",
"'car_dealership'",
"'car_dealership'",
"'pub'",
"'pub'",
"'school'",
"'computer_shop'",
"'hairdresser'",
"'pub'"],
["'supermarket'", "'furniture_shop'", "'school'"],
["'park'",
"'school'",
"'doityourself'",
"'doityourself'",
"'park'",
"'park'",
"'park'",
"'shelter'",
"'playground'",
"'pitch'",
"'park'",
"'pitch'",
"'pitch'",
"'pub'",
"'park'"],
["'park'",
"'school'",
"'pitch'",
"'kindergarten'",
"'swimming_pool'",
"'swimming_pool'",
"'park'"],
["'car_wash'",
"'convenience'",
"'pharmacy'",
"'doctors'",
"'supermarket'",
"'dentist'",
"'park'",
"'community_centre'"],
["'cafe'", "'veterinary'", "'library'", "'pub'", "'school'", "'fast_food'"],
["'playground'",
"'park'",
"'attraction'",
"'school'",
"'sports_centre'",
"'school'"],
["'fast_food'",
"'clinic'",
"'convenience'",
"'beauty_shop'",
"'fast_food'",
"'butcher'",
"'hairdresser'",
"'fast_food'",
"'fast_food'",
"'convenience'",
"'pub'",
"'pitch'",
"'pitch'",
"'pub'",
"'pitch'",
"'graveyard'",
"'park'",
"'school'",
"'playground'"],
["'park'",
"'pub'",
"'hairdresser'",
"'fast_food'",
"'convenience'",
"'graveyard'",
"'school'"],
["'graveyard'",
"'park'",
"'pub'",
"'kindergarten'",
"'community_centre'",
"'car_dealership'"],
["'convenience'",
"'beverages'",
"'hairdresser'",
"'fast_food'",
"'beauty_shop'",
"'hairdresser'",
"'fast_food'",
"'supermarket'",
"'supermarket'",
"'fast_food'",
"'greengrocer'",
"'park'",
"'school'",
"'school'",
"'pitch'",
"'park'",
"'playground'",
"'community_centre'",
"'community_centre'",
"'school'",
"'pitch'"],
["'hairdresser'",
"'supermarket'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'school'",
"'school'",
"'school'",
"'swimming_pool'"],
["'playground'",
"'car_dealership'",
"'furniture_shop'",
"'clothes'",
"'furniture_shop'",
"'sports_shop'",
"'clothes'",
"'fast_food'",
"'track'",
"'track'",
"'golf_course'",
"'pitch'",
"'track'",
"'school'",
"'track'",
"'playground'",
"'park'",
"'community_centre'",
"'park'"],
["'car_dealership'",
"'car_wash'",
"'doctors'",
"'pitch'",
"'playground'",
"'school'",
"'nursing_home'",
"'convenience'",
"'doityourself'",
"'convenience'",
"'hairdresser'"],
["'school'",
"'pitch'",
"'school'",
"'pharmacy'",
"'doctors'",
"'supermarket'",
"'community_centre'",
"'doityourself'"],
["'park'",
"'school'",
"'playground'",
"'playground'",
"'pitch'",
"'playground'",
"'park'"],
["'pitch'",
"'restaurant'",
"'pub'",
"'furniture_shop'",
"'optician'",
"'hairdresser'",
"'clothes'",
"'supermarket'",
"'pub'",
"'clinic'",
"'bar'",
"'restaurant'",
"'cafe'",
"'convenience'",
"'restaurant'",
"'convenience'",
"'greengrocer'",
"'fast_food'",
"'hairdresser'",
"'fast_food'",
"'bar'",
"'fast_food'",
"'pub'",
"'hairdresser'",
"'computer_shop'",
"'supermarket'",
"'convenience'",
"'fast_food'",
"'beverages'",
"'convenience'",
"'restaurant'",
"'pharmacy'",
"'restaurant'",
"'fast_food'",
"'hairdresser'",
"'hairdresser'",
"'dentist'",
"'cafe'",
"'restaurant'",
"'clothes'",
"'cafe'",
"'clothes'",
"'bakery'",
"'bar'",
"'pharmacy'",
"'hairdresser'",
"'mobile_phone_shop'",
"'clothes'",
"'pub'",
"'fast_food'",
"'laundry'",
"'convenience'",
"'florist'",
"'restaurant'",
"'swimming_pool'",
"'park'",
"'playground'"],
["'bakery'",
"'fast_food'",
"'pub'",
"'restaurant'",
"'restaurant'",
"'convenience'",
"'community_centre'",
"'hairdresser'",
"'pub'",
"'pub'",
"'fast_food'"],
["'pitch'", "'pitch'", "'school'", "'pitch'", "'pub'", "'park'"],
["'pub'",
"'library'",
"'florist'",
"'hairdresser'",
"'beauty_shop'",
"'hairdresser'",
"'fast_food'",
"'furniture_shop'",
"'beauty_shop'",
"'jeweller'",
"'hairdresser'",
"'beauty_shop'",
"'supermarket'",
"'clothes'"],
["'pitch'", "'playground'", "'school'"],
["'supermarket'",
"'pharmacy'",
"'beverages'",
"'hairdresser'",
"'convenience'",
"'bakery'",
"'cafe'",
"'video_shop'",
"'convenience'",
"'fast_food'",
"'beauty_shop'",
"'fast_food'",
"'fast_food'",
"'clothes'",
"'hairdresser'",
"'car_dealership'",
"'cafe'",
"'florist'",
"'butcher'",
"'hairdresser'",
"'fast_food'",
"'fast_food'",
"'optician'",
"'bakery'",
"'convenience'",
"'newsagent'",
"'post_office'",
"'hairdresser'",
"'fast_food'",
"'beauty_shop'",
"'fast_food'",
"'hairdresser'",
"'hairdresser'",
"'school'"],
["'veterinary'",
"'sports_centre'",
"'school'",
"'school'",
"'pitch'",
"'school'",
"'pitch'",
"'park'",
"'swimming_pool'",
"'kindergarten'",
"'school'",
"'school'",
"'park'",
"'swimming_pool'"],
["'school'", "'golf_course'", "'park'"],
["'pub'",
"'golf_course'",
"'convenience'",
"'pitch'",
"'school'",
"'bar'",
"'kindergarten'",
"'water_works'",
"'playground'",
"'park'",
"'hairdresser'"],
["'garden_centre'",
"'doityourself'",
"'pitch'",
"'pitch'",
"'community_centre'",
"'convenience'",
"'school'",
"'pitch'",
"'car_dealership'",
"'sports_centre'"],
["'pub'",
"'pub'",
"'kindergarten'",
"'convenience'",
"'hairdresser'",
"'beauty_shop'",
"'pub'",
"'bakery'",
"'beauty_shop'",
"'restaurant'",
"'sports_centre'",
"'kindergarten'",
"'school'",
"'dentist'",
"'convenience'",
"'school'",
"'restaurant'",
"'bicycle_shop'",
"'restaurant'",
"'nightclub'",
"'convenience'",
"'cafe'",
"'hairdresser'",
"'cafe'",
"'supermarket'",
"'clinic'",
"'fast_food'",
"'beauty_shop'",
"'hairdresser'",
"'bicycle_shop'"],
["'convenience'",
"'pitch'",
"'car_wash'",
"'pitch'",
"'pitch'",
"'pitch'",
"'park'",
"'pitch'",
"'school'",
"'pitch'",
"'furniture_shop'",
"'playground'",
"'park'"],
["'track'",
"'graveyard'",
"'guesthouse'",
"'pitch'",
"'playground'",
"'furniture_shop'",
"'hotel'",
"'supermarket'",
"'park'",
"'graveyard'",
"'park'",
"'park'",
"'hospital'",
"'pitch'"],
["'fast_food'",
"'hairdresser'",
"'recycling'",
"'toy_shop'",
"'restaurant'",
"'beauty_shop'",
"'hairdresser'",
"'supermarket'",
"'convenience'",
"'restaurant'",
"'toy_shop'",
"'fast_food'",
"'toilet'",
"'fast_food'",
"'community_centre'",
"'bar'",
"'car_dealership'",
"'cafe'",
"'library'",
"'playground'",
"'cafe'",
"'park'",
"'pitch'",
"'pitch'",
"'pitch'",
"'graveyard'",
"'beauty_shop'",
"'fast_food'",
"'hairdresser'",
"'hairdresser'",
"'car_dealership'"],
["'school'",
"'bank'",
"'kindergarten'",
"'pub'",
"'car_wash'",
"'hairdresser'"],
["'veterinary'",
"'pub'",
"'dentist'",
"'car_wash'",
"'car_dealership'",
"'cafe'",
"'hairdresser'",
"'hairdresser'",
"'newsagent'",
"'restaurant'",
"'supermarket'",
"'dentist'",
"'convenience'",
"'park'",
"'graveyard'"],
["'convenience'",
"'fast_food'",
"'pub'",
"'hairdresser'",
"'convenience'",
"'pharmacy'",
"'butcher'",
"'hairdresser'",
"'supermarket'",
"'school'",
"'dentist'",
"'guesthouse'",
"'kindergarten'"],
["'playground'", "'park'", "'pitch'", "'pub'", "'pub'"],
["'restaurant'",
"'fast_food'",
"'hairdresser'",
"'fast_food'",
"'beverages'",
"'graveyard'",
"'pub'",
"'beauty_shop'",
"'car_dealership'",
"'beauty_shop'",
"'hairdresser'",
"'convenience'",
"'beauty_shop'",
"'outdoor_shop'",
"'laundry'",
"'fast_food'",
"'restaurant'",
"'butcher'",
"'hairdresser'",
"'fast_food'",
"'hairdresser'",
"'cafe'",
"'laundry'",
"'beauty_shop'",
"'hairdresser'",
"'fast_food'",
"'greengrocer'",
"'hairdresser'",
"'convenience'",
"'bakery'",
"'convenience'",
"'shelter'",
"'post_office'",
"'hairdresser'",
"'beauty_shop'",
"'bicycle_shop'",
"'pub'",
"'restaurant'",
"'fast_food'",
"'convenience'",
"'supermarket'",
"'pub'",
"'castle'",
"'car_dealership'",
"'car_dealership'",
"'car_dealership'",
"'car_dealership'",
"'school'",
"'newsagent'",
"'beauty_shop'"],
["'park'",
"'supermarket'",
"'library'",
"'fast_food'",
"'pub'",
"'school'",
"'school'",
"'school'",
"'convenience'",
"'school'"],
["'pub'",
"'supermarket'",
"'fast_food'",
"'fire_station'",
"'hotel'",
"'college'",
"'memorial'",
"'tower'",
"'park'",
"'pub'",
"'restaurant'",
"'supermarket'",
"'fast_food'",
"'cafe'",
"'pub'",
"'pub'",
"'bar'",
"'cafe'",
"'courthouse'",
"'kindergarten'",
"'cafe'",
"'newsagent'",
"'clinic'",
"'fast_food'",
"'restaurant'",
"'school'",
"'hotel'",
"'restaurant'",
"'park'",
"'hotel'",
"'hotel'",
"'fast_food'",
"'bank'",
"'hairdresser'",
"'hotel'",
"'pub'",
"'restaurant'",
"'fast_food'",
"'bakery'",
"'laundry'",
"'fast_food'",
"'park'",
"'cafe'",
"'hairdresser'",
"'convenience'",
"'pub'",
"'fast_food'",
"'ruins'",
"'pub'",
"'cafe'"],
["'park'",
"'pitch'",
"'pitch'",
"'pitch'",
"'toilet'",
"'playground'",
"'park'",
"'park'",
"'school'",
"'swimming_pool'",
"'restaurant'",
"'convenience'",
"'car_rental'",
"'museum'",
"'pitch'",
"'school'",
"'playground'",
"'pitch'",
"'community_centre'",
"'park'",
"'swimming_pool'"],
["'school'", "'pitch'"],
["'police'",
"'school'",
"'park'",
"'fast_food'",
"'fast_food'",
"'convenience'"],
["'school'",
"'playground'",
"'playground'",
"'school'",
"'pitch'",
"'pitch'",
"'car_dealership'",
"'beauty_shop'",
"'doityourself'",
"'car_dealership'",
"'mobile_phone_shop'",
"'fast_food'",
"'fast_food'",
"'pub'",
"'fast_food'",
"'hairdresser'",
"'hairdresser'",
"'hairdresser'",
"'hairdresser'",
"'hairdresser'",
"'hairdresser'",
"'cafe'",
"'doityourself'",
"'supermarket'",
"'doityourself'",
"'doityourself'",
"'pitch'",
"'pitch'",
"'pitch'",
"'park'",
"'pitch'",
"'fast_food'",
"'convenience'",
"'fast_food'",
"'fast_food'"],
["'pitch'",
"'pitch'",
"'shelter'",
"'school'",
"'school'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'"],
["'hairdresser'", "'fast_food'", "'convenience'", "'pitch'", "'stadium'"],
["'pitch'",
"'school'",
"'track'",
"'pitch'",
"'fast_food'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'beverages'"],
["'bar'",
"'doityourself'",
"'park'",
"'playground'",
"'school'",
"'restaurant'",
"'fast_food'",
"'hairdresser'",
"'fast_food'",
"'market_place'",
"'car_dealership'",
"'cafe'",
"'car_rental'",
"'cafe'",
"'car_dealership'",
"'car_dealership'",
"'cafe'",
"'newsagent'",
"'hairdresser'",
"'car_dealership'",
"'car_rental'",
"'ruins'",
"'park'",
"'playground'",
"'pitch'",
"'stationery'",
"'cafe'",
"'car_dealership'",
"'graveyard'",
"'pub'",
"'doityourself'",
"'pitch'",
"'doityourself'",
"'hotel'"],
["'computer_shop'",
"'beauty_shop'",
"'sports_shop'",
"'hairdresser'",
"'convenience'"],
["'guesthouse'",
"'hairdresser'",
"'hairdresser'",
"'doityourself'",
"'guesthouse'",
"'guesthouse'"],
["'fast_food'",
"'furniture_shop'",
"'butcher'",
"'newsagent'",
"'restaurant'",
"'furniture_shop'",
"'school'",
"'park'",
"'stationery'",
"'fast_food'",
"'hairdresser'",
"'convenience'",
"'car_wash'",
"'convenience'",
"'community_centre'",
"'park'",
"'community_centre'",
"'clothes'",
"'hairdresser'",
"'hairdresser'",
"'cafe'",
"'fast_food'",
"'convenience'",
"'convenience'",
"'fast_food'",
"'dentist'",
"'convenience'",
"'travel_agent'",
"'convenience'",
"'pub'",
"'travel_agent'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'hairdresser'",
"'supermarket'",
"'restaurant'",
"'restaurant'",
"'pitch'",
"'pitch'",
"'park'",
"'convenience'",
"'school'",
"'pub'",
"'clinic'",
"'computer_shop'",
"'pub'",
"'playground'",
"'playground'",
"'park'",
"'park'",
"'pitch'",
"'playground'",
"'hairdresser'",
"'restaurant'",
"'restaurant'",
"'convenience'",
"'laundry'",
"'post_office'",
"'fast_food'",
"'fast_food'",
"'playground'",
"'bicycle_shop'",
"'sports_centre'",
"'pitch'",
"'pitch'",
"'pitch'",
"'cafe'",
"'pitch'",
"'school'",
"'laundry'",
"'hairdresser'",
"'convenience'",
"'butcher'"],
["'playground'",
"'clinic'",
"'playground'",
"'dentist'",
"'furniture_shop'",
"'fast_food'",
"'pub'",
"'restaurant'",
"'playground'",
"'clinic'",
"'pub'",
"'school'",
"'pub'"],
["'bakery'",
"'car_dealership'",
"'car_dealership'",
"'doityourself'",
"'doityourself'",
"'car_dealership'",
"'supermarket'",
"'doityourself'",
"'car_dealership'",
"'car_wash'",
"'car_dealership'",
"'car_dealership'",
"'fast_food'",
"'sports_shop'",
"'fast_food'",
"'beverages'",
"'newsagent'",
"'hairdresser'",
"'mobile_phone_shop'",
"'pub'",
"'park'"],
["'college'",
"'stadium'",
"'pitch'",
"'school'",
"'pub'",
"'pub'",
"'kindergarten'",
"'restaurant'",
"'restaurant'",
"'optician'",
"'cafe'",
"'doityourself'",
"'fast_food'",
"'fast_food'",
"'restaurant'",
"'cafe'",
"'newsagent'",
"'cafe'",
"'laundry'",
"'laundry'",
"'bicycle_shop'",
"'gift_shop'",
"'hairdresser'",
"'cafe'",
"'bank'",
"'kindergarten'",
"'pub'",
"'car_wash'",
"'butcher'",
"'theatre'",
"'beauty_shop'",
"'hairdresser'",
"'sports_centre'",
"'restaurant'",
"'florist'",
"'greengrocer'",
"'car_dealership'",
"'furniture_shop'",
"'gift_shop'",
"'pub'",
"'newsagent'",
"'cafe'",
"'shoe_shop'",
"'gift_shop'",
"'beauty_shop'",
"'hairdresser'",
"'veterinary'",
"'cafe'",
"'bicycle_shop'",
"'furniture_shop'",
"'hairdresser'",
"'florist'",
"'toy_shop'",
"'bar'",
"'restaurant'",
"'fast_food'",
"'fast_food'",
"'bakery'",
"'pub'",
"'convenience'",
"'cafe'",
"'prison'"],
["'convenience'",
"'fast_food'",
"'beauty_shop'",
"'convenience'",
"'fast_food'",
"'florist'",
"'convenience'",
"'fast_food'",
"'hairdresser'",
"'supermarket'",
"'park'",
"'school'",
"'kindergarten'",
"'pitch'",
"'pitch'",
"'park'",
"'playground'",
"'kindergarten'",
"'clinic'",
"'park'",
"'clinic'",
"'park'",
"'fire_station'",
"'car_rental'",
"'car_dealership'"],
["'fast_food'",
"'convenience'",
"'hairdresser'",
"'florist'",
"'fast_food'",
"'hairdresser'",
"'convenience'",
"'beverages'",
"'fast_food'",
"'cafe'",
"'fast_food'",
"'laundry'",
"'car_dealership'",
"'pub'",
"'butcher'",
"'pub'"],
["'clothes'",
"'pub'",
"'pitch'",
"'clothes'",
"'park'",
"'nightclub'",
"'hotel'",
"'car_rental'",
"'doityourself'",
"'doityourself'",
"'sports_centre'",
"'pub'",
"'nightclub'",
"'pub'",
"'cafe'",
"'dentist'",
"'pub'",
"'cafe'",
"'restaurant'",
"'pub'",
"'fast_food'",
"'hairdresser'",
"'clothes'",
"'pub'",
"'dentist'",
"'pub'",
"'stationery'",
"'convenience'",
"'fast_food'",
"'bicycle_shop'",
"'bakery'",
"'pub'",
"'hairdresser'",
"'fast_food'",
"'travel_agent'",
"'bakery'",
"'pub'",
"'florist'",
"'theatre'",
"'veterinary'",
"'market_place'",
"'park'",
"'nightclub'",
"'car_wash'",
"'school'",
"'car_dealership'",
"'restaurant'",
"'computer_shop'",
"'cafe'",
"'nightclub'",
"'bakery'",
"'restaurant'",
"'shelter'",
"'shelter'",
"'cafe'",
"'clinic'",
"'cafe'",
"'pub'",
"'bar'",
"'cafe'"],
["'clothes'",
"'school'",
"'pitch'",
"'park'",
"'playground'",
"'pitch'",
"'track'",
"'doctors'",
"'hospital'",
"'swimming_pool'",
"'water_works'",
"'hairdresser'",
"'fast_food'"],
["'pitch'", "'park'", "'park'", "'park'"],
["'park'",
"'college'",
"'playground'",
"'stadium'",
"'pitch'",
"'convenience'",
"'school'",
"'convenience'",
"'fast_food'",
"'pharmacy'",
"'fast_food'",
"'hairdresser'",
"'fast_food'",
"'pub'",
"'pub'"],
["'pub'",
"'supermarket'",
"'playground'",
"'doctors'",
"'pitch'",
"'pitch'",
"'school'",
"'kindergarten'",
"'pitch'",
"'park'"],
["'public_building'",
"'dentist'",
"'park'",
"'park'",
"'pitch'",
"'pitch'",
"'park'"],
["'park'"],
["'convenience'",
"'school'",
"'convenience'",
"'fast_food'",
"'nightclub'",
"'clinic'",
"'supermarket'",
"'pitch'",
"'pitch'",
"'pitch'",
"'playground'",
"'pitch'",
"'pitch'",
"'pitch'",
"'school'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'"],
["'sports_shop'",
"'pitch'",
"'pitch'",
"'pitch'",
"'school'",
"'hairdresser'",
"'guesthouse'",
"'hairdresser'",
"'fast_food'",
"'hairdresser'",
"'laundry'",
"'fast_food'",
"'fast_food'",
"'convenience'",
"'pub'",
"'guesthouse'",
"'car_dealership'",
"'fast_food'",
"'car_dealership'",
"'hairdresser'",
"'fast_food'",
"'supermarket'",
"'beauty_shop'",
"'hairdresser'",
"'hairdresser'",
"'fast_food'",
"'restaurant'",
"'hairdresser'",
"'hairdresser'",
"'restaurant'",
"'hairdresser'",
"'restaurant'",
"'beauty_shop'",
"'beauty_shop'",
"'fast_food'",
"'hairdresser'",
"'convenience'",
"'restaurant'",
"'convenience'",
"'pub'",
"'hairdresser'",
"'cafe'",
"'sports_shop'",
"'beauty_shop'"],
["'playground'",
"'playground'",
"'artwork'",
"'shelter'",
"'pitch'",
"'park'",
"'playground'",
"'convenience'",
"'bakery'",
"'fast_food'",
"'convenience'",
"'playground'",
"'pitch'",
"'greengrocer'",
"'pitch'",
"'newsagent'",
"'park'",
"'park'"],
["'toilet'",
"'university'",
"'park'",
"'museum'",
"'nursing_home'",
"'pitch'",
"'pitch'",
"'recycling_glass'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'town_hall'",
"'beauty_shop'",
"'bicycle_shop'",
"'beverages'",
"'nursing_home'"],
["'dentist'",
"'restaurant'",
"'pub'",
"'pub'",
"'kindergarten'",
"'pitch'",
"'pitch'",
"'library'",
"'pitch'",
"'graveyard'",
"'park'",
"'kindergarten'",
"'supermarket'",
"'bar'",
"'hairdresser'",
"'convenience'",
"'bakery'",
"'hairdresser'",
"'stationery'",
"'beauty_shop'",
"'fast_food'",
"'laundry'",
"'hairdresser'",
"'pharmacy'",
"'laundry'",
"'kindergarten'",
"'hairdresser'",
"'playground'"],
["'pitch'",
"'pitch'",
"'playground'",
"'cafe'",
"'pub'",
"'school'",
"'school'",
"'kindergarten'"],
["'cafe'",
"'graveyard'",
"'pub'",
"'beauty_shop'",
"'convenience'",
"'pub'",
"'kindergarten'",
"'pitch'"],
["'hairdresser'",
"'hairdresser'",
"'convenience'",
"'community_centre'",
"'school'"],
["'park'",
"'convenience'",
"'public_building'",
"'community_centre'",
"'public_building'",
"'park'",
"'convenience'",
"'fast_food'",
"'pub'",
"'park'",
"'clinic'",
"'park'",
"'library'",
"'park'",
"'community_centre'",
"'fast_food'",
"'supermarket'",
"'community_centre'",
"'restaurant'",
"'fast_food'",
"'cafe'",
"'hairdresser'",
"'cafe'",
"'pharmacy'",
"'hairdresser'",
"'hairdresser'",
"'hairdresser'",
"'beverages'",
"'supermarket'",
"'cafe'",
"'pharmacy'",
"'bakery'",
"'fast_food'",
"'fast_food'",
"'convenience'",
"'park'"],
["'pitch'",
"'school'",
"'pitch'",
"'pitch'",
"'playground'",
"'pitch'",
"'park'",
"'hospital'",
"'school'",
"'supermarket'"],
["'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'school'",
"'pitch'",
"'pitch'",
"'community_centre'"],
["'park'",
"'park'",
"'convenience'",
"'convenience'",
"'veterinary'",
"'veterinary'",
"'car_dealership'",
"'car_dealership'",
"'hairdresser'",
"'hairdresser'",
"'fast_food'",
"'cafe'",
"'convenience'",
"'convenience'",
"'library'"],
["'school'",
"'hairdresser'",
"'park'",
"'pitch'",
"'kindergarten'",
"'school'"],
["'playground'",
"'school'",
"'school'",
"'pitch'",
"'pitch'",
"'playground'",
"'park'"],
["'clinic'",
"'dentist'",
"'convenience'",
"'fast_food'",
"'bakery'",
"'fast_food'",
"'convenience'",
"'convenience'",
"'convenience'",
"'pharmacy'",
"'convenience'",
"'fast_food'",
"'park'",
"'fast_food'",
"'cafe'",
"'hairdresser'",
"'hairdresser'",
"'cafe'",
"'convenience'",
"'playground'",
"'community_centre'",
"'supermarket'",
"'pitch'",
"'school'",
"'pitch'",
"'school'",
"'graveyard'",
"'pitch'",
"'pitch'",
"'pitch'",
"'doctors'"],
["'pub'",
"'bakery'",
"'pub'",
"'pitch'",
"'park'",
"'playground'",
"'car_dealership'",
"'school'"],
["'library'",
"'hairdresser'",
"'fast_food'",
"'cafe'",
"'dentist'",
"'beauty_shop'",
"'restaurant'",
"'pitch'",
"'pitch'",
"'playground'"],
["'school'", "'track'", "'community_centre'", "'pub'"],
["'beauty_shop'",
"'pub'",
"'doityourself'",
"'pub'",
"'convenience'",
"'hairdresser'",
"'bank'",
"'pharmacy'",
"'fast_food'",
"'optician'",
"'butcher'",
"'cafe'",
"'florist'",
"'hairdresser'",
"'supermarket'",
"'hairdresser'",
"'hairdresser'",
"'convenience'",
"'fast_food'",
"'cafe'",
"'convenience'",
"'convenience'",
"'fast_food'",
"'kindergarten'",
"'hairdresser'",
"'hairdresser'",
"'playground'",
"'park'",
"'playground'",
"'dentist'",
"'post_office'",
"'cafe'",
"'cafe'",
"'veterinary'",
"'beverages'",
"'bakery'",
"'school'",
"'graveyard'",
"'convenience'"],
["'restaurant'",
"'clothes'",
"'hairdresser'",
"'clothes'",
"'pub'",
"'hairdresser'",
"'pub'",
"'restaurant'",
"'gift_shop'",
"'restaurant'",
"'convenience'",
"'restaurant'",
"'playground'",
"'restaurant'",
"'museum'",
"'attraction'",
"'fast_food'",
"'furniture_shop'",
"'fast_food'",
"'post_office'",
"'cafe'",
"'pub'",
"'fast_food'",
"'restaurant'",
"'restaurant'",
"'bakery'",
"'park'",
"'laundry'",
"'cafe'",
"'mall'",
"'cafe'",
"'park'"],
["'pub'",
"'library'",
"'school'",
"'school'",
"'pub'",
"'pub'",
"'park'",
"'school'",
"'school'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'playground'",
"'pitch'"],
["'pitch'",
"'pitch'",
"'school'",
"'pitch'",
"'pitch'",
"'water_works'",
"'park'",
"'playground'",
"'park'",
"'pub'",
"'community_centre'",
"'school'"],
["'beverages'",
"'restaurant'",
"'doityourself'",
"'hairdresser'",
"'clinic'",
"'kindergarten'",
"'florist'",
"'hairdresser'",
"'cafe'",
"'optician'",
"'cafe'",
"'cafe'",
"'bar'",
"'beauty_shop'",
"'hairdresser'",
"'bookshop'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'computer_shop'",
"'pharmacy'",
"'cafe'",
"'butcher'",
"'restaurant'",
"'bakery'",
"'fast_food'",
"'bakery'",
"'fast_food'",
"'beauty_shop'",
"'restaurant'",
"'greengrocer'",
"'convenience'",
"'hairdresser'",
"'newsagent'",
"'pub'",
"'bank'",
"'fast_food'",
"'supermarket'",
"'doityourself'",
"'stadium'"],
["'kindergarten'",
"'beauty_shop'",
"'clothes'",
"'toy_shop'",
"'hairdresser'",
"'supermarket'",
"'cafe'",
"'fast_food'",
"'gift_shop'",
"'pub'",
"'beverages'",
"'bookshop'",
"'post_office'",
"'toy_shop'",
"'supermarket'",
"'supermarket'",
"'gift_shop'",
"'beverages'",
"'bakery'",
"'hairdresser'",
"'greengrocer'",
"'gift_shop'",
"'mobile_phone_shop'",
"'bar'",
"'bicycle_shop'",
"'florist'",
"'hairdresser'",
"'beauty_shop'",
"'cafe'",
"'greengrocer'",
"'restaurant'",
"'pub'",
"'cafe'",
"'gift_shop'",
"'cafe'",
"'convenience'",
"'pub'",
"'bakery'",
"'newsagent'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'cafe'",
"'library'",
"'pub'"],
["'playground'",
"'sports_centre'",
"'park'",
"'park'",
"'community_centre'",
"'pub'",
"'pitch'",
"'park'",
"'playground'",
"'newsagent'",
"'fast_food'",
"'fast_food'",
"'pub'",
"'bar'"],
["'park'",
"'park'",
"'park'",
"'clinic'",
"'playground'",
"'clinic'",
"'pitch'",
"'pitch'",
"'park'"],
["'clinic'",
"'clinic'",
"'school'",
"'kindergarten'",
"'park'",
"'park'",
"'park'",
"'park'",
"'school'",
"'park'",
"'playground'",
"'community_centre'",
"'playground'",
"'playground'",
"'playground'",
"'community_centre'",
"'playground'",
"'pitch'",
"'pitch'",
"'pitch'",
"'park'",
"'kindergarten'"],
["'park'",
"'community_centre'",
"'school'",
"'playground'",
"'park'",
"'park'",
"'golf_course'"],
["'guesthouse'",
"'pub'",
"'sports_shop'",
"'pub'",
"'pub'",
"'college'",
"'dentist'",
"'ruins'",
"'school'",
"'convenience'",
"'cafe'",
"'school'",
"'clinic'",
"'sports_centre'"],
["'newsagent'",
"'fast_food'",
"'cafe'",
"'shoe_shop'",
"'florist'",
"'furniture_shop'",
"'cafe'",
"'hairdresser'",
"'fast_food'",
"'park'",
"'cafe'",
"'restaurant'",
"'optician'",
"'pharmacy'",
"'fast_food'",
"'school'",
"'supermarket'",
"'cafe'",
"'laundry'",
"'park'",
"'bar'",
"'graveyard'",
"'hairdresser'",
"'hairdresser'"],
["'dentist'",
"'doityourself'",
"'supermarket'",
"'car_wash'",
"'pitch'",
"'school'",
"'doctors'",
"'doctors'",
"'pub'",
"'florist'",
"'hospital'",
"'pitch'",
"'pitch'",
"'water_works'",
"'veterinary'",
"'community_centre'",
"'restaurant'",
"'beauty_shop'",
"'convenience'"],
["'pitch'",
"'playground'",
"'park'",
"'hairdresser'",
"'golf_course'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'sports_centre'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'"],
["'park'",
"'shelter'",
"'toilet'",
"'water_tower'",
"'water_works'",
"'cafe'",
"'park'",
"'park'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'toilet'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'swimming_pool'",
"'pitch'",
"'ruins'",
"'swimming_pool'",
"'swimming_pool'",
"'swimming_pool'",
"'doctors'",
"'nursing_home'",
"'swimming_pool'",
"'swimming_pool'",
"'college'"],
["'restaurant'",
"'toilet'",
"'florist'",
"'jeweller'",
"'laundry'",
"'jeweller'",
"'pub'",
"'playground'",
"'attraction'",
"'clinic'",
"'theatre'",
"'pitch'",
"'pitch'",
"'pitch'",
"'attraction'",
"'shelter'",
"'shelter'",
"'pitch'",
"'shelter'",
"'school'",
"'park'",
"'school'",
"'doctors'"],
["'fast_food'",
"'sports_centre'",
"'pitch'",
"'pub'",
"'park'",
"'school'",
"'arts_centre'",
"'supermarket'",
"'pub'",
"'hotel'",
"'guesthouse'",
"'graveyard'",
"'dentist'",
"'doctors'",
"'clinic'",
"'cafe'",
"'hairdresser'",
"'pub'",
"'pitch'",
"'pitch'",
"'playground'",
"'fast_food'",
"'car_wash'",
"'doctors'",
"'park'",
"'hairdresser'",
"'beverages'",
"'post_office'",
"'restaurant'",
"'hairdresser'",
"'bank'",
"'beauty_shop'",
"'hairdresser'",
"'hairdresser'",
"'butcher'",
"'pharmacy'",
"'hairdresser'",
"'bank'",
"'cafe'",
"'cafe'",
"'clothes'",
"'pub'",
"'hairdresser'",
"'mobile_phone_shop'",
"'greengrocer'",
"'fast_food'",
"'bakery'",
"'cafe'",
"'hairdresser'",
"'toy_shop'",
"'cafe'",
"'beauty_shop'",
"'convenience'",
"'fast_food'",
"'restaurant'",
"'hairdresser'",
"'community_centre'",
"'dentist'",
"'optician'",
"'newsagent'",
"'clothes'",
"'fast_food'",
"'doityourself'",
"'car_wash'",
"'car_wash'"],
["'park'",
"'school'",
"'swimming_pool'",
"'pitch'",
"'pitch'",
"'sports_centre'",
"'pitch'",
"'doctors'",
"'pitch'",
"'convenience'",
"'veterinary'",
"'pub'",
"'furniture_shop'",
"'pitch'",
"'school'",
"'pitch'",
"'pitch'",
"'pitch'",
"'park'",
"'pitch'",
"'school'",
"'sports_centre'",
"'park'",
"'school'"],
["'doityourself'",
"'playground'",
"'park'",
"'hairdresser'",
"'convenience'",
"'fast_food'",
"'fast_food'",
"'garden_centre'",
"'supermarket'",
"'fast_food'",
"'supermarket'",
"'fast_food'",
"'supermarket'",
"'fast_food'",
"'bakery'",
"'cafe'",
"'sports_shop'",
"'pharmacy'",
"'cinema'",
"'doityourself'",
"'doityourself'",
"'fast_food'",
"'doityourself'",
"'doityourself'",
"'park'",
"'park'",
"'kindergarten'",
"'bar'",
"'school'",
"'car_rental'",
"'comms_tower'",
"'car_dealership'",
"'police'",
"'doityourself'",
"'car_dealership'",
"'doityourself'",
"'playground'"],
["'school'",
"'school'",
"'pub'",
"'supermarket'",
"'beverages'",
"'fast_food'",
"'car_dealership'",
"'hairdresser'",
"'fast_food'",
"'kindergarten'"],
["'beauty_shop'",
"'hairdresser'",
"'clothes'",
"'restaurant'",
"'clothes'",
"'beauty_shop'",
"'pub'",
"'restaurant'",
"'clothes'",
"'clothes'",
"'jeweller'",
"'laundry'",
"'furniture_shop'",
"'pub'",
"'hairdresser'",
"'restaurant'",
"'bookshop'",
"'pub'",
"'restaurant'",
"'restaurant'",
"'florist'",
"'mobile_phone_shop'",
"'library'",
"'graveyard'",
"'pub'",
"'cafe'",
"'beverages'",
"'bookshop'",
"'clothes'",
"'bakery'",
"'fast_food'",
"'jeweller'",
"'pharmacy'",
"'clothes'",
"'fast_food'",
"'pub'",
"'dentist'",
"'hotel'",
"'cafe'",
"'park'",
"'pitch'",
"'graveyard'",
"'hairdresser'",
"'cafe'",
"'pharmacy'",
"'cafe'",
"'hospital'",
"'doityourself'",
"'fast_food'",
"'hairdresser'",
"'beauty_shop'",
"'pub'",
"'restaurant'",
"'restaurant'",
"'convenience'",
"'clinic'",
"'jeweller'",
"'hairdresser'",
"'restaurant'",
"'cafe'",
"'hairdresser'",
"'restaurant'",
"'car_dealership'",
"'supermarket'",
"'restaurant'",
"'pub'",
"'shoe_shop'",
"'laundry'",
"'optician'",
"'jeweller'",
"'bookshop'",
"'jeweller'",
"'gift_shop'",
"'computer_shop'",
"'travel_agent'",
"'florist'",
"'cafe'",
"'restaurant'",
"'butcher'",
"'restaurant'",
"'cafe'",
"'beauty_shop'",
"'clothes'",
"'jeweller'",
"'restaurant'",
"'clothes'",
"'jeweller'",
"'cafe'",
"'bar'",
"'clothes'",
"'gift_shop'",
"'clothes'",
"'restaurant'",
"'clothes'",
"'clothes'",
"'hotel'",
"'clothes'",
"'furniture_shop'",
"'hairdresser'",
"'pub'",
"'dentist'",
"'jeweller'",
"'restaurant'",
"'cafe'"],
["'library'",
"'park'",
"'playground'",
"'kindergarten'",
"'community_centre'",
"'track'",
"'beauty_shop'",
"'pitch'",
"'school'"],
["'graveyard'",
"'pitch'",
"'school'",
"'convenience'",
"'school'",
"'pitch'",
"'park'",
"'toilet'"],
["'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'playground'",
"'school'",
"'park'",
"'hospital'",
"'school'",
"'furniture_shop'",
"'school'",
"'pub'",
"'nursing_home'",
"'nursing_home'",
"'nursing_home'",
"'nursing_home'",
"'school'",
"'park'"],
["'park'",
"'playground'",
"'clothes'",
"'pub'",
"'car_wash'",
"'computer_shop'",
"'fast_food'",
"'convenience'",
"'clinic'",
"'car_dealership'",
"'cafe'",
"'convenience'",
"'hairdresser'",
"'florist'",
"'school'",
"'sports_shop'",
"'fast_food'",
"'fast_food'",
"'pub'",
"'pub'",
"'beauty_shop'",
"'car_dealership'"],
["'park'", "'school'"],
["'hairdresser'", "'park'", "'pitch'", "'school'", "'convenience'"],
["'sports_centre'",
"'pitch'",
"'pitch'",
"'doctors'",
"'park'",
"'cafe'",
"'convenience'",
"'playground'",
"'graveyard'",
"'park'"],
["'hairdresser'",
"'guesthouse'",
"'hairdresser'",
"'cafe'",
"'optician'",
"'hairdresser'",
"'pharmacy'",
"'optician'",
"'cafe'",
"'supermarket'",
"'dentist'",
"'stationery'",
"'cafe'",
"'greengrocer'",
"'hairdresser'",
"'laundry'",
"'bakery'",
"'beauty_shop'",
"'butcher'",
"'gift_shop'",
"'restaurant'",
"'school'",
"'community_centre'",
"'pitch'",
"'beverages'",
"'school'",
"'bar'",
"'pitch'",
"'pitch'",
"'school'",
"'pitch'",
"'convenience'",
"'garden_centre'",
"'fast_food'",
"'post_office'",
"'hairdresser'",
"'fast_food'",
"'hairdresser'",
"'doityourself'",
"'hairdresser'",
"'hairdresser'",
"'bicycle_shop'",
"'beauty_shop'",
"'pub'",
"'restaurant'",
"'hairdresser'",
"'playground'",
"'park'"],
["'archaeological'", "'swimming_pool'", "'park'", "'swimming_pool'"],
["'pub'",
"'park'",
"'park'",
"'pitch'",
"'track'",
"'playground'",
"'pitch'"],
["'community_centre'",
"'hairdresser'",
"'convenience'",
"'fast_food'",
"'restaurant'",
"'pub'",
"'beauty_shop'",
"'florist'",
"'convenience'",
"'beauty_shop'",
"'fast_food'",
"'dentist'",
"'cafe'",
"'hairdresser'",
"'supermarket'",
"'pitch'",
"'pitch'",
"'park'",
"'graveyard'",
"'pitch'",
"'school'",
"'playground'",
"'kindergarten'",
"'beauty_shop'",
"'pub'",
"'bicycle_shop'",
"'laundry'",
"'cafe'",
"'mobile_phone_shop'",
"'hairdresser'",
"'cafe'",
"'computer_shop'",
"'hairdresser'",
"'hairdresser'",
"'pub'",
"'car_dealership'",
"'park'",
"'furniture_shop'"],
["'park'",
"'pub'",
"'pub'",
"'hairdresser'",
"'hotel'",
"'restaurant'",
"'restaurant'",
"'pub'",
"'dentist'"],
["'department_store'",
"'pub'",
"'theatre'",
"'car_rental'",
"'doityourself'",
"'arts_centre'",
"'bar'",
"'supermarket'",
"'furniture_shop'",
"'furniture_shop'",
"'cafe'",
"'school'",
"'park'",
"'kindergarten'",
"'pub'",
"'park'",
"'restaurant'",
"'mall'",
"'hotel'",
"'bar'",
"'playground'",
"'hotel'",
"'pub'",
"'newsagent'",
"'pub'",
"'community_centre'",
"'cafe'",
"'furniture_shop'",
"'convenience'",
"'clothes'",
"'hairdresser'",
"'cafe'",
"'restaurant'",
"'computer_shop'",
"'restaurant'",
"'police'",
"'community_centre'",
"'park'",
"'bar'",
"'pitch'",
"'fast_food'",
"'florist'",
"'hairdresser'",
"'bakery'",
"'fast_food'",
"'hairdresser'",
"'school'"],
["'furniture_shop'",
"'fast_food'",
"'supermarket'",
"'beverages'",
"'pub'",
"'bookshop'",
"'restaurant'",
"'beauty_shop'",
"'convenience'",
"'bicycle_shop'",
"'pitch'",
"'school'"],
["'bank'",
"'clothes'",
"'cafe'",
"'clothes'",
"'clothes'",
"'clothes'",
"'bank'",
"'restaurant'",
"'optician'",
"'bank'",
"'mobile_phone_shop'",
"'cafe'",
"'pharmacy'",
"'clothes'",
"'restaurant'",
"'travel_agent'",
"'shoe_shop'",
"'restaurant'",
"'cafe'",
"'bank'",
"'video_shop'",
"'clothes'",
"'toy_shop'",
"'fast_food'",
"'beauty_shop'",
"'cafe'",
"'mall'",
"'fast_food'",
"'clothes'",
"'cafe'",
"'fast_food'",
"'supermarket'",
"'mall'",
"'fast_food'",
"'nightclub'",
"'mall'",
"'jeweller'",
"'cinema'",
"'hairdresser'",
"'beauty_shop'",
"'jeweller'",
"'gift_shop'",
"'mall'",
"'cafe'",
"'hairdresser'",
"'jeweller'",
"'bakery'",
"'fast_food'",
"'cafe'",
"'shoe_shop'",
"'school'",
"'outdoor_shop'",
"'gift_shop'",
"'shoe_shop'",
"'shoe_shop'",
"'travel_agent'",
"'hostel'",
"'sports_centre'",
"'bicycle_shop'",
"'mobile_phone_shop'",
"'mall'",
"'beauty_shop'",
"'gift_shop'",
"'outdoor_shop'",
"'pharmacy'",
"'doctors'",
"'fast_food'",
"'mobile_phone_shop'",
"'clothes'",
"'convenience'",
"'community_centre'",
"'mobile_phone_shop'",
"'bank'",
"'fast_food'",
"'mobile_phone_shop'",
"'cafe'",
"'cafe'",
"'bakery'",
"'mobile_phone_shop'",
"'clothes'",
"'mobile_phone_shop'",
"'restaurant'",
"'bar'",
"'restaurant'",
"'hairdresser'",
"'restaurant'",
"'bar'",
"'pub'",
"'pub'",
"'cafe'",
"'pub'",
"'restaurant'",
"'clothes'",
"'cafe'",
"'travel_agent'",
"'cafe'",
"'pub'",
"'hairdresser'",
"'shoe_shop'",
"'hairdresser'",
"'cafe'",
"'cinema'",
"'video_shop'",
"'clothes'",
"'beverages'",
"'hairdresser'",
"'pub'",
"'fast_food'",
"'bar'",
"'pub'",
"'bicycle_shop'",
"'hairdresser'",
"'fast_food'",
"'pub'",
"'clothes'",
"'bar'",
"'restaurant'",
"'pub'",
"'pub'",
"'bar'",
"'gift_shop'",
"'gift_shop'",
"'mobile_phone_shop'",
"'bar'",
"'guesthouse'",
"'cafe'",
"'cafe'",
"'bookshop'",
"'bar'",
"'gift_shop'",
"'bookshop'",
"'jeweller'",
"'mall'",
"'restaurant'",
"'bookshop'",
"'pub'",
"'bar'",
"'cafe'",
"'food_court'",
"'market_place'",
"'travel_agent'",
"'mall'",
"'fast_food'",
"'hotel'",
"'clothes'",
"'cafe'",
"'ruins'",
"'travel_agent'",
"'bar'",
"'clothes'",
"'hotel'",
"'bakery'",
"'cafe'",
"'hairdresser'",
"'clothes'",
"'cafe'",
"'ruins'",
"'pitch'",
"'convenience'",
"'hotel'",
"'cafe'",
"'bank'",
"'hairdresser'",
"'toy_shop'",
"'pub'",
"'cafe'",
"'beauty_shop'",
"'mobile_phone_shop'",
"'hairdresser'",
"'bicycle_shop'",
"'clinic'",
"'restaurant'",
"'clothes'",
"'bookshop'",
"'mall'",
"'observation_tower'",
"'observation_tower'",
"'hairdresser'",
"'fast_food'",
"'pub'",
"'park'",
"'fast_food'",
"'fast_food'",
"'hotel'",
"'bank'",
"'clothes'",
"'cafe'",
"'clothes'",
"'clothes'",
"'clothes'",
"'clothes'",
"'clothes'",
"'clothes'",
"'shoe_shop'",
"'cafe'",
"'hairdresser'",
"'convenience'",
"'nightclub'",
"'restaurant'",
"'fast_food'",
"'optician'",
"'restaurant'",
"'fast_food'",
"'cafe'",
"'restaurant'",
"'restaurant'",
"'bar'",
"'bar'",
"'hairdresser'",
"'cafe'",
"'cafe'",
"'restaurant'",
"'dentist'",
"'beauty_shop'",
"'restaurant'",
"'clinic'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'bar'",
"'restaurant'",
"'restaurant'",
"'bookshop'",
"'bar'",
"'bar'",
"'cafe'",
"'hostel'",
"'bar'",
"'cafe'",
"'pub'",
"'bar'",
"'arts_centre'",
"'bar'",
"'cafe'",
"'courthouse'",
"'monument'",
"'cafe'",
"'bar'",
"'pub'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'bar'",
"'hotel'",
"'fast_food'",
"'hotel'",
"'convenience'",
"'restaurant'",
"'restaurant'",
"'fast_food'",
"'restaurant'",
"'newsagent'",
"'restaurant'",
"'nightclub'",
"'restaurant'",
"'restaurant'",
"'cafe'",
"'florist'",
"'hairdresser'",
"'restaurant'",
"'fast_food'",
"'beauty_shop'",
"'hotel'",
"'pub'",
"'clinic'",
"'pub'",
"'park'",
"'nightclub'",
"'attraction'",
"'restaurant'",
"'restaurant'",
"'pub'",
"'cafe'",
"'dentist'",
"'restaurant'",
"'cafe'",
"'doctors'",
"'sports_centre'",
"'restaurant'",
"'hotel'",
"'kindergarten'",
"'pub'",
"'pub'",
"'bar'",
"'pub'",
"'pub'",
"'restaurant'",
"'theatre'",
"'doctors'",
"'convenience'",
"'restaurant'",
"'memorial'",
"'fast_food'",
"'hotel'",
"'convenience'",
"'fast_food'",
"'restaurant'",
"'hairdresser'",
"'restaurant'",
"'travel_agent'",
"'hairdresser'",
"'bakery'",
"'pub'",
"'fast_food'",
"'restaurant'",
"'hairdresser'",
"'fast_food'",
"'hairdresser'",
"'fast_food'",
"'fast_food'",
"'jeweller'",
"'clothes'",
"'cafe'",
"'restaurant'",
"'restaurant'",
"'fast_food'",
"'restaurant'",
"'bicycle_shop'",
"'clothes'",
"'bakery'",
"'beverages'",
"'fast_food'",
"'butcher'",
"'fast_food'",
"'museum'",
"'attraction'",
"'attraction'",
"'attraction'",
"'cafe'",
"'bicycle_shop'",
"'arts_centre'",
"'bank'",
"'pub'",
"'restaurant'",
"'restaurant'",
"'nightclub'",
"'hostel'",
"'cafe'",
"'convenience'",
"'cafe'",
"'pub'",
"'hotel'",
"'restaurant'",
"'bar'",
"'park'",
"'college'",
"'pub'",
"'dentist'",
"'college'",
"'pub'",
"'pub'",
"'pub'",
"'pub'",
"'restaurant'",
"'pub'",
"'ruins'",
"'park'",
"'attraction'",
"'gift_shop'",
"'pub'",
"'bookshop'",
"'beauty_shop'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'playground'",
"'pitch'",
"'pub'"],
["'doityourself'",
"'pub'",
"'doityourself'",
"'supermarket'",
"'beauty_shop'",
"'restaurant'",
"'beauty_shop'",
"'computer_shop'",
"'cafe'",
"'restaurant'",
"'optician'",
"'car_dealership'",
"'convenience'",
"'doityourself'"],
["'park'",
"'pub'",
"'cafe'",
"'museum'",
"'cafe'",
"'cafe'",
"'museum'",
"'tourist_info'",
"'attraction'",
"'sports_centre'",
"'attraction'",
"'sports_centre'",
"'museum'",
"'convenience'",
"'museum'",
"'pub'",
"'caravan_site'",
"'arts_centre'",
"'arts_centre'",
"'pub'",
"'restaurant'",
"'toilet'",
"'attraction'",
"'bicycle_shop'",
"'attraction'",
"'restaurant'",
"'park'",
"'bicycle_shop'",
"'sports_centre'"],
["'pub'", "'school'"],
["'park'",
"'fast_food'",
"'hairdresser'",
"'cafe'",
"'post_office'",
"'convenience'",
"'convenience'",
"'park'",
"'park'",
"'park'"],
["'hairdresser'",
"'convenience'",
"'hotel'",
"'beauty_shop'",
"'pub'",
"'kindergarten'"],
["'shelter'",
"'park'",
"'cafe'",
"'cafe'",
"'fast_food'",
"'community_centre'",
"'playground'",
"'park'",
"'fire_station'",
"'clinic'",
"'fast_food'",
"'fast_food'",
"'track'",
"'fire_station'",
"'tower'",
"'school'",
"'park'",
"'laundry'",
"'hotel'",
"'hairdresser'",
"'pub'",
"'pub'",
"'doctors'",
"'supermarket'",
"'sports_shop'",
"'hairdresser'",
"'fast_food'",
"'pharmacy'",
"'bakery'",
"'fast_food'",
"'guesthouse'",
"'fast_food'",
"'library'",
"'hotel'",
"'playground'"],
["'park'",
"'convenience'",
"'pitch'",
"'guesthouse'",
"'pitch'",
"'hairdresser'",
"'restaurant'",
"'playground'",
"'graveyard'",
"'pitch'",
"'graveyard'",
"'park'"],
["'car_dealership'",
"'pharmacy'",
"'clothes'",
"'clothes'",
"'supermarket'",
"'fast_food'",
"'doityourself'",
"'furniture_shop'",
"'supermarket'",
"'sports_shop'",
"'park'",
"'park'",
"'school'",
"'pub'",
"'car_dealership'"],
["'bank'",
"'university'",
"'theatre'",
"'hotel'",
"'veterinary'",
"'clinic'",
"'pub'",
"'kindergarten'",
"'swimming_pool'",
"'attraction'",
"'kindergarten'",
"'water_works'",
"'cafe'",
"'mobile_phone_shop'",
"'pharmacy'",
"'supermarket'",
"'mall'",
"'clinic'",
"'fast_food'",
"'laundry'",
"'optician'",
"'gift_shop'",
"'florist'",
"'fast_food'",
"'newsagent'",
"'mobile_phone_shop'",
"'cafe'",
"'cafe'",
"'clinic'",
"'clinic'",
"'doityourself'",
"'clinic'",
"'dentist'",
"'clinic'",
"'supermarket'",
"'cinema'",
"'butcher'",
"'restaurant'",
"'garden_centre'"],
["'school'", "'playground'"],
["'park'", "'picnic_site'", "'pitch'", "'pitch'", "'playground'"],
["'pub'",
"'hospital'",
"'hospital'",
"'hospital'",
"'hospital'",
"'hospital'",
"'pub'",
"'clinic'",
"'university'",
"'beauty_shop'",
"'cafe'",
"'cafe'",
"'school'",
"'bar'",
"'fast_food'",
"'park'",
"'university'",
"'arts_centre'",
"'college'",
"'tower'",
"'school'",
"'wastewater_plant'",
"'bank'",
"'hotel'",
"'park'",
"'park'",
"'hostel'",
"'pub'",
"'fast_food'",
"'bar'",
"'cafe'",
"'fast_food'",
"'fast_food'",
"'cafe'",
"'gift_shop'",
"'hairdresser'",
"'tower'",
"'fast_food'",
"'clothes'",
"'stationery'",
"'cafe'",
"'bar'",
"'post_office'",
"'cafe'",
"'clothes'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'museum'",
"'nightclub'",
"'bank'",
"'restaurant'",
"'hairdresser'",
"'bar'",
"'stationery'",
"'clothes'",
"'bar'",
"'cafe'",
"'optician'",
"'hairdresser'",
"'museum'",
"'bank'",
"'supermarket'",
"'mobile_phone_shop'",
"'outdoor_shop'",
"'clothes'",
"'nightclub'",
"'pharmacy'",
"'hostel'",
"'fast_food'",
"'fast_food'",
"'university'",
"'nightclub'",
"'restaurant'",
"'sports_centre'",
"'bank'",
"'cafe'",
"'bicycle_shop'",
"'university'",
"'food_court'",
"'fast_food'",
"'car_rental'",
"'police'",
"'pub'",
"'hairdresser'",
"'restaurant'",
"'beauty_shop'",
"'restaurant'",
"'nightclub'",
"'cafe'",
"'hairdresser'",
"'cafe'",
"'pub'",
"'theatre'",
"'clinic'",
"'hairdresser'",
"'hotel'",
"'hairdresser'",
"'hairdresser'",
"'pub'",
"'clinic'",
"'cafe'",
"'hairdresser'",
"'hairdresser'",
"'stationery'",
"'bookshop'",
"'park'",
"'hairdresser'",
"'convenience'",
"'florist'",
"'doityourself'",
"'school'",
"'arts_centre'",
"'florist'",
"'hotel'",
"'fast_food'",
"'cafe'",
"'bicycle_shop'",
"'restaurant'",
"'pub'",
"'cafe'",
"'clothes'",
"'bicycle_shop'",
"'jeweller'",
"'restaurant'",
"'hostel'",
"'sports_shop'",
"'cafe'",
"'guesthouse'",
"'pub'",
"'pub'",
"'pub'",
"'outdoor_shop'",
"'clothes'",
"'bar'",
"'restaurant'",
"'restaurant'",
"'cafe'",
"'bar'",
"'nightclub'",
"'gift_shop'",
"'cafe'",
"'bar'",
"'supermarket'",
"'clothes'",
"'clothes'",
"'restaurant'",
"'restaurant'",
"'bookshop'",
"'stationery'",
"'restaurant'",
"'restaurant'",
"'bar'",
"'fast_food'",
"'bar'",
"'optician'",
"'department_store'",
"'clothes'",
"'car_wash'",
"'restaurant'",
"'bar'",
"'convenience'",
"'cafe'",
"'hairdresser'",
"'theatre'",
"'museum'",
"'bar'",
"'restaurant'",
"'hairdresser'",
"'cafe'",
"'pub'",
"'restaurant'",
"'fast_food'",
"'bar'",
"'clothes'",
"'bakery'",
"'clothes'",
"'fast_food'",
"'cafe'",
"'clothes'",
"'bar'",
"'bar'",
"'dentist'",
"'clothes'",
"'cafe'",
"'restaurant'",
"'optician'",
"'newsagent'",
"'mobile_phone_shop'",
"'bookshop'",
"'cafe'",
"'cafe'",
"'bar'",
"'pub'",
"'restaurant'",
"'clothes'",
"'beauty_shop'",
"'cafe'",
"'cafe'",
"'beauty_shop'",
"'beverages'",
"'clothes'",
"'arts_centre'",
"'nightclub'",
"'arts_centre'",
"'hairdresser'",
"'hairdresser'",
"'travel_agent'",
"'cafe'",
"'fast_food'",
"'nightclub'"],
["'school'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'hairdresser'",
"'supermarket'",
"'doityourself'",
"'car_dealership'"],
["'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'graveyard'",
"'school'",
"'golf_course'",
"'park'"],
["'guesthouse'",
"'pub'",
"'convenience'",
"'garden_centre'",
"'park'",
"'pitch'",
"'park'",
"'water_works'",
"'pitch'",
"'doityourself'",
"'newsagent'",
"'cafe'",
"'greengrocer'",
"'cafe'",
"'fast_food'",
"'restaurant'",
"'community_centre'",
"'car_dealership'",
"'bakery'",
"'pub'",
"'fast_food'",
"'beauty_shop'",
"'pub'",
"'stationery'",
"'cafe'",
"'bakery'",
"'computer_shop'",
"'sports_centre'",
"'pitch'",
"'restaurant'",
"'pitch'",
"'school'",
"'pitch'",
"'school'",
"'pitch'",
"'pitch'",
"'pitch'",
"'stadium'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'school'",
"'pitch'",
"'pub'",
"'park'",
"'pitch'",
"'track'",
"'college'",
"'pitch'",
"'park'",
"'university'",
"'playground'",
"'university'",
"'pitch'",
"'park'",
"'theme_park'",
"'playground'",
"'kindergarten'"],
["'school'",
"'nursing_home'",
"'park'",
"'park'",
"'clinic'",
"'dentist'",
"'convenience'",
"'laundry'"],
["'school'",
"'track'",
"'playground'",
"'golf_course'",
"'school'",
"'park'"],
["'convenience'",
"'car_dealership'",
"'car_dealership'",
"'car_dealership'",
"'car_dealership'",
"'school'",
"'park'",
"'hairdresser'",
"'fast_food'",
"'car_dealership'",
"'school'",
"'pharmacy'"],
["'outdoor_shop'",
"'hospital'",
"'optician'",
"'convenience'",
"'park'",
"'guesthouse'",
"'pub'",
"'guesthouse'",
"'guesthouse'"],
["'school'", "'school'", "'school'"],
["'swimming_pool'",
"'park'",
"'swimming_pool'",
"'swimming_pool'",
"'supermarket'",
"'hairdresser'",
"'hairdresser'",
"'supermarket'",
"'restaurant'",
"'sports_centre'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'beauty_shop'",
"'veterinary'",
"'dentist'",
"'pub'",
"'swimming_pool'",
"'school'",
"'pitch'",
"'pitch'",
"'park'",
"'pitch'"],
["'school'",
"'laundry'",
"'bar'",
"'hairdresser'",
"'pub'",
"'car_dealership'",
"'hairdresser'",
"'car_wash'",
"'car_dealership'",
"'car_dealership'",
"'car_dealership'",
"'car_dealership'",
"'pub'",
"'cafe'",
"'hairdresser'",
"'hairdresser'",
"'fast_food'",
"'playground'"],
["'pub'",
"'school'",
"'sports_centre'",
"'community_centre'",
"'school'",
"'pub'",
"'hotel'",
"'supermarket'",
"'veterinary'",
"'playground'",
"'pitch'",
"'school'",
"'pitch'",
"'school'",
"'park'",
"'castle'",
"'graveyard'",
"'cafe'",
"'toilet'",
"'playground'",
"'pitch'",
"'playground'",
"'museum'",
"'park'"],
["'hairdresser'",
"'travel_agent'",
"'convenience'",
"'fast_food'",
"'car_dealership'",
"'clinic'",
"'pitch'",
"'cafe'",
"'monument'",
"'playground'",
"'park'",
"'graveyard'",
"'pitch'",
"'school'",
"'convenience'",
"'cafe'",
"'butcher'",
"'fast_food'",
"'butcher'",
"'butcher'",
"'supermarket'",
"'beverages'",
"'pitch'",
"'restaurant'",
"'clothes'"],
["'school'", "'park'", "'fast_food'", "'park'"],
["'furniture_shop'",
"'cafe'",
"'hairdresser'",
"'hairdresser'",
"'cafe'",
"'sports_shop'",
"'restaurant'",
"'beauty_shop'",
"'beverages'",
"'veterinary'",
"'restaurant'",
"'pub'",
"'fast_food'",
"'fast_food'",
"'restaurant'",
"'convenience'",
"'clothes'",
"'convenience'",
"'hairdresser'",
"'hairdresser'",
"'dentist'",
"'supermarket'",
"'hairdresser'",
"'restaurant'",
"'cafe'",
"'cafe'",
"'hairdresser'",
"'restaurant'",
"'restaurant'",
"'clinic'",
"'butcher'",
"'jeweller'",
"'hairdresser'",
"'fast_food'",
"'clinic'",
"'restaurant'",
"'cafe'",
"'clothes'",
"'restaurant'",
"'fast_food'",
"'fast_food'",
"'cafe'",
"'beauty_shop'",
"'hairdresser'",
"'pub'",
"'computer_shop'",
"'supermarket'",
"'convenience'",
"'hairdresser'",
"'beauty_shop'",
"'cafe'",
"'hairdresser'",
"'fast_food'",
"'cafe'",
"'sports_centre'",
"'cafe'",
"'supermarket'",
"'doityourself'",
"'restaurant'",
"'restaurant'",
"'mobile_phone_shop'",
"'cafe'",
"'hairdresser'",
"'hairdresser'",
"'cafe'",
"'fast_food'",
"'furniture_shop'",
"'shelter'",
"'school'",
"'prison'",
"'pitch'",
"'pitch'",
"'sports_centre'",
"'pitch'",
"'park'"],
["'park'",
"'supermarket'",
"'pitch'",
"'pitch'",
"'pitch'",
"'sports_centre'",
"'pitch'",
"'pitch'"],
["'hospital'",
"'sports_centre'",
"'pitch'",
"'cinema'",
"'playground'",
"'pitch'",
"'park'",
"'pitch'",
"'restaurant'",
"'fast_food'",
"'restaurant'",
"'fast_food'",
"'pub'",
"'hotel'",
"'sports_centre'",
"'park'",
"'track'",
"'school'"],
["'doctors'", "'cafe'", "'playground'"],
["'park'",
"'restaurant'",
"'hairdresser'",
"'beauty_shop'",
"'fast_food'",
"'pub'",
"'hairdresser'",
"'hairdresser'",
"'hairdresser'",
"'school'"],
["'community_centre'",
"'hairdresser'",
"'hairdresser'",
"'school'",
"'dentist'",
"'fast_food'",
"'car_wash'",
"'restaurant'",
"'dentist'",
"'post_office'",
"'doctors'",
"'pitch'",
"'pitch'",
"'pitch'",
"'sports_centre'",
"'fast_food'",
"'newsagent'",
"'convenience'"],
["'comms_tower'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'convenience'",
"'school'",
"'fast_food'",
"'playground'",
"'community_centre'",
"'park'",
"'attraction'"],
["'playground'",
"'pitch'",
"'fast_food'",
"'hospital'",
"'supermarket'",
"'fast_food'",
"'doityourself'",
"'fast_food'",
"'newsagent'",
"'supermarket'",
"'doityourself'",
"'dentist'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'golf_course'",
"'school'"],
["'park'",
"'kindergarten'",
"'cafe'",
"'restaurant'",
"'bakery'",
"'hairdresser'",
"'hairdresser'",
"'community_centre'",
"'clinic'",
"'fast_food'",
"'fast_food'",
"'sports_centre'",
"'clinic'",
"'hairdresser'",
"'convenience'",
"'pharmacy'",
"'cafe'",
"'pub'",
"'fast_food'",
"'travel_agent'",
"'convenience'",
"'nursing_home'"],
["'community_centre'",
"'school'",
"'school'",
"'pub'",
"'fast_food'",
"'doityourself'",
"'doityourself'",
"'hairdresser'",
"'playground'",
"'school'",
"'prison'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'sports_centre'",
"'pitch'",
"'cafe'",
"'park'"],
["'playground'",
"'pitch'",
"'school'",
"'school'",
"'school'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'"],
["'park'",
"'park'",
"'park'",
"'playground'",
"'convenience'",
"'fast_food'",
"'school'",
"'park'",
"'school'"],
["'dentist'",
"'college'",
"'kindergarten'",
"'car_dealership'",
"'community_centre'",
"'clinic'",
"'pub'",
"'hairdresser'",
"'furniture_shop'",
"'hairdresser'",
"'furniture_shop'",
"'clinic'",
"'clothes'",
"'restaurant'",
"'clinic'",
"'beauty_shop'",
"'optician'",
"'dentist'",
"'beauty_shop'",
"'cafe'",
"'convenience'",
"'cafe'",
"'supermarket'",
"'mall'",
"'pitch'",
"'pub'",
"'pub'",
"'sports_centre'"],
["'hairdresser'", "'cafe'", "'fast_food'", "'fast_food'", "'clinic'"],
["'dentist'",
"'clothes'",
"'fast_food'",
"'pub'",
"'car_dealership'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'cafe'",
"'fast_food'",
"'hairdresser'",
"'convenience'",
"'fast_food'",
"'convenience'",
"'convenience'",
"'sports_shop'",
"'park'",
"'car_wash'"],
["'gift_shop'",
"'toy_shop'",
"'convenience'",
"'beauty_shop'",
"'greengrocer'",
"'doctors'",
"'clinic'",
"'pub'",
"'clinic'",
"'doityourself'",
"'computer_shop'",
"'beauty_shop'",
"'hairdresser'",
"'pub'",
"'hairdresser'",
"'cafe'",
"'supermarket'",
"'computer_shop'",
"'cafe'",
"'bar'",
"'bank'",
"'cafe'",
"'fast_food'",
"'hairdresser'",
"'optician'",
"'bank'",
"'bakery'",
"'supermarket'",
"'sports_centre'",
"'bank'",
"'doityourself'",
"'bank'",
"'hairdresser'",
"'optician'",
"'bank'",
"'dentist'",
"'pharmacy'",
"'hairdresser'",
"'doctors'",
"'jeweller'",
"'shoe_shop'",
"'beauty_shop'",
"'newsagent'",
"'pub'",
"'pub'",
"'fast_food'",
"'restaurant'",
"'laundry'",
"'convenience'",
"'clinic'",
"'restaurant'",
"'cafe'",
"'hairdresser'",
"'fast_food'",
"'beauty_shop'",
"'hairdresser'",
"'florist'",
"'restaurant'",
"'supermarket'"],
["'car_dealership'",
"'pitch'",
"'pitch'",
"'pitch'",
"'pitch'",
"'school'",
"'pitch'",
"'pitch'",
"'shelter'",
"'museum'",
"'kindergarten'",
"'school'",
"'clinic'",
"'doctors'"],
["'park'", "'park'", "'swimming_pool'", "'college'"],
["'park'",
"'clinic'",
"'hairdresser'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'convenience'",
"'hairdresser'",
"'park'",
"'golf_course'"],
["'park'",
"'convenience'",
"'fast_food'",
"'convenience'",
"'community_centre'",
"'park'",
"'park'",
"'convenience'",
"'park'",
"'fast_food'"],
["'community_centre'", "'school'", "'doctors'", "'pub'", "'school'"],
["'park'", "'newsagent'", "'fast_food'", "'convenience'", "'park'"],
["'hairdresser'",
"'water_works'",
"'convenience'",
"'beauty_shop'",
"'bar'",
"'kindergarten'",
"'pub'",
"'park'",
"'car_dealership'",
"'supermarket'",
"'restaurant'",
"'fast_food'",
"'fast_food'",
"'newsagent'",
"'park'",
"'park'",
"'park'",
"'park'"],
["'pitch'",
"'park'",
"'school'",
"'arts_centre'",
"'guesthouse'",
"'pub'",
"'pub'",
"'car_dealership'",
"'pharmacy'",
"'laundry'",
"'convenience'",
"'hairdresser'",
"'beverages'",
"'fast_food'",
"'cafe'",
"'arts_centre'",
"'clothes'",
"'hairdresser'",
"'hairdresser'",
"'newsagent'",
"'cafe'",
"'fast_food'",
"'cafe'",
"'restaurant'",
"'toy_shop'",
"'cafe'",
"'cafe'",
"'playground'",
"'school'",
"'community_centre'"],
["'playground'",
"'car_dealership'",
"'playground'",
"'convenience'",
"'clothes'",
"'recycling_glass'",
"'park'",
"'stationery'",
"'playground'",
"'park'",
"'doctors'",
"'pharmacy'",
"'park'",
"'pub'",
"'playground'",
"'school'"],
["'butcher'",
"'hairdresser'",
"'fast_food'",
"'fast_food'",
"'convenience'",
"'pharmacy'",
"'park'",
"'playground'",
"'pitch'",
"'school'",
"'hairdresser'",
"'convenience'"],
["'beauty_shop'",
"'restaurant'",
"'newsagent'",
"'car_wash'",
"'cafe'",
"'hairdresser'",
"'fast_food'"],
["'cinema'",
"'restaurant'",
"'kindergarten'",
"'guesthouse'",
"'hotel'",
"'park'",
"'school'",
"'park'",
"'kindergarten'",
"'restaurant'",
"'kindergarten'",
"'hairdresser'",
"'hairdresser'",
"'beauty_shop'",
"'shelter'",
"'clothes'",
"'dentist'",
"'sports_shop'",
"'cafe'",
"'dentist'",
"'restaurant'",
"'veterinary'",
"'pharmacy'",
"'butcher'",
"'travel_agent'",
"'dentist'",
"'bookshop'",
"'hairdresser'",
"'bookshop'",
"'supermarket'"],
["'library'",
"'university'",
"'clinic'",
"'restaurant'",
"'fast_food'",
"'pub'",
"'bakery'",
"'kindergarten'",
"'school'",
"'arts_centre'",
"'cafe'",
"'school'",
"'sports_centre'",
"'university'",
"'hotel'",
"'kindergarten'",
"'cafe'",
"'university'",
"'university'",
"'cafe'",
"'university'",
"'pharmacy'",
"'hairdresser'",
"'restaurant'",
"'hairdresser'",
"'hairdresser'",
"'stationery'",
"'pub'",
"'hairdresser'",
"'pub'",
"'supermarket'",
"'pub'",
"'cafe'",
"'bar'",
"'furniture_shop'",
"'clinic'",
"'sports_shop'",
"'clinic'",
"'doctors'",
"'pub'",
"'sports_centre'",
"'university'"],
["'park'",
"'school'",
"'doityourself'",
"'doityourself'",
"'beverages'",
"'hairdresser'",
"'fast_food'",
"'fast_food'",
"'hairdresser'",
"'pharmacy'",
"'school'",
"'pitch'"],
["'hospital'",
"'playground'",
"'mobile_phone_shop'",
"'clothes'",
"'clothes'",
"'clothes'",
"'outdoor_shop'",
"'clothes'",
"'beauty_shop'",
"'mobile_phone_shop'",
"'beauty_shop'",
"'clothes'",
"'hairdresser'",
"'fast_food'",
"'outdoor_shop'",
"'bookshop'",
"'clinic'",
"'fast_food'",
"'bookshop'",
"'clothes'",
"'newsagent'",
"'mobile_phone_shop'",
"'clothes'",
"'beauty_shop'",
"'clothes'",
"'hairdresser'",
"'fast_food'",
"'fast_food'",
"'fast_food'",
"'beauty_shop'",
"'bank'",
"'hairdresser'",
"'cafe'",
"'fast_food'",
"'fast_food'",
"'beauty_shop'",
"'furniture_shop'",
"'department_store'",
"'park'",
"'hairdresser'",
"'cafe'",
"'park'",
"'clothes'",
"'fast_food'",
"'bakery'",
"'theme_park'",
"'cafe'",
"'cafe'",
"'bakery'",
"'fast_food'",
"'cafe'",
"'mobile_phone_shop'",
"'hairdresser'",
"'bicycle_shop'",
"'beauty_shop'",
"'hairdresser'",
"'fast_food'",
"'hotel'",
"'cafe'",
"'restaurant'",
"'cafe'",
"'courthouse'",
"'clothes'",
"'convenience'",
"'fast_food'",
"'fast_food'",
"'hairdresser'",
"'furniture_shop'",
"'mobile_phone_shop'",
"'cinema'",
"'mall'",
"'park'",
"'clothes'",
"'clothes'",
"'department_store'",
"'clothes'",
"'clothes'",
"'clothes'",
"'clothes'",
"'restaurant'",
"'clothes'",
"'clothes'",
"'restaurant'",
"'clothes'",
"'clothes'",
"'bank'",
"'hairdresser'",
"'gift_shop'",
"'sports_shop'",
"'bank'",
"'optician'",
"'toy_shop'",
"'mobile_phone_shop'",
"'convenience'",
"'video_shop'",
"'clothes'",
"'fast_food'",
"'fast_food'",
"'mall'",
"'cafe'",
"'shoe_shop'",
"'clothes'",
"'outdoor_shop'",
"'shoe_shop'",
"'bank'",
"'gift_shop'",
"'fast_food'",
"'shoe_shop'",
"'fast_food'",
"'beauty_shop'",
"'cafe'",
"'shoe_shop'",
"'convenience'",
"'mobile_phone_shop'",
"'convenience'",
"'mobile_phone_shop'",
"'mobile_phone_shop'",
"'cafe'",
"'cafe'",
"'beauty_shop'",
"'mobile_phone_shop'",
"'clothes'",
"'jeweller'",
"'mobile_phone_shop'",
"'travel_agent'",
"'restaurant'"],
["'bar'",
"'restaurant'",
"'hairdresser'",
"'clinic'",
"'restaurant'",
"'fast_food'",
"'fast_food'",
"'travel_agent'",
"'fast_food'",
"'restaurant'",
"'fast_food'",
"'clothes'",
"'hairdresser'",
"'furniture_shop'",
"'cafe'",
"'school'",
"'university'",
"'park'",
"'hotel'",
"'theatre'",
"'nightclub'",
"'cafe'",
"'clinic'",
"'supermarket'",
"'bar'",
"'restaurant'",
"'hotel'",
"'cafe'",
"'hotel'",
"'hairdresser'",
"'school'",
"'college'",
"'bookshop'",
"'pub'",
"'bicycle_shop'",
"'school'",
"'hairdresser'",
"'pub'",
"'fast_food'",
"'pub'",
"'cafe'",
"'school'",
"'convenience'",
"'clothes'",
"'school'",
"'library'",
"'school'",
"'bank'",
"'pitch'",
"'playground'",
"'pub'",
"'cafe'",
"'park'",
"'cafe'",
"'park'",
"'toilet'",
"'jeweller'",
"'museum'",
"'clothes'",
"'fast_food'",
"'attraction'",
"'sports_centre'",
"'cafe'",
"'pub'",
"'restaurant'",
"'restaurant'",
"'attraction'",
"'museum'",
"'pub'"],
["'shelter'",
"'playground'",
"'school'",
"'graveyard'",
"'doctors'",
"'pitch'",
"'pitch'",
"'sports_centre'",
"'park'",
"'playground'",
"'pub'"],
["'clothes'",
"'convenience'",
"'pharmacy'",
"'cafe'",
"'restaurant'",
"'convenience'",
"'fast_food'",
"'fast_food'",
"'restaurant'",
"'bicycle_shop'",
"'cafe'",
"'restaurant'",
"'hairdresser'",
"'convenience'",
"'bar'",
"'travel_agent'",
"'fast_food'",
"'hairdresser'",
"'convenience'",
"'community_centre'",
"'arts_centre'",
"'park'",
"'restaurant'",
"'convenience'",
"'restaurant'",
"'fast_food'",
"'restaurant'",
"'convenience'"],
["'kindergarten'",
"'pharmacy'",
"'kindergarten'",
"'playground'",
"'clinic'",
"'dentist'"],
["'restaurant'",
"'convenience'",
"'kindergarten'",
"'pub'",
"'community_centre'",
"'playground'",
"'pitch'",
"'school'",
"'pitch'",
"'park'",
"'pitch'",
"'pub'",
"'car_dealership'",
"'playground'",
"'pitch'"],
["'park'",
"'fast_food'",
"'fast_food'",
"'hairdresser'",
"'fast_food'",
"'supermarket'",
"'hairdresser'",
"'newsagent'",
"'supermarket'",
"'convenience'",
"'cafe'",
"'beverages'",
"'optician'",
"'hairdresser'",
"'supermarket'",
"'hairdresser'",
"'fast_food'",
"'bakery'",
"'fast_food'",
"'fast_food'",
"'pitch'",
"'beauty_shop'",
"'park'",
"'kindergarten'",
"'dentist'",
"'library'",
"'pub'",
"'community_centre'",
"'doctors'",
"'car_rental'",
"'car_dealership'"],
["'clinic'",
"'pub'",
"'convenience'",
"'hairdresser'",
"'veterinary'",
"'school'",
"'pitch'",
"'playground'"],
["'school'",
"'pitch'",
"'playground'",
"'convenience'",
"'school'",
"'school'",
"'playground'",
"'park'",
"'ruins'"],
["'playground'", "'park'", "'school'"],
["'golf_course'",
"'park'",
"'pitch'",
"'kindergarten'",
"'park'",
"'beauty_shop'",
"'hairdresser'",
"'pitch'",
"'pitch'",
"'stadium'",
"'park'",
"'stadium'",
"'school'",
"'pitch'",
"'pharmacy'",
"'doctors'",
"'swimming_pool'",
"'pitch'"],
["'library'",
"'bakery'",
"'fast_food'",
"'post_office'",
"'pharmacy'",
"'supermarket'",
"'supermarket'"],
["'park'"],
["'kindergarten'",
"'clinic'",
"'swimming_pool'",
"'school'",
"'pitch'",
"'pitch'",
"'clinic'",
"'school'",
"'pitch'",
"'pitch'",
"'convenience'",
"'dentist'",
"'clothes'",
"'pub'",
"'cafe'",
"'greengrocer'",
"'clothes'",
"'shoe_shop'",
"'hairdresser'",
"'greengrocer'",
"'cafe'",
"'bookshop'",
"'restaurant'",
"'cafe'",
"'gift_shop'",
"'cafe'",
"'furniture_shop'",
"'beverages'",
"'jeweller'",
"'jeweller'",
"'cafe'",
"'jeweller'",
"'clothes'",
"'mall'",
"'florist'",
"'bar'",
"'gift_shop'",
"'restaurant'",
"'hairdresser'",
"'beauty_shop'"],
["'community_centre'",
"'restaurant'",
"'beauty_shop'",
"'restaurant'",
"'pub'",
"'school'",
"'park'",
"'nursing_home'",
"'pitch'",
"'nursing_home'",
"'nursing_home'",
"'water_works'",
"'car_wash'",
"'supermarket'",
"'golf_course'",
"'pitch'"],
["'kindergarten'",
"'school'",
"'park'",
"'park'",
"'pitch'",
"'park'",
"'park'",
"'restaurant'",
"'hairdresser'",
"'fast_food'",
"'bakery'",
"'fast_food'",
"'optician'",
"'bank'",
"'travel_agent'",
"'convenience'",
"'pub'",
"'fast_food'",
"'beauty_shop'",
"'stationery'",
"'pharmacy'",
"'fast_food'",
"'community_centre'",
"'cafe'",
"'cafe'",
"'pub'",
"'chemist'",
"'bank'",
"'fast_food'",
"'convenience'",
"'hairdresser'",
"'pub'",
"'butcher'",
"'playground'",
"'hairdresser'",
"'clinic'",
"'park'",
"'restaurant'",
"'restaurant'",
"'bar'",
"'restaurant'",
"'optician'",
"'park'",
"'dentist'",
"'hairdresser'",
"'fast_food'",
"'newsagent'",
"'fast_food'",
"'fast_food'",
"'laundry'",
"'veterinary'",
"'hairdresser'",
"'doctors'",
"'convenience'",
"'car_wash'",
"'doctors'"],
["'school'",
"'school'",
"'school'",
"'pitch'",
"'clinic'",
"'school'",
"'veterinary'",
"'convenience'",
"'florist'",
"'park'",
"'fast_food'",
"'convenience'",
"'hairdresser'",
"'fast_food'",
"'pharmacy'",
"'hairdresser'"],
["'stationery'",
"'newsagent'",
"'school'",
"'pub'",
"'school'",
"'playground'",
"'kindergarten'",
"'pub'",
"'park'",
"'playground'",
"'community_centre'",
"'park'",
"'park'",
"'supermarket'",
"'furniture_shop'",
"'community_centre'",
"'convenience'",
"'sports_centre'",
"'sports_centre'",
"'recycling_metal'"],
["'hairdresser'",
"'fast_food'",
"'bar'",
"'beauty_shop'",
"'beauty_shop'",
"'restaurant'",
"'bookshop'",
"'pub'",
"'beverages'",
"'sports_centre'",
"'optician'",
"'hairdresser'",
"'cafe'",
"'restaurant'",
"'clinic'",
"'laundry'",
"'clothes'",
"'supermarket'",
"'hairdresser'",
"'cafe'",
"'restaurant'",
"'cafe'",
"'supermarket'",
"'gift_shop'",
"'fast_food'",
"'clothes'",
"'convenience'",
"'restaurant'",
"'restaurant'",
"'restaurant'",
"'fast_food'",
"'bar'",
"'pub'",
"'restaurant'",
"'restaurant'",
"'hairdresser'",
"'bar'",
"'beauty_shop'",
"'hairdresser'",
"'restaurant'",
"'doityourself'",
"'restaurant'",
"'hairdresser'",
"'bar'",
"'beauty_shop'",
"'cafe'",
"'hairdresser'",
"'pharmacy'",
"'greengrocer'",
"'pub'",
"'beverages'",
"'hairdresser'",
"'bank'",
"'fast_food'",
"'clinic'",
"'beverages'",
"'cafe'",
"'bakery'",
"'restaurant'",
"'fast_food'",
"'fast_food'",
"'beauty_shop'",
"'cafe'",
"'doityourself'",
"'cafe'",
"'pub'",
"'fast_food'",
"'cafe'",
"'doctors'",
"'nursing_home'",
"'pub'",
"'bar'",
"'restaurant'",
"'school'",
"'restaurant'",
"'restaurant'",
"'clothes'",
"'hairdresser'"],
["'playground'",
"'community_centre'",
"'playground'",
"'park'",
"'pitch'",
"'cafe'",
"'convenience'",
"'pharmacy'",
"'fast_food'",
"'community_centre'",
"'hairdresser'",
"'fast_food'",
"'convenience'",
"'convenience'",
"'ruins'",
"'ruins'",
"'ruins'",
"'ruins'",
"'archaeological'",
"'ruins'",
"'ruins'",
"'park'",
"'attraction'",
"'community_centre'",
"'playground'",
"'archaeological'",
"'pitch'",
"'playground'"],
["'park'", "'pub'", "'convenience'"],
["'fast_food'",
"'convenience'",
"'pitch'",
"'pitch'",
"'community_centre'",
"'pitch'",
"'kindergarten'"]]
With such a corpus
, we then can train the neural network to learn the embedding for words in the corpus based on their use in the document. All the detailed processes (e.g., initializing, sampling, optimization, etc.) are encapsulated in the function called Word2Vec
provided by gensim
’s models
module (it also includes other advanced NLP models such as ldamodel
). I recommend you read its documentation to learn more.
# train the model
model = gensim.models.Word2Vec(corpus, min_count=1, vector_size=100, workers=3, window =3, sg = 1)
For the full documentation of the function Word2Vec
, please check it official webpage. In this example, you will see we used a set of parameters: corpus
as the main input; min_count
to indicate that if the frequency of a word is less than it, it will not be considered in the model; vector_size
to represent the dimensionality of the word embedding vector; workers
as the number of worker threads to train the model (it depends on your systems capability); window
, maximum distance between the current and predicted word (define the length of the context window); and sg
, training algorithm: 1 for skip-gram; otherwise CBOW.
How to determine these parameters sometimes depends on modeler’s experience as well as the experiment results. In general Machine Learning (ML)/Deep Learning (DL) field, scientists usually conduct a lot of experienment in order to tune the model to its best performance.
Now, with the trained model
, we can see the embedding of any word (place type here) by using code below. Note that wv
is short for word vector.
model.wv["'swimming_pool'"]
array([-0.07868499, 0.15542263, -0.02243729, 0.0780982 , 0.06268492,
-0.17506836, 0.04474231, 0.20812719, -0.13518877, -0.16856225,
-0.00936876, -0.17530479, 0.01920592, 0.06494124, 0.07890018,
-0.09049972, 0.1396448 , -0.0625847 , 0.02220419, -0.24016841,
0.07719523, 0.04147688, 0.23926069, -0.08266466, -0.04260313,
0.02942185, -0.18089837, 0.06170765, -0.07240283, 0.01278588,
0.18554497, -0.03862393, 0.10925482, -0.22881277, -0.03959438,
0.0808387 , -0.04443993, -0.03407258, -0.05998663, -0.09209066,
0.08889414, -0.13964166, 0.02546455, 0.04552466, 0.09247473,
-0.07186268, -0.1320326 , -0.13340887, 0.07253008, 0.02472992,
0.1134716 , -0.05348552, -0.02400114, -0.06314866, -0.0511739 ,
-0.00187449, 0.06196023, -0.021495 , -0.1258097 , -0.01221897,
0.05093616, 0.030013 , 0.10772886, 0.12132879, -0.03753583,
0.19692194, -0.01060558, 0.1904243 , -0.21384415, 0.07034072,
0.01108875, 0.13314134, 0.1497802 , 0.05222565, 0.23323917,
-0.01140199, 0.02901526, 0.07102555, 0.04408478, -0.06825732,
-0.24026968, -0.04105622, -0.16340482, 0.1894222 , -0.04756841,
-0.14866339, 0.23206829, 0.05334442, 0.09805954, 0.03633046,
0.18999442, -0.01930966, 0.03973996, -0.06707993, 0.18876894,
0.03653833, 0.06362845, -0.01829909, -0.01852821, -0.05128703],
dtype=float32)
Same to what we have done in Lab 03, we next can compute the simiarlity between these vectors. Here are where the “magic” comes from. The semantics of these qualitative terms becomes computable, which empowers us, assisted by the machine, to conduct a lot of interesting analyses/processes.
For instance, we can compute the (semantic) simiarity between words (place types):
# compute similairity between the semantics of cafe and park
sim_restaurant_park = model.wv.similarity("'cafe'", "'park'")
sim_restaurant_park
0.9949972
# compute similairity between the semantics of cafe and fast food
sim_restaurant_fastFood = model.wv.similarity("'cafe'", "'fast_food'")
sim_restaurant_fastFood
0.9970947
First, you can see that the similarity between cafe
and park
is smaller than cafe
and fast food
, which aligns to our intuition. Next, we can also use the built-in function most_similar()
to get the most similar word to the target:
model.wv.most_similar("'cafe'")[:5]
[("'travel_agent'", 0.997725248336792),
("'bakery'", 0.9977042078971863),
("'outdoor_shop'", 0.9976359009742737),
("'arts_centre'", 0.9976054430007935),
("'mall'", 0.9975782036781311)]
Note that such a similarity is computed based on Euclidean distance. Here, we can also implement the version of computing cosine simiarity (you acatully have done it in previous labs). Before doing that, we need to build a function to get the vocabulary based on the corpus:
# get the vocabulary of the corpus
def voc_generator(corpus):
voc = []
for doc in corpus:
for word in doc:
voc.append(word)
voc = list(set(voc))
return voc
voc = voc_generator(corpus)
voc
["'archaeological'",
"'bank'",
"'theme_park'",
"'golf_course'",
"'car_rental'",
"'cinema'",
"'fire_station'",
"'toilet'",
"'mobile_phone_shop'",
"'bar'",
"'library'",
"'recycling'",
"'optician'",
"'tower'",
"'guesthouse'",
"'post_office'",
"'butcher'",
"'cafe'",
"'nightclub'",
"'dentist'",
"'chemist'",
"'bakery'",
"'convenience'",
"'stadium'",
"'food_court'",
"'stationery'",
"'recycling_metal'",
"'veterinary'",
"'tourist_info'",
"'shelter'",
"'monument'",
"'hospital'",
"'mall'",
"'toy_shop'",
"'travel_agent'",
"'caravan_site'",
"'fast_food'",
"'video_shop'",
"'nan'",
"'graveyard'",
"'water_tower'",
"'greengrocer'",
"'bookshop'",
"'artwork'",
"'police'",
"'sports_centre'",
"'water_works'",
"'wastewater_plant'",
"'hotel'",
"'kindergarten'",
"'track'",
"'hostel'",
"'furniture_shop'",
"'jeweller'",
"'shoe_shop'",
"'supermarket'",
"'car_dealership'",
"'sports_shop'",
"'computer_shop'",
"'attraction'",
"'clothes'",
"'swimming_pool'",
"'arts_centre'",
"'fountain'",
"'clinic'",
"'university'",
"'pub'",
"'doctors'",
"'museum'",
"'prison'",
"'department_store'",
"'observation_tower'",
"'memorial'",
"'market_place'",
"'recycling_glass'",
"'courthouse'",
"'park'",
"'community_centre'",
"'newsagent'",
"'college'",
"'ruins'",
"'restaurant'",
"'nursing_home'",
"'town_hall'",
"'garden_centre'",
"'pharmacy'",
"'doityourself'",
"'school'",
"'public_building'",
"'comms_tower'",
"'laundry'",
"'gift_shop'",
"'theatre'",
"'car_wash'",
"'camp_site'",
"'playground'",
"'outdoor_shop'",
"'pitch'",
"'picnic_site'",
"'florist'",
"'beauty_shop'",
"'beverages'",
"'hairdresser'",
"'bicycle_shop'",
"'castle'"]
The below function is to compute the cosine similarity, which is slightly different than what we have implemented before; but the core is the same! Try to read it and see if you can understand the code. It is to show you that in Python, you have the flexibilty to write any functions. Different people might write the function in different ways to achieve the same thing.
# compute Cosine similarity
import numpy as np
from numpy.linalg import norm
def cosine_distance (model, word,target_list , num) :
cosine_dict ={}
word_list = []
a = model.wv[word]
for item in target_list :
if item != word :
b = model.wv[item]
cos_sim = np.dot(a, b)/(norm(a)*norm(b))
cosine_dict[item] = cos_sim
dist_sort=sorted(cosine_dict.items(), key=lambda dist: dist[1],reverse = True) ## in Descedning order
for item in dist_sort:
word_list.append((item[0], item[1]))
return word_list[0:num]
Now, we can test the function by checking the top 5 similar words for ‘cafe’ based on its cosine similarity with other word vectors:
cosine_distance (model,"'cafe'",voc,5)
[("'travel_agent'", 0.99772507),
("'bakery'", 0.99770415),
("'outdoor_shop'", 0.9976358),
("'arts_centre'", 0.99760526),
("'mall'", 0.99757826)]
Okay! So far you have learnt and implemented the very fundemental technique in NLP/Geospatial Semantics: Word2Vec. Such a quantitative representation of semantics facilitates us to do many interesting things in addition to simply computing similarities between words. For instance, you can use the word vectors to cluster/group words, to execute calssification when seeing a new word, to predict the next words giving a set of seen words, etc. (ChaptGPT is indeed built on this idea!) Again, be creative in your future projects.
Part 2: Visualizing Word Embedding#
In Lab 3, we learned a technique called WordCloud to visulize natural language based on the frequency of terms. WordCloud can only tell us which word occurs the most in the document without having any implications on the meaning of these words. With word embedding, we can now visulize natural language based on their semantics. In the code below, we implememt a technique called TSNE (t-distributed stochastic neighbor embedding). It maps the high dimensional vector into 2-dimension (2D), so that words’ embeddings can now be mapped onto a plane. During such a mapping, TSNE attempts to keep the intrinsic relationships between the embeddings in the original high dimension. There are many mathematical formulas involved in this technique, which is beyond the scope of this unit. But if you are interested at the details (not required), check https://en.wikipedia.org/wiki/T-distributed_stochastic_neighbor_embedding.
Basically, in the code below, we use sklearn
library’s TSNE package, where the mapping will be produced. Then we extract the 2 dimensions as x-val
and y-val
. Finally, we use matplotlib
to visualize the 2 dimensions as a 2D plot. During the process, we also randomly selected 25 words (place types) and label them on the plot.
Note that we have used both sklearn
and matplotlib
already in previous labs. Indeed, these libraries introduced in this unit are the main ones spatial data scientists often use. You will get more and more familiar of using them in your career.
## tsn visualization
from sklearn.decomposition import IncrementalPCA
from sklearn.manifold import TSNE
import numpy as np
import matplotlib.pyplot as plt
import random
def reduce_dimensions(model):
num_dimensions = 2 # final num dimensions (2D, 3D, etc)
# extract the words & their vectors, as numpy arrays
vectors = np.asarray(model.wv.vectors)
labels = np.asarray(model.wv.index_to_key)
# reduce using t-SNE
tsne = TSNE(n_components=num_dimensions, random_state=0)
vectors = tsne.fit_transform(vectors)
x_vals = [v[0] for v in vectors]
y_vals = [v[1] for v in vectors]
return x_vals, y_vals, labels
def plot_with_matplotlib(x_vals, y_vals, labels):
random.seed(0)
plt.figure(figsize=(12, 12))
plt.scatter(x_vals, y_vals)
#
# Label randomly subsampled 25 data points
#
indices = list(range(len(labels)))
selected_indices = random.sample(indices, 25)
for i in selected_indices:
plt.annotate(labels[i], (x_vals[i], y_vals[i]))
x_vals, y_vals, labels = reduce_dimensions(model)
plot_with_matplotlib(x_vals, y_vals, labels)
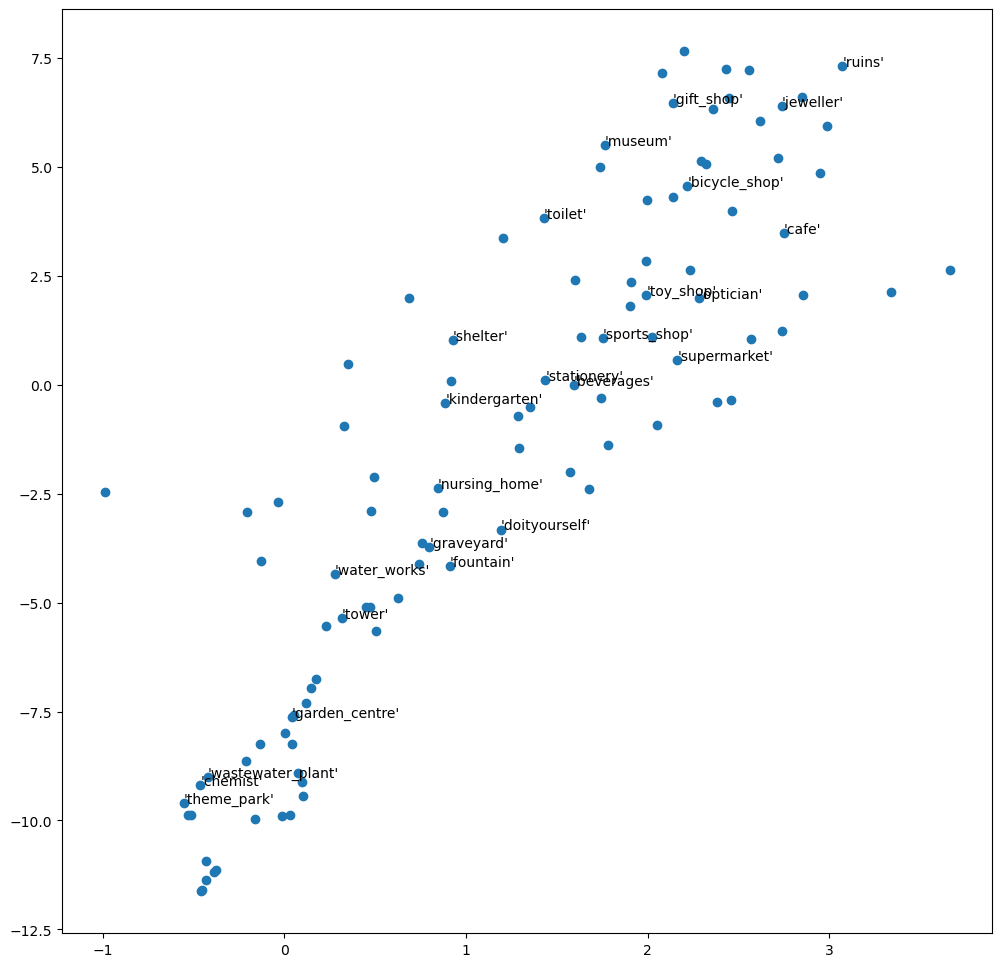
From the plot, you can see that, for example, theme park
is close to wastewater_plant
, fountain
is close to graveyard
, and so on. It shows the semantic similarities between words. Again, note that since our data is relatively small, these similarities might not always align to human’s judgement.
Part 3: Computing Document Embedding#
With the word embedding, you may then wonder how we can compute the document embedding? There are many existing methods. For instance, the most popular approach is called Doc2Vec
(such a name is not suprising, right?). gensim
also provides a module for it, check here: https://radimrehurek.com/gensim/models/doc2vec.html if you are interested (it should be straightforward after you have implemented Word2Vec
). In addition to Doc2Vec
, a simpler approach is to use the embedding from words, and compute the average of word embeddings contained in the document as the document embedding. The function can be implemented as below:
def compute_docEmbedding(docList, model, dim):
doc_embed_list = []
for doc in docList:
doc_embed = np.zeros(dim)
doc_n = 0
for word in doc:
word_embed = model.wv[word]
doc_embed = doc_embed+ word_embed
doc_n +=1
doc_embed_list.append(doc_embed/doc_n)
return doc_embed_list
We can then use this function to get the document embeddings:
doc_embedding = compute_docEmbedding(corpus, model, 100)
doc_embedding[0] # an example
array([-0.08329689, 0.15261881, -0.0233225 , 0.07659075, 0.06435439,
-0.17650226, 0.03916298, 0.20592766, -0.13470526, -0.17028349,
-0.00497893, -0.1680778 , 0.01734873, 0.06769949, 0.08084807,
-0.0821867 , 0.14041259, -0.06189171, 0.01712094, -0.23346457,
0.07382787, 0.04751505, 0.23992209, -0.08007029, -0.0396951 ,
0.02947526, -0.17599729, 0.06590427, -0.06975804, 0.01705565,
0.18358559, -0.03782592, 0.10420692, -0.21963379, -0.04270642,
0.08902049, -0.0432584 , -0.03484922, -0.05787984, -0.09186516,
0.09282112, -0.12858916, 0.02434939, 0.0415485 , 0.0961752 ,
-0.06557396, -0.13535978, -0.13008115, 0.0721503 , 0.02454712,
0.11480464, -0.05069575, -0.02788911, -0.06926043, -0.04776864,
-0.00395558, 0.05532643, -0.01488321, -0.11633152, -0.01831812,
0.04589558, 0.03747189, 0.10735734, 0.11727599, -0.03319626,
0.19077188, -0.00932337, 0.18625706, -0.20991499, 0.06863999,
0.0126708 , 0.13343945, 0.14200287, 0.05179945, 0.22576441,
-0.0154038 , 0.02880589, 0.06625615, 0.04314442, -0.06523215,
-0.23392626, -0.03967105, -0.1568101 , 0.18817133, -0.04292334,
-0.14623692, 0.22846387, 0.05329637, 0.09399697, 0.03575206,
0.18140565, -0.01724123, 0.03809967, -0.06591525, 0.18705835,
0.03376547, 0.06510156, -0.01710109, -0.01255403, -0.04729071])
With such an embedding, you can then do all the similar things we have done for words (e.g., compare different documents (regions), visualize the similarity between regions, etc.) Try it yourself. We can also impelement a simple IR system like what we did in Lab 03 using document emebedding now. Can you do it yourself?
After computing document embedding by averaging vectors of word, do you see any disadvantage of such an approach?
Part 4: Topic Modeling on Geo-text (optional)#
Beyond Word2Vec
, where word embedding is learnt first to represent the semantic similarity between words, another more advanced technique is the family of Topic Modeling. It extracts the hidden topics from large volumes of text. So, it means to capture more complex semantics hidden in texts, rather than simply relying on the interaction between words. It heavily involves probablistic models. Topic models are useful for purpose of document clustering, organizing large blocks of textual data, information retrieval from unstructured text, feature selection, etc.
There are many such topic models, including Latent Semantic Analysis or Indexing(LSA/LSI), Hierarchical Dirichlet process (HDP), and Latent Dirichlet Allocation(LDA). We will cover LDA in this lab due to its recent popularity.
In LDA, it considers each document as a collection of topics and each topic as collection of keywords (words/terms). You can see there is a hidden/middle layer - called topic - here between document and words. Once you set up the parameters (e.g., number of topics), the model will then optimize the topic distribution of the document, as well as the word distribution of each topic.
We will use the same dataset bristol_lsoal_poi_full
for this experiment for the sake of simplicity. But again, it might not be a good dataset as it is relatively small and many documents only contain couple of words, so it might be difficult to learn the topic distribution for these document.
When you have a larger dataset, like newspapers, you might also want to use the library Spacy
to preprocess the data, such as removing stopwords, applying lemmatization, removing punctuation, etc before train the topic model LDA.
To implement LDA, we can still use the gensim
library as before. To visualize its results, we need to install a new package:
After all libraries are installed, now let’s import all of them:
import gensim.corpora as corpora
import pyLDAvis
import pyLDAvis.gensim_models as gensimvis
from gensim.models import CoherenceModel
First, we need to have a Document Term matrix (DTM). We have implemented it in Lab 3 as well. Luckily, in gensim
, we do not need to do it manually. But we need to pass the vocabulary (tokenized words) to gensim
:
dict_ = corpora.Dictionary(corpus)
print(dict_)
Dictionary<105 unique tokens: ["'park'", "'playground'", "'swimming_pool'", "'butcher'", "'cafe'"]...>
We can see the words from the vocabulary using:
for word in dict_.values():
print(word)
'park'
'playground'
'swimming_pool'
'butcher'
'cafe'
'car_dealership'
'car_wash'
'clinic'
'college'
'community_centre'
'computer_shop'
'convenience'
'dentist'
'doityourself'
'fast_food'
'hairdresser'
'kindergarten'
'nursing_home'
'restaurant'
'sports_centre'
'theatre'
'beauty_shop'
'pitch'
'pub'
'sports_shop'
'toy_shop'
'furniture_shop'
'hotel'
'school'
'pharmacy'
'bakery'
'gift_shop'
'greengrocer'
'arts_centre'
'attraction'
'bar'
'beverages'
'cinema'
'clothes'
'florist'
'fountain'
'library'
'museum'
'nightclub'
'supermarket'
'toilet'
'tourist_info'
'town_hall'
'track'
'outdoor_shop'
'veterinary'
'mobile_phone_shop'
'travel_agent'
'bank'
'car_rental'
'chemist'
'doctors'
'jeweller'
'optician'
'post_office'
'shoe_shop'
'tower'
'bookshop'
'laundry'
'university'
'graveyard'
'mall'
'newsagent'
'shelter'
'bicycle_shop'
'hospital'
'guesthouse'
'police'
'golf_course'
'fire_station'
'hostel'
'archaeological'
'recycling_glass'
'wastewater_plant'
'nan'
'water_tower'
'camp_site'
'water_works'
'department_store'
'market_place'
'ruins'
'video_shop'
'garden_centre'
'recycling'
'castle'
'courthouse'
'memorial'
'stadium'
'stationery'
'prison'
'public_building'
'artwork'
'comms_tower'
'food_court'
'monument'
'observation_tower'
'caravan_site'
'picnic_site'
'theme_park'
'recycling_metal'
Now, we are ready to generate the DTM using doc2bow()
function. bow
means “bag of words”.
doc_term_matrix = [dict_.doc2bow(i) for i in corpus]
doc_term_matrix
[[(0, 1), (1, 1), (2, 2)],
[(3, 1),
(4, 1),
(5, 1),
(6, 1),
(7, 1),
(8, 1),
(9, 1),
(10, 1),
(11, 3),
(12, 1),
(13, 3),
(14, 1),
(15, 2),
(16, 1),
(17, 1),
(18, 3),
(19, 3),
(20, 1)],
[(0, 2), (1, 1), (4, 1), (9, 1), (11, 2), (14, 3), (15, 2), (16, 2), (21, 1)],
[(1, 2),
(5, 1),
(11, 1),
(14, 1),
(21, 1),
(22, 2),
(23, 1),
(24, 1),
(25, 1)],
[(0, 1),
(1, 1),
(5, 1),
(8, 1),
(11, 1),
(14, 2),
(16, 1),
(18, 1),
(22, 3),
(23, 2),
(26, 1),
(27, 2),
(28, 1)],
[(0, 1),
(5, 1),
(6, 1),
(11, 1),
(13, 3),
(14, 2),
(15, 1),
(21, 1),
(23, 1),
(29, 1)],
[(11, 1), (18, 1), (19, 1), (23, 4), (30, 1), (31, 1), (32, 1)],
[(0, 5),
(4, 14),
(7, 1),
(9, 1),
(11, 2),
(14, 13),
(15, 9),
(18, 23),
(19, 1),
(20, 2),
(21, 2),
(23, 9),
(26, 1),
(27, 1),
(28, 1),
(29, 1),
(31, 1),
(33, 2),
(34, 1),
(35, 7),
(36, 3),
(37, 1),
(38, 2),
(39, 1),
(40, 5),
(41, 1),
(42, 2),
(43, 4),
(44, 2),
(45, 1),
(46, 1),
(47, 1)],
[(0, 1),
(1, 1),
(5, 2),
(11, 3),
(13, 2),
(15, 2),
(17, 1),
(28, 2),
(30, 1)],
[(0, 3), (8, 1), (14, 1), (19, 1), (22, 1), (28, 1), (48, 1)],
[(1, 1),
(4, 1),
(7, 2),
(11, 2),
(12, 1),
(13, 1),
(14, 3),
(18, 2),
(19, 1),
(23, 5),
(24, 1),
(49, 1),
(50, 1)],
[(0, 2),
(1, 2),
(3, 3),
(4, 3),
(6, 1),
(11, 5),
(14, 7),
(15, 5),
(18, 9),
(21, 2),
(23, 4),
(25, 1),
(29, 2),
(30, 2),
(31, 1),
(38, 3),
(44, 4),
(51, 1),
(52, 2)],
[(0, 1),
(1, 1),
(3, 1),
(4, 8),
(5, 1),
(6, 1),
(7, 1),
(11, 2),
(14, 9),
(15, 7),
(18, 1),
(19, 1),
(21, 3),
(22, 1),
(23, 6),
(26, 2),
(29, 1),
(31, 1),
(32, 1),
(33, 1),
(36, 1),
(38, 2),
(41, 1),
(43, 1),
(44, 1),
(45, 1),
(49, 1),
(51, 7),
(52, 1),
(53, 2),
(54, 1),
(55, 1),
(56, 1),
(57, 1),
(58, 2),
(59, 1),
(60, 1)],
[(1, 1), (22, 1), (61, 1)],
[(0, 1),
(2, 1),
(4, 1),
(7, 1),
(11, 2),
(12, 1),
(14, 2),
(15, 3),
(16, 1),
(18, 3),
(23, 3),
(27, 2),
(28, 1),
(35, 1),
(62, 1),
(63, 1),
(64, 2)],
[(0, 2), (9, 1), (11, 1), (22, 2), (28, 1)],
[(28, 1), (59, 1)],
[(0, 2),
(1, 1),
(4, 1),
(6, 1),
(7, 1),
(9, 1),
(12, 1),
(14, 3),
(15, 2),
(16, 2),
(22, 4),
(23, 1),
(28, 1),
(30, 1),
(31, 1),
(39, 1),
(45, 1),
(50, 1),
(53, 2),
(65, 1),
(66, 1),
(67, 1),
(68, 1)],
[(5, 1),
(11, 3),
(12, 1),
(13, 1),
(14, 5),
(15, 2),
(18, 2),
(21, 2),
(22, 3),
(23, 3),
(26, 1),
(28, 1),
(29, 1),
(30, 2),
(36, 4),
(58, 1),
(59, 1),
(67, 1)],
[(0, 4),
(1, 2),
(3, 4),
(4, 7),
(5, 1),
(7, 1),
(9, 1),
(10, 1),
(11, 6),
(14, 7),
(15, 3),
(18, 3),
(19, 2),
(22, 3),
(26, 2),
(28, 3),
(29, 1),
(32, 1),
(35, 2),
(38, 2),
(41, 1),
(51, 2),
(52, 1),
(58, 1),
(69, 1)],
[(9, 1), (14, 1), (15, 2), (18, 2), (23, 1), (50, 1), (56, 1), (65, 1)],
[(1, 1), (4, 1), (11, 1), (14, 1), (22, 1), (23, 1), (28, 1)],
[(0, 1),
(4, 1),
(5, 4),
(9, 1),
(11, 1),
(15, 1),
(22, 1),
(23, 1),
(29, 1),
(32, 1),
(36, 1)],
[(15, 2), (67, 1)],
[(0, 1),
(2, 1),
(7, 2),
(11, 1),
(12, 1),
(14, 2),
(15, 3),
(18, 2),
(21, 1),
(30, 1),
(53, 1),
(56, 1)],
[(0, 3),
(1, 1),
(4, 1),
(11, 2),
(14, 1),
(15, 2),
(17, 1),
(18, 1),
(22, 3),
(28, 1),
(29, 1),
(64, 4),
(70, 1)],
[(0, 4),
(4, 1),
(7, 1),
(9, 1),
(14, 2),
(15, 1),
(16, 1),
(21, 1),
(23, 1),
(44, 1),
(71, 1),
(72, 1)],
[(4, 1),
(11, 3),
(14, 5),
(15, 2),
(16, 1),
(28, 2),
(29, 2),
(41, 1),
(50, 1),
(58, 1)],
[(0, 2), (11, 2), (14, 2), (15, 2), (28, 1), (39, 1)],
[(0, 3), (1, 2), (4, 1), (9, 1), (11, 1), (22, 1), (41, 1)],
[(4, 9),
(7, 1),
(9, 1),
(11, 2),
(13, 1),
(14, 2),
(15, 2),
(16, 2),
(18, 7),
(19, 1),
(21, 3),
(22, 1),
(23, 3),
(24, 2),
(26, 9),
(28, 1),
(29, 1),
(31, 1),
(32, 1),
(35, 3),
(38, 1),
(41, 1),
(44, 2),
(45, 1),
(56, 1),
(58, 2),
(62, 1),
(63, 1),
(66, 1),
(67, 1),
(68, 2),
(69, 1),
(72, 1)],
[(1, 1),
(9, 1),
(11, 1),
(12, 1),
(13, 1),
(14, 2),
(15, 3),
(19, 1),
(22, 4),
(28, 1),
(29, 1),
(44, 1),
(56, 1),
(63, 1),
(70, 1)],
[(0, 6),
(1, 1),
(2, 1),
(4, 1),
(9, 1),
(11, 2),
(12, 1),
(14, 1),
(15, 1),
(21, 2),
(22, 2),
(28, 1),
(41, 1),
(73, 1)],
[(0, 2), (1, 1), (11, 1), (13, 1), (14, 1), (23, 2)],
[(0, 3),
(1, 1),
(5, 1),
(14, 4),
(15, 2),
(18, 1),
(21, 2),
(22, 1),
(23, 2),
(28, 2),
(38, 1),
(41, 1),
(42, 1),
(44, 4),
(51, 1),
(52, 1),
(53, 1),
(57, 1),
(58, 1),
(64, 1),
(70, 1)],
[(0, 2),
(1, 1),
(4, 1),
(7, 1),
(14, 1),
(15, 1),
(16, 1),
(18, 1),
(22, 1),
(24, 1),
(26, 1),
(28, 1),
(31, 1),
(32, 1),
(38, 1),
(51, 1),
(68, 1)],
[(1, 1),
(5, 1),
(11, 1),
(21, 1),
(22, 1),
(23, 1),
(28, 1),
(54, 1),
(70, 1),
(74, 1)],
[(0, 1),
(4, 3),
(5, 2),
(7, 1),
(11, 1),
(13, 3),
(14, 5),
(15, 4),
(18, 2),
(21, 2),
(23, 3),
(26, 2),
(35, 1),
(36, 1),
(38, 1),
(39, 1),
(44, 1),
(57, 1),
(62, 1),
(67, 1),
(69, 1)],
[(0, 1)],
[(5, 1), (11, 1), (14, 1), (22, 2), (28, 1), (44, 1), (54, 1), (56, 1)],
[(0, 4),
(1, 1),
(2, 2),
(4, 1),
(11, 2),
(15, 1),
(17, 1),
(22, 2),
(23, 1),
(28, 2)],
[(0, 1), (23, 1)],
[(0, 1),
(1, 1),
(11, 1),
(15, 1),
(16, 1),
(22, 7),
(23, 1),
(28, 1),
(36, 1),
(61, 1),
(65, 1)],
[(0, 1),
(1, 1),
(6, 1),
(11, 1),
(13, 1),
(14, 2),
(15, 2),
(21, 1),
(22, 3),
(28, 1),
(36, 1)],
[(0, 3),
(1, 2),
(4, 2),
(8, 1),
(11, 2),
(15, 2),
(18, 2),
(20, 1),
(23, 1),
(27, 3),
(28, 2),
(35, 3),
(43, 1),
(65, 1),
(75, 1)],
[(0, 1),
(7, 1),
(9, 2),
(10, 1),
(14, 2),
(15, 4),
(17, 2),
(22, 3),
(36, 1),
(67, 1)],
[(0, 3),
(1, 1),
(5, 3),
(11, 1),
(14, 2),
(22, 6),
(28, 1),
(30, 1),
(42, 1),
(48, 1),
(54, 2),
(68, 1),
(72, 1),
(76, 2),
(77, 1),
(78, 1)],
[(2, 1), (5, 2), (7, 2), (13, 1), (15, 1), (22, 3)],
[(0, 1),
(1, 1),
(4, 1),
(11, 2),
(12, 1),
(14, 2),
(18, 1),
(19, 1),
(23, 4),
(30, 1),
(44, 1)],
[(11, 1), (12, 1), (14, 1), (15, 1), (18, 1), (21, 2), (50, 1)],
[(1, 1), (9, 2), (16, 1), (19, 1), (22, 5), (28, 2)],
[(5, 1), (14, 1), (15, 1), (23, 1)],
[(0, 4),
(1, 3),
(3, 2),
(4, 2),
(9, 1),
(11, 6),
(14, 2),
(18, 2),
(21, 1),
(22, 2),
(23, 2),
(26, 1),
(28, 2),
(44, 1),
(51, 1),
(52, 1),
(57, 1),
(59, 1),
(63, 1)],
[(79, 1)],
[(0, 4), (9, 1), (28, 1), (56, 1), (70, 1)],
[(0, 1), (2, 1), (9, 1), (16, 1), (23, 1), (56, 1), (80, 1)],
[(11, 1), (28, 1), (41, 1)],
[(0, 1), (22, 4), (28, 2), (41, 1)],
[(0, 5), (22, 2), (28, 1), (34, 1), (44, 2), (54, 1), (70, 1), (81, 1)],
[(0, 2),
(1, 2),
(3, 1),
(4, 6),
(7, 1),
(9, 1),
(11, 6),
(14, 5),
(15, 2),
(18, 4),
(22, 3),
(23, 5),
(28, 2),
(30, 1),
(35, 1),
(44, 1),
(53, 1),
(56, 1)],
[(0, 3),
(1, 1),
(3, 3),
(4, 1),
(6, 1),
(7, 2),
(9, 1),
(11, 10),
(12, 1),
(14, 9),
(15, 8),
(18, 3),
(21, 1),
(22, 1),
(23, 2),
(29, 1),
(32, 1),
(38, 1),
(39, 1),
(51, 1),
(52, 2),
(63, 1),
(69, 1)],
[(0, 2), (9, 1), (11, 1), (14, 2), (22, 1), (23, 1)],
[(0, 1), (4, 1), (15, 1), (23, 1), (25, 1), (28, 2), (30, 1), (67, 1)],
[(0, 1),
(4, 1),
(9, 1),
(11, 2),
(14, 2),
(15, 2),
(21, 3),
(28, 1),
(30, 1),
(35, 1),
(36, 1),
(39, 1),
(52, 1),
(56, 2),
(58, 1),
(66, 1),
(67, 1),
(69, 1)],
[(0, 5),
(1, 1),
(5, 2),
(9, 1),
(11, 2),
(14, 4),
(15, 1),
(18, 2),
(23, 1),
(24, 1),
(28, 1),
(30, 1),
(35, 2),
(36, 1),
(56, 1)],
[(0, 3),
(1, 1),
(4, 2),
(5, 1),
(6, 2),
(14, 2),
(22, 4),
(23, 1),
(29, 1),
(44, 1),
(56, 1),
(82, 1)],
[(0, 1), (1, 2), (4, 1), (9, 1), (22, 4), (23, 1), (28, 1), (45, 1), (68, 1)],
[(0, 2),
(1, 1),
(4, 1),
(6, 1),
(9, 2),
(11, 1),
(14, 2),
(15, 2),
(26, 2),
(28, 3),
(29, 1),
(34, 1),
(44, 3),
(50, 1),
(56, 1),
(63, 1),
(69, 1),
(83, 1),
(84, 1)],
[(0, 1),
(1, 1),
(2, 1),
(4, 3),
(5, 2),
(13, 2),
(14, 1),
(16, 1),
(19, 2),
(22, 21),
(27, 1),
(28, 2),
(82, 1),
(85, 1)],
[(0, 3),
(5, 1),
(9, 1),
(11, 2),
(12, 1),
(13, 3),
(14, 2),
(15, 1),
(17, 1),
(22, 1),
(23, 1),
(28, 1)],
[(1, 1),
(2, 1),
(8, 1),
(12, 2),
(14, 1),
(15, 2),
(18, 2),
(21, 2),
(22, 1),
(28, 1),
(36, 1),
(44, 1),
(69, 1)],
[(11, 1),
(14, 2),
(15, 1),
(18, 7),
(21, 1),
(23, 2),
(26, 2),
(27, 1),
(35, 1),
(38, 1),
(60, 1),
(63, 1)],
[(5, 3), (10, 1), (11, 2), (14, 1), (15, 3), (21, 1), (23, 3), (28, 1)],
[(26, 1), (28, 1), (44, 1)],
[(0, 6), (1, 1), (13, 2), (22, 3), (23, 1), (28, 1), (68, 1)],
[(0, 2), (2, 2), (16, 1), (22, 1), (28, 1)],
[(0, 1), (6, 1), (9, 1), (11, 1), (12, 1), (29, 1), (44, 1), (56, 1)],
[(4, 1), (14, 1), (23, 1), (28, 1), (41, 1), (50, 1)],
[(0, 1), (1, 1), (19, 1), (28, 2), (34, 1)],
[(0, 1),
(1, 1),
(3, 1),
(7, 1),
(11, 2),
(14, 4),
(15, 1),
(21, 1),
(22, 3),
(23, 2),
(28, 1),
(65, 1)],
[(0, 1), (11, 1), (14, 1), (15, 1), (23, 1), (28, 1), (65, 1)],
[(0, 1), (5, 1), (9, 1), (16, 1), (23, 1), (65, 1)],
[(0, 2),
(1, 1),
(9, 2),
(11, 1),
(14, 3),
(15, 2),
(21, 1),
(22, 2),
(28, 3),
(32, 1),
(36, 1),
(44, 2)],
[(2, 1), (15, 1), (22, 5), (28, 3), (44, 1)],
[(0, 2),
(1, 2),
(5, 1),
(9, 1),
(14, 1),
(22, 1),
(24, 1),
(26, 2),
(28, 1),
(38, 2),
(48, 4),
(73, 1)],
[(1, 1),
(5, 1),
(6, 1),
(11, 2),
(13, 1),
(15, 1),
(17, 1),
(22, 1),
(28, 1),
(56, 1)],
[(9, 1), (13, 1), (22, 1), (28, 2), (29, 1), (44, 1), (56, 1)],
[(0, 2), (1, 3), (22, 1), (28, 1)],
[(0, 1),
(1, 1),
(2, 1),
(4, 3),
(7, 1),
(10, 1),
(11, 5),
(12, 1),
(14, 6),
(15, 6),
(18, 7),
(22, 1),
(23, 4),
(26, 1),
(29, 2),
(30, 1),
(32, 1),
(35, 3),
(36, 1),
(38, 4),
(39, 1),
(44, 2),
(51, 1),
(58, 1),
(63, 1)],
[(9, 1), (11, 1), (14, 2), (15, 1), (18, 2), (23, 3), (30, 1)],
[(0, 1), (22, 3), (23, 1), (28, 1)],
[(14, 1),
(15, 3),
(21, 3),
(23, 1),
(26, 1),
(38, 1),
(39, 1),
(41, 1),
(44, 1),
(57, 1)],
[(1, 1), (22, 1), (28, 1)],
[(3, 1),
(4, 2),
(5, 1),
(11, 3),
(14, 7),
(15, 6),
(21, 2),
(28, 1),
(29, 1),
(30, 2),
(36, 1),
(38, 1),
(39, 1),
(44, 1),
(58, 1),
(59, 1),
(67, 1),
(86, 1)],
[(0, 2), (2, 2), (16, 1), (19, 1), (22, 2), (28, 5), (50, 1)],
[(0, 1), (28, 1), (73, 1)],
[(0, 1),
(1, 1),
(11, 1),
(15, 1),
(16, 1),
(22, 1),
(23, 1),
(28, 1),
(35, 1),
(73, 1),
(82, 1)],
[(5, 1), (9, 1), (11, 1), (13, 1), (19, 1), (22, 3), (28, 1), (87, 1)],
[(4, 2),
(7, 1),
(11, 3),
(12, 1),
(14, 1),
(15, 3),
(16, 2),
(18, 3),
(19, 1),
(21, 3),
(23, 3),
(28, 2),
(30, 1),
(43, 1),
(44, 1),
(69, 2)],
[(0, 2), (1, 1), (6, 1), (11, 1), (22, 6), (26, 1), (28, 1)],
[(0, 3),
(1, 1),
(22, 2),
(26, 1),
(27, 1),
(44, 1),
(48, 1),
(65, 2),
(70, 1),
(71, 1)],
[(0, 1),
(1, 1),
(4, 2),
(5, 2),
(9, 1),
(11, 1),
(14, 4),
(15, 4),
(18, 2),
(21, 2),
(22, 3),
(25, 2),
(35, 1),
(41, 1),
(44, 1),
(45, 1),
(65, 1),
(88, 1)],
[(6, 1), (15, 1), (16, 1), (23, 1), (28, 1), (53, 1)],
[(0, 1),
(4, 1),
(5, 1),
(6, 1),
(11, 1),
(12, 2),
(15, 2),
(18, 1),
(23, 1),
(44, 1),
(50, 1),
(65, 1),
(67, 1)],
[(3, 1),
(11, 2),
(12, 1),
(14, 1),
(15, 2),
(16, 1),
(23, 1),
(28, 1),
(29, 1),
(44, 1),
(71, 1)],
[(0, 1), (1, 1), (22, 1), (23, 2)],
[(3, 1),
(4, 1),
(5, 5),
(11, 4),
(14, 6),
(15, 7),
(18, 3),
(21, 6),
(23, 3),
(28, 1),
(30, 1),
(32, 1),
(36, 1),
(44, 1),
(49, 1),
(59, 1),
(63, 2),
(65, 1),
(67, 1),
(68, 1),
(69, 1),
(89, 1)],
[(0, 1), (11, 1), (14, 1), (23, 1), (28, 4), (41, 1), (44, 1)],
[(0, 3),
(4, 5),
(7, 1),
(8, 1),
(11, 1),
(14, 7),
(15, 2),
(16, 1),
(18, 4),
(23, 7),
(27, 5),
(28, 1),
(30, 1),
(35, 1),
(44, 2),
(53, 1),
(61, 1),
(63, 1),
(67, 1),
(74, 1),
(85, 1),
(90, 1),
(91, 1)],
[(0, 4),
(1, 2),
(2, 2),
(9, 1),
(11, 1),
(18, 1),
(22, 5),
(28, 2),
(42, 1),
(45, 1),
(54, 1)],
[(22, 1), (28, 1)],
[(0, 1), (11, 1), (14, 2), (28, 1), (72, 1)],
[(0, 1),
(1, 2),
(4, 1),
(5, 2),
(11, 1),
(13, 4),
(14, 6),
(15, 6),
(21, 1),
(22, 6),
(23, 1),
(28, 2),
(44, 1),
(51, 1)],
[(22, 6), (28, 2), (68, 1)],
[(11, 1), (14, 1), (15, 1), (22, 1), (92, 1)],
[(14, 1), (22, 6), (28, 1), (36, 1), (48, 1)],
[(0, 2),
(1, 2),
(4, 4),
(5, 5),
(13, 3),
(14, 2),
(15, 2),
(18, 1),
(22, 2),
(23, 1),
(27, 1),
(28, 1),
(35, 1),
(54, 2),
(65, 1),
(67, 1),
(84, 1),
(85, 1),
(93, 1)],
[(10, 1), (11, 1), (15, 1), (21, 1), (24, 1)],
[(13, 1), (15, 2), (71, 3)],
[(0, 5),
(1, 4),
(3, 2),
(4, 2),
(6, 1),
(7, 1),
(9, 2),
(10, 1),
(11, 9),
(12, 1),
(14, 6),
(15, 6),
(18, 8),
(19, 1),
(22, 7),
(23, 3),
(26, 2),
(28, 3),
(38, 1),
(44, 1),
(52, 2),
(59, 1),
(63, 2),
(67, 1),
(69, 1),
(93, 1)],
[(1, 3), (7, 2), (12, 1), (14, 1), (18, 1), (23, 3), (26, 1), (28, 1)],
[(0, 1),
(5, 6),
(6, 1),
(13, 3),
(14, 2),
(15, 1),
(23, 1),
(24, 1),
(30, 1),
(36, 1),
(44, 1),
(51, 1),
(67, 1)],
[(3, 1),
(4, 7),
(5, 1),
(6, 1),
(8, 1),
(11, 1),
(13, 1),
(14, 4),
(15, 4),
(16, 2),
(18, 5),
(19, 1),
(20, 1),
(21, 2),
(22, 1),
(23, 5),
(25, 1),
(26, 2),
(28, 1),
(30, 1),
(31, 3),
(32, 1),
(35, 1),
(39, 2),
(50, 1),
(53, 1),
(58, 1),
(60, 1),
(63, 2),
(67, 2),
(69, 2),
(92, 1),
(94, 1)],
[(0, 4),
(1, 1),
(5, 1),
(7, 2),
(11, 3),
(14, 3),
(15, 1),
(16, 2),
(21, 1),
(22, 2),
(28, 1),
(39, 1),
(44, 1),
(54, 1),
(74, 1)],
[(3, 1),
(4, 1),
(5, 1),
(11, 2),
(14, 4),
(15, 2),
(23, 2),
(36, 1),
(39, 1),
(63, 1)],
[(0, 2),
(4, 6),
(5, 1),
(6, 1),
(7, 1),
(10, 1),
(11, 1),
(12, 2),
(13, 2),
(14, 3),
(15, 2),
(18, 3),
(19, 1),
(20, 1),
(22, 1),
(23, 10),
(27, 1),
(28, 1),
(30, 3),
(35, 1),
(38, 3),
(39, 1),
(43, 4),
(50, 1),
(52, 1),
(54, 1),
(68, 2),
(69, 1),
(84, 1),
(93, 1)],
[(0, 1),
(1, 1),
(2, 1),
(14, 1),
(15, 1),
(22, 2),
(28, 1),
(38, 1),
(48, 1),
(56, 1),
(70, 1),
(82, 1)],
[(0, 3), (22, 1)],
[(0, 1),
(1, 1),
(8, 1),
(11, 2),
(14, 3),
(15, 1),
(22, 1),
(23, 2),
(28, 1),
(29, 1),
(92, 1)],
[(0, 1), (1, 1), (16, 1), (22, 3), (23, 1), (28, 1), (44, 1), (56, 1)],
[(0, 3), (12, 1), (22, 2), (95, 1)],
[(0, 1)],
[(1, 1), (7, 1), (11, 2), (14, 1), (22, 11), (28, 2), (43, 1), (44, 1)],
[(4, 1),
(5, 2),
(11, 3),
(14, 7),
(15, 11),
(18, 4),
(21, 4),
(22, 3),
(23, 2),
(24, 2),
(28, 1),
(44, 1),
(63, 1),
(71, 2)],
[(0, 3),
(1, 4),
(11, 2),
(14, 1),
(22, 3),
(30, 1),
(32, 1),
(67, 1),
(68, 1),
(96, 1)],
[(0, 1),
(17, 2),
(21, 1),
(22, 6),
(36, 1),
(42, 1),
(45, 1),
(47, 1),
(64, 1),
(69, 1),
(77, 1)],
[(0, 1),
(1, 1),
(11, 1),
(12, 1),
(14, 1),
(15, 4),
(16, 3),
(18, 1),
(21, 1),
(22, 3),
(23, 2),
(29, 1),
(30, 1),
(35, 1),
(41, 1),
(44, 1),
(63, 2),
(65, 1),
(93, 1)],
[(1, 1), (4, 1), (16, 1), (22, 2), (23, 1), (28, 2)],
[(4, 1), (11, 1), (16, 1), (21, 1), (22, 1), (23, 2), (65, 1)],
[(9, 1), (11, 1), (15, 2), (28, 1)],
[(0, 6),
(4, 3),
(7, 1),
(9, 3),
(11, 3),
(14, 5),
(15, 4),
(18, 1),
(23, 1),
(29, 2),
(30, 1),
(36, 1),
(41, 1),
(44, 2),
(95, 2)],
[(0, 1), (1, 1), (22, 4), (28, 2), (44, 1), (70, 1)],
[(9, 1), (22, 6), (28, 1)],
[(0, 2), (4, 1), (5, 2), (11, 4), (14, 1), (15, 2), (41, 1), (50, 2)],
[(0, 1), (15, 1), (16, 1), (22, 1), (28, 2)],
[(0, 1), (1, 2), (22, 2), (28, 2)],
[(0, 1),
(1, 1),
(4, 2),
(7, 1),
(9, 1),
(11, 6),
(12, 1),
(14, 4),
(15, 2),
(22, 5),
(28, 2),
(29, 1),
(30, 1),
(44, 1),
(56, 1),
(65, 1)],
[(0, 1), (1, 1), (5, 1), (22, 1), (23, 2), (28, 1), (30, 1)],
[(1, 1),
(4, 1),
(12, 1),
(14, 1),
(15, 1),
(18, 1),
(21, 1),
(22, 2),
(41, 1)],
[(9, 1), (23, 1), (28, 1), (48, 1)],
[(0, 1),
(1, 2),
(3, 1),
(4, 4),
(11, 5),
(12, 1),
(13, 1),
(14, 3),
(15, 6),
(16, 1),
(21, 1),
(23, 2),
(28, 1),
(29, 1),
(30, 1),
(36, 1),
(39, 1),
(44, 1),
(50, 1),
(53, 1),
(58, 1),
(59, 1),
(65, 1)],
[(0, 2),
(1, 1),
(4, 3),
(11, 1),
(14, 3),
(15, 2),
(18, 7),
(23, 3),
(26, 1),
(30, 1),
(31, 1),
(34, 1),
(38, 2),
(42, 1),
(59, 1),
(63, 1),
(66, 1)],
[(0, 1), (1, 1), (22, 5), (23, 3), (28, 4), (41, 1)],
[(0, 2), (1, 1), (9, 1), (22, 4), (23, 1), (28, 2), (82, 1)],
[(3, 1),
(4, 4),
(7, 1),
(10, 1),
(11, 1),
(13, 2),
(14, 3),
(15, 4),
(16, 1),
(18, 6),
(21, 2),
(23, 1),
(29, 1),
(30, 2),
(32, 1),
(35, 1),
(36, 1),
(39, 1),
(44, 1),
(53, 1),
(58, 1),
(62, 1),
(67, 1),
(92, 1)],
[(4, 5),
(11, 1),
(14, 1),
(15, 3),
(16, 1),
(18, 4),
(21, 2),
(23, 4),
(25, 2),
(30, 2),
(31, 4),
(32, 2),
(35, 1),
(36, 2),
(38, 1),
(39, 1),
(41, 1),
(44, 3),
(51, 1),
(59, 1),
(62, 1),
(67, 1),
(69, 1)],
[(0, 3),
(1, 2),
(9, 1),
(14, 2),
(19, 1),
(22, 1),
(23, 2),
(35, 1),
(67, 1)],
[(0, 4), (1, 1), (7, 2), (22, 2)],
[(0, 6), (1, 5), (7, 2), (9, 2), (16, 2), (22, 3), (28, 2)],
[(0, 3), (1, 1), (9, 1), (28, 1), (73, 1)],
[(4, 1),
(7, 1),
(8, 1),
(11, 1),
(12, 1),
(19, 1),
(23, 3),
(24, 1),
(28, 2),
(71, 1),
(85, 1)],
[(0, 2),
(4, 4),
(14, 3),
(15, 3),
(18, 1),
(26, 1),
(28, 1),
(29, 1),
(35, 1),
(39, 1),
(44, 1),
(58, 1),
(60, 1),
(63, 1),
(65, 1),
(67, 1)],
[(6, 1),
(9, 1),
(11, 1),
(12, 1),
(13, 1),
(18, 1),
(21, 1),
(22, 3),
(23, 1),
(28, 1),
(39, 1),
(44, 1),
(50, 1),
(56, 2),
(70, 1),
(82, 1)],
[(0, 1), (1, 1), (15, 1), (19, 1), (22, 14), (73, 1)],
[(0, 3),
(2, 6),
(4, 1),
(8, 1),
(17, 1),
(22, 20),
(45, 2),
(56, 1),
(68, 1),
(80, 1),
(82, 1),
(85, 1)],
[(0, 1),
(1, 1),
(7, 1),
(18, 1),
(20, 1),
(22, 4),
(23, 1),
(28, 2),
(34, 2),
(39, 1),
(45, 1),
(56, 1),
(57, 2),
(63, 1),
(68, 3)],
[(0, 2),
(1, 1),
(3, 1),
(4, 5),
(6, 3),
(7, 1),
(9, 1),
(11, 1),
(12, 2),
(13, 1),
(14, 5),
(15, 9),
(18, 2),
(19, 1),
(21, 2),
(22, 3),
(23, 4),
(25, 1),
(27, 1),
(28, 1),
(29, 1),
(30, 1),
(32, 1),
(33, 1),
(36, 1),
(38, 2),
(44, 1),
(51, 1),
(53, 2),
(56, 2),
(58, 1),
(59, 1),
(65, 1),
(67, 1),
(71, 1)],
[(0, 3),
(2, 1),
(11, 1),
(19, 2),
(22, 9),
(23, 1),
(26, 1),
(28, 4),
(50, 1),
(56, 1)],
[(0, 3),
(1, 2),
(4, 1),
(5, 2),
(11, 1),
(13, 7),
(14, 6),
(15, 1),
(16, 1),
(24, 1),
(28, 1),
(29, 1),
(30, 1),
(35, 1),
(37, 1),
(44, 3),
(54, 1),
(72, 1),
(87, 1),
(97, 1)],
[(5, 1), (14, 2), (15, 1), (16, 1), (23, 1), (28, 2), (36, 1), (44, 1)],
[(0, 1),
(3, 1),
(4, 9),
(5, 1),
(7, 1),
(10, 1),
(11, 1),
(12, 2),
(13, 1),
(14, 3),
(15, 7),
(18, 15),
(21, 4),
(22, 1),
(23, 8),
(26, 2),
(27, 2),
(29, 2),
(30, 1),
(31, 2),
(35, 1),
(36, 1),
(38, 13),
(39, 2),
(41, 1),
(44, 1),
(51, 1),
(52, 1),
(57, 8),
(58, 1),
(60, 1),
(62, 3),
(63, 2),
(65, 2),
(70, 1)],
[(0, 1),
(1, 1),
(9, 1),
(16, 1),
(21, 1),
(22, 1),
(28, 1),
(41, 1),
(48, 1)],
[(0, 1), (11, 1), (22, 2), (28, 2), (45, 1), (65, 1)],
[(0, 2), (1, 1), (17, 4), (22, 7), (23, 1), (26, 1), (28, 4), (70, 1)],
[(0, 1),
(1, 1),
(4, 1),
(5, 2),
(6, 1),
(7, 1),
(10, 1),
(11, 2),
(14, 3),
(15, 1),
(21, 1),
(23, 3),
(24, 1),
(28, 1),
(38, 1),
(39, 1)],
[(0, 1), (28, 1)],
[(0, 1), (11, 1), (15, 1), (22, 1), (28, 1)],
[(0, 2), (1, 1), (4, 1), (11, 1), (19, 1), (22, 2), (56, 1), (65, 1)],
[(0, 1),
(1, 1),
(3, 1),
(4, 3),
(9, 1),
(11, 1),
(12, 1),
(13, 1),
(14, 2),
(15, 9),
(18, 2),
(21, 2),
(22, 4),
(23, 1),
(28, 3),
(29, 1),
(30, 1),
(31, 1),
(32, 1),
(35, 1),
(36, 1),
(44, 1),
(58, 2),
(59, 1),
(63, 1),
(69, 1),
(71, 1),
(87, 1),
(93, 1)],
[(0, 1), (2, 2), (76, 1)],
[(0, 2), (1, 1), (22, 2), (23, 1), (48, 1)],
[(0, 2),
(1, 1),
(4, 3),
(5, 1),
(9, 1),
(10, 1),
(11, 2),
(12, 1),
(14, 2),
(15, 5),
(16, 1),
(18, 1),
(21, 3),
(22, 3),
(23, 3),
(26, 1),
(28, 1),
(39, 1),
(44, 1),
(51, 1),
(63, 1),
(65, 1),
(69, 1)],
[(0, 1), (12, 1), (15, 1), (18, 2), (23, 3), (27, 1)],
[(0, 3),
(1, 1),
(4, 3),
(9, 2),
(10, 1),
(11, 1),
(13, 1),
(14, 2),
(15, 3),
(16, 1),
(18, 3),
(20, 1),
(22, 1),
(23, 4),
(26, 3),
(27, 2),
(28, 2),
(30, 1),
(33, 1),
(35, 3),
(38, 1),
(39, 1),
(44, 1),
(54, 1),
(66, 1),
(67, 1),
(72, 1),
(83, 1)],
[(11, 1),
(14, 1),
(18, 1),
(21, 1),
(22, 1),
(23, 1),
(26, 1),
(28, 1),
(36, 1),
(44, 1),
(62, 1),
(69, 1)],
[(0, 4),
(1, 1),
(3, 1),
(4, 37),
(7, 3),
(8, 2),
(9, 1),
(11, 7),
(12, 3),
(14, 27),
(15, 18),
(16, 1),
(18, 52),
(19, 2),
(20, 1),
(21, 7),
(22, 2),
(23, 32),
(25, 2),
(27, 10),
(28, 1),
(29, 2),
(30, 5),
(31, 7),
(33, 2),
(34, 5),
(35, 21),
(36, 2),
(37, 2),
(38, 25),
(39, 1),
(42, 1),
(43, 5),
(44, 1),
(49, 2),
(51, 9),
(52, 6),
(53, 8),
(56, 3),
(57, 5),
(58, 2),
(60, 6),
(62, 6),
(66, 8),
(67, 1),
(69, 5),
(71, 1),
(75, 3),
(84, 1),
(85, 3),
(86, 2),
(90, 1),
(91, 1),
(98, 1),
(99, 1),
(100, 2)],
[(4, 1),
(5, 1),
(10, 1),
(11, 1),
(13, 3),
(18, 2),
(21, 2),
(23, 1),
(44, 1),
(58, 1)],
[(0, 2),
(4, 3),
(11, 1),
(18, 2),
(19, 3),
(23, 3),
(33, 2),
(34, 4),
(42, 4),
(45, 1),
(46, 1),
(69, 2),
(101, 1)],
[(23, 1), (28, 1)],
[(0, 4), (4, 1), (11, 2), (14, 1), (15, 1), (59, 1)],
[(11, 1), (15, 1), (16, 1), (21, 1), (23, 1), (27, 1)],
[(0, 3),
(1, 2),
(4, 2),
(7, 1),
(9, 1),
(14, 6),
(15, 2),
(23, 2),
(24, 1),
(27, 2),
(28, 1),
(29, 1),
(30, 1),
(41, 1),
(44, 1),
(48, 1),
(56, 1),
(61, 1),
(63, 1),
(68, 1),
(71, 1),
(74, 2)],
[(0, 2), (1, 1), (11, 1), (15, 1), (18, 1), (22, 3), (65, 2), (71, 1)],
[(0, 2),
(5, 2),
(13, 1),
(14, 1),
(23, 1),
(24, 1),
(26, 1),
(28, 1),
(29, 1),
(38, 2),
(44, 2)],
[(2, 1),
(3, 1),
(4, 3),
(7, 6),
(12, 1),
(13, 1),
(14, 2),
(16, 2),
(18, 1),
(20, 1),
(23, 1),
(27, 1),
(29, 1),
(31, 1),
(34, 1),
(37, 1),
(39, 1),
(44, 2),
(50, 1),
(51, 2),
(53, 1),
(58, 1),
(63, 1),
(64, 1),
(66, 1),
(67, 1),
(82, 1),
(87, 1)],
[(1, 1), (28, 1)],
[(0, 1), (1, 1), (22, 2), (102, 1)],
[(0, 4),
(4, 25),
(6, 1),
(7, 3),
(8, 1),
(11, 2),
(12, 1),
(13, 1),
(14, 13),
(15, 15),
(18, 20),
(19, 1),
(20, 2),
(21, 4),
(23, 12),
(24, 1),
(27, 3),
(28, 3),
(29, 1),
(30, 1),
(31, 2),
(33, 4),
(35, 16),
(36, 1),
(38, 15),
(39, 2),
(42, 3),
(43, 7),
(44, 2),
(49, 2),
(51, 2),
(52, 1),
(53, 4),
(54, 1),
(57, 1),
(58, 3),
(59, 1),
(61, 2),
(62, 3),
(64, 4),
(67, 1),
(69, 3),
(70, 5),
(71, 1),
(72, 1),
(75, 3),
(78, 1),
(83, 1),
(93, 4),
(98, 1)],
[(5, 1), (13, 1), (15, 1), (22, 5), (28, 1), (44, 1)],
[(0, 1), (22, 5), (28, 1), (65, 1), (73, 1)],
[(0, 5),
(1, 2),
(4, 3),
(5, 1),
(8, 1),
(9, 1),
(10, 1),
(11, 1),
(13, 1),
(14, 2),
(16, 1),
(18, 2),
(19, 1),
(21, 1),
(22, 16),
(23, 4),
(28, 3),
(30, 2),
(32, 1),
(48, 1),
(64, 2),
(67, 1),
(71, 1),
(82, 1),
(87, 1),
(92, 1),
(93, 1),
(103, 1)],
[(0, 2), (7, 1), (11, 1), (12, 1), (17, 1), (28, 1), (63, 1)],
[(0, 1), (1, 1), (28, 2), (48, 1), (73, 1)],
[(0, 1), (5, 5), (11, 1), (14, 1), (15, 1), (28, 2), (29, 1)],
[(0, 1), (11, 1), (23, 1), (49, 1), (58, 1), (70, 1), (71, 3)],
[(28, 3)],
[(0, 2),
(2, 4),
(12, 1),
(14, 3),
(15, 2),
(18, 1),
(19, 1),
(21, 1),
(22, 3),
(23, 1),
(28, 1),
(44, 2),
(50, 1)],
[(1, 1),
(4, 1),
(5, 5),
(6, 1),
(14, 1),
(15, 4),
(23, 2),
(28, 1),
(35, 1),
(63, 1)],
[(0, 2),
(1, 3),
(4, 1),
(9, 1),
(19, 1),
(22, 3),
(23, 2),
(27, 1),
(28, 4),
(42, 1),
(44, 1),
(45, 1),
(50, 1),
(65, 1),
(89, 1)],
[(0, 1),
(1, 1),
(3, 3),
(4, 2),
(5, 1),
(7, 1),
(11, 2),
(14, 2),
(15, 1),
(18, 1),
(22, 3),
(28, 1),
(36, 1),
(38, 1),
(44, 1),
(52, 1),
(65, 1),
(99, 1)],
[(0, 2), (14, 1), (28, 1)],
[(0, 1),
(3, 1),
(4, 11),
(7, 2),
(10, 1),
(11, 3),
(12, 1),
(13, 1),
(14, 7),
(15, 12),
(18, 10),
(19, 2),
(21, 3),
(22, 3),
(23, 2),
(24, 1),
(26, 2),
(28, 1),
(36, 1),
(38, 2),
(44, 3),
(50, 1),
(51, 1),
(57, 1),
(68, 1),
(94, 1)],
[(0, 1), (19, 1), (22, 5), (44, 1)],
[(0, 2),
(1, 1),
(14, 2),
(18, 2),
(19, 2),
(22, 3),
(23, 1),
(27, 1),
(28, 1),
(37, 1),
(48, 1),
(70, 1)],
[(1, 1), (4, 1), (56, 1)],
[(0, 1), (14, 1), (15, 4), (18, 1), (21, 1), (23, 1), (28, 1)],
[(6, 1),
(9, 1),
(11, 1),
(12, 2),
(14, 2),
(15, 2),
(18, 1),
(19, 1),
(22, 3),
(28, 1),
(56, 1),
(59, 1),
(67, 1)],
[(0, 1),
(1, 1),
(9, 1),
(11, 1),
(14, 1),
(22, 4),
(28, 1),
(34, 1),
(97, 1)],
[(1, 1),
(12, 1),
(13, 2),
(14, 6),
(22, 1),
(28, 1),
(44, 2),
(67, 1),
(70, 1),
(73, 1)],
[(0, 1),
(4, 2),
(7, 2),
(9, 1),
(11, 2),
(14, 3),
(15, 3),
(16, 1),
(17, 1),
(18, 1),
(19, 1),
(23, 1),
(29, 1),
(30, 1),
(52, 1)],
[(0, 1),
(1, 1),
(4, 1),
(9, 1),
(13, 2),
(14, 1),
(15, 1),
(19, 1),
(22, 5),
(23, 1),
(28, 3),
(94, 1)],
[(1, 1), (22, 5), (28, 3)],
[(0, 4), (1, 1), (11, 1), (14, 1), (28, 2)],
[(4, 2),
(5, 1),
(7, 3),
(8, 1),
(9, 1),
(11, 1),
(12, 2),
(15, 2),
(16, 1),
(18, 1),
(19, 1),
(21, 2),
(22, 1),
(23, 3),
(26, 2),
(38, 1),
(44, 1),
(58, 1),
(66, 1)],
[(4, 1), (7, 1), (14, 2), (15, 1)],
[(0, 1),
(4, 1),
(5, 1),
(6, 1),
(11, 3),
(12, 1),
(14, 7),
(15, 1),
(23, 1),
(24, 1),
(38, 1)],
[(4, 4),
(7, 3),
(10, 2),
(11, 2),
(12, 1),
(13, 2),
(14, 3),
(15, 7),
(18, 3),
(19, 1),
(21, 4),
(23, 4),
(25, 1),
(29, 1),
(30, 1),
(31, 1),
(32, 1),
(35, 1),
(39, 1),
(44, 3),
(53, 5),
(56, 2),
(57, 1),
(58, 2),
(60, 1),
(63, 1),
(67, 1)],
[(5, 1), (7, 1), (16, 1), (22, 6), (28, 2), (42, 1), (56, 1), (68, 1)],
[(0, 2), (2, 1), (8, 1)],
[(0, 2), (7, 1), (11, 1), (14, 3), (15, 2), (73, 1)],
[(0, 4), (9, 1), (11, 3), (14, 2)],
[(9, 1), (23, 1), (28, 2), (56, 1)],
[(0, 2), (11, 1), (14, 1), (67, 1)],
[(0, 5),
(5, 1),
(11, 1),
(14, 2),
(15, 1),
(16, 1),
(18, 1),
(21, 1),
(23, 1),
(35, 1),
(44, 1),
(67, 1),
(82, 1)],
[(0, 1),
(1, 1),
(4, 5),
(5, 1),
(9, 1),
(11, 1),
(14, 2),
(15, 3),
(18, 1),
(22, 1),
(23, 2),
(25, 1),
(28, 2),
(29, 1),
(33, 2),
(36, 1),
(38, 1),
(63, 1),
(67, 1),
(71, 1)],
[(0, 3),
(1, 4),
(5, 1),
(11, 1),
(23, 1),
(28, 1),
(29, 1),
(38, 1),
(56, 1),
(77, 1),
(93, 1)],
[(0, 1),
(1, 1),
(3, 1),
(11, 2),
(14, 2),
(15, 2),
(22, 1),
(28, 1),
(29, 1)],
[(4, 1), (6, 1), (14, 1), (15, 1), (18, 1), (21, 1), (67, 1)],
[(0, 2),
(3, 1),
(4, 1),
(12, 3),
(15, 3),
(16, 3),
(18, 3),
(21, 1),
(24, 1),
(27, 1),
(28, 1),
(29, 1),
(37, 1),
(38, 1),
(44, 1),
(50, 1),
(52, 1),
(62, 2),
(68, 1),
(71, 1)],
[(4, 4),
(7, 3),
(14, 1),
(15, 4),
(16, 2),
(18, 2),
(19, 2),
(23, 5),
(24, 1),
(26, 1),
(27, 1),
(28, 2),
(29, 1),
(30, 1),
(33, 1),
(35, 1),
(41, 1),
(44, 1),
(56, 1),
(64, 6),
(93, 1)],
[(0, 1), (13, 2), (14, 2), (15, 2), (22, 1), (28, 2), (29, 1), (36, 1)],
[(0, 3),
(1, 1),
(4, 11),
(7, 1),
(11, 4),
(14, 16),
(15, 8),
(18, 4),
(21, 8),
(24, 1),
(25, 1),
(26, 2),
(27, 1),
(30, 2),
(31, 2),
(37, 1),
(38, 23),
(49, 3),
(51, 11),
(52, 1),
(53, 4),
(57, 1),
(58, 1),
(60, 4),
(62, 2),
(66, 2),
(67, 1),
(69, 1),
(70, 1),
(83, 2),
(86, 1),
(90, 1),
(103, 1)],
[(0, 3),
(1, 1),
(4, 7),
(7, 2),
(8, 1),
(11, 1),
(14, 6),
(15, 4),
(18, 6),
(19, 1),
(20, 1),
(22, 1),
(23, 6),
(26, 1),
(27, 3),
(28, 6),
(34, 2),
(35, 2),
(38, 3),
(41, 1),
(42, 2),
(43, 1),
(44, 1),
(45, 1),
(52, 1),
(53, 1),
(57, 1),
(62, 1),
(64, 1),
(69, 1)],
[(0, 1),
(1, 2),
(19, 1),
(22, 2),
(23, 1),
(28, 1),
(56, 1),
(65, 1),
(68, 1)],
[(0, 1),
(4, 2),
(9, 1),
(11, 6),
(14, 4),
(15, 2),
(18, 6),
(29, 1),
(33, 1),
(35, 1),
(38, 1),
(52, 1),
(69, 1)],
[(1, 1), (7, 1), (12, 1), (16, 2), (29, 1)],
[(0, 1),
(1, 2),
(5, 1),
(9, 1),
(11, 1),
(16, 1),
(18, 1),
(22, 4),
(23, 2),
(28, 1)],
[(0, 2),
(4, 1),
(5, 1),
(9, 1),
(11, 1),
(12, 1),
(14, 6),
(15, 4),
(16, 1),
(21, 1),
(22, 1),
(23, 1),
(30, 1),
(36, 1),
(41, 1),
(44, 3),
(54, 1),
(56, 1),
(58, 1),
(67, 1)],
[(1, 1), (7, 1), (11, 1), (15, 1), (22, 1), (23, 1), (28, 1), (50, 1)],
[(0, 1), (1, 2), (11, 1), (22, 1), (28, 3), (85, 1)],
[(0, 1), (1, 1), (28, 1)],
[(0, 3),
(2, 1),
(15, 1),
(16, 1),
(21, 1),
(22, 5),
(28, 1),
(29, 1),
(56, 1),
(73, 1),
(92, 2)],
[(14, 1), (29, 1), (30, 1), (41, 1), (44, 2), (59, 1)],
[(0, 1)],
[(2, 1),
(4, 5),
(7, 2),
(11, 1),
(12, 1),
(15, 2),
(16, 1),
(18, 2),
(21, 1),
(22, 4),
(23, 1),
(26, 1),
(28, 2),
(31, 2),
(32, 2),
(35, 1),
(36, 1),
(38, 3),
(39, 1),
(57, 3),
(60, 1),
(62, 1),
(66, 1)],
[(0, 1),
(6, 1),
(9, 1),
(17, 3),
(18, 2),
(21, 1),
(22, 2),
(23, 1),
(28, 1),
(44, 1),
(73, 1),
(82, 1)],
[(0, 6),
(1, 1),
(3, 1),
(4, 2),
(6, 1),
(7, 1),
(9, 1),
(11, 3),
(12, 1),
(14, 8),
(15, 5),
(16, 1),
(18, 4),
(21, 1),
(22, 1),
(23, 3),
(28, 1),
(29, 1),
(30, 1),
(35, 1),
(50, 1),
(52, 1),
(53, 2),
(55, 1),
(56, 2),
(58, 2),
(63, 1),
(67, 1),
(93, 1)],
[(0, 1),
(7, 1),
(11, 2),
(14, 2),
(15, 2),
(22, 1),
(28, 4),
(29, 1),
(39, 1),
(50, 1)],
[(0, 3),
(1, 2),
(9, 2),
(11, 1),
(16, 1),
(19, 2),
(23, 2),
(26, 1),
(28, 2),
(44, 1),
(67, 1),
(93, 1),
(104, 1)],
[(4, 8),
(7, 2),
(11, 1),
(13, 2),
(14, 7),
(15, 9),
(17, 1),
(18, 14),
(19, 1),
(21, 5),
(23, 5),
(28, 1),
(29, 1),
(30, 1),
(31, 1),
(32, 1),
(35, 5),
(36, 3),
(38, 3),
(44, 2),
(53, 1),
(56, 1),
(58, 1),
(62, 1),
(63, 1)],
[(0, 2),
(1, 4),
(4, 1),
(9, 3),
(11, 3),
(14, 2),
(15, 1),
(22, 2),
(29, 1),
(34, 1),
(76, 2),
(85, 6)],
[(0, 1), (11, 1), (23, 1)],
[(9, 1), (11, 1), (14, 1), (16, 1), (22, 3)]]
In the output, the inner list represent a document, where the tuples indicate the index of the word and its frequency. For example, for the first document [(0, 1), (1, 1), (2, 2)], the 0th word is repeated once, then the 1st word repeated once, and the 2nd word repeated twice. What is the 0th word? It corresponds to the index of words in dict_
.
Then, we can implement the LDA model by creating the object and passing the required arguments to it:
Lda = gensim.models.ldamodel.LdaModel
lda_bristol = Lda(corpus= doc_term_matrix, num_topics =6, id2word =dict_, random_state=0, eval_every=None)
To generate an LDA model, we input the corpus (doc_term_matrix
) to it together with several parameters.
num_topics
: indicates the number of topics will be usedid2word
: is the vocabulary generated from thecorpora.Dictionary()
functionrandom_state
: used for reproducibility (because we need to randomly initialize some embeddings, so everytime you run the mode, it might result into different outputs. Setting up a seed value here guarantees you the models will be trained the same if input data and parameters are all the same)eval_every
: Log perplexity (an evaluation metric for the model) is estimated every that many updates. If it isNone
, the model will only be evaluated at the end of the training.
For more parameters to set up, check its official website: https://radimrehurek.com/gensim/models/ldamodel.html
We can see how the topic is represented:
lda_bristol.print_topics()
[(0,
'0.137*"\'fast_food\'" + 0.134*"\'park\'" + 0.065*"\'convenience\'" + 0.055*"\'hairdresser\'" + 0.045*"\'cafe\'" + 0.037*"\'clothes\'" + 0.032*"\'pub\'" + 0.031*"\'playground\'" + 0.030*"\'pitch\'" + 0.027*"\'school\'"'),
(1,
'0.108*"\'hairdresser\'" + 0.089*"\'fast_food\'" + 0.070*"\'pub\'" + 0.059*"\'cafe\'" + 0.057*"\'restaurant\'" + 0.050*"\'convenience\'" + 0.042*"\'pitch\'" + 0.038*"\'park\'" + 0.037*"\'car_dealership\'" + 0.031*"\'school\'"'),
(2,
'0.112*"\'pitch\'" + 0.067*"\'cafe\'" + 0.065*"\'pub\'" + 0.059*"\'school\'" + 0.059*"\'park\'" + 0.056*"\'restaurant\'" + 0.038*"\'fast_food\'" + 0.034*"\'hairdresser\'" + 0.034*"\'clothes\'" + 0.029*"\'playground\'"'),
(3,
'0.126*"\'restaurant\'" + 0.095*"\'cafe\'" + 0.084*"\'fast_food\'" + 0.071*"\'hairdresser\'" + 0.054*"\'pub\'" + 0.044*"\'clothes\'" + 0.040*"\'bar\'" + 0.027*"\'supermarket\'" + 0.026*"\'park\'" + 0.022*"\'beauty_shop\'"'),
(4,
'0.073*"\'park\'" + 0.071*"\'restaurant\'" + 0.068*"\'school\'" + 0.064*"\'pub\'" + 0.061*"\'fast_food\'" + 0.055*"\'playground\'" + 0.055*"\'convenience\'" + 0.055*"\'cafe\'" + 0.054*"\'hairdresser\'" + 0.041*"\'pitch\'"'),
(5,
'0.237*"\'pitch\'" + 0.062*"\'school\'" + 0.058*"\'convenience\'" + 0.049*"\'hairdresser\'" + 0.042*"\'park\'" + 0.038*"\'fast_food\'" + 0.033*"\'pub\'" + 0.033*"\'restaurant\'" + 0.029*"\'playground\'" + 0.027*"\'cafe\'"')]
As can be seen, the 6 topics (listed as 0 - 5) are reprsented as a distribution of words (place types here), and each of the 105 unique words (palce types) is given weights based on the topics. In other words, it implies which of the words (place types) is more likely to dominate the topic. For example, topic 1 is moare about “fast food” and “park” while topic 2 is more about “hairdresser” and topic 5 is about “pitch”.
We can also only show the top 5 words (place types) for each topic by using code:
lda_bristol.print_topics(num_topics = 6, num_words=5)
[(0,
'0.137*"\'fast_food\'" + 0.134*"\'park\'" + 0.065*"\'convenience\'" + 0.055*"\'hairdresser\'" + 0.045*"\'cafe\'"'),
(1,
'0.108*"\'hairdresser\'" + 0.089*"\'fast_food\'" + 0.070*"\'pub\'" + 0.059*"\'cafe\'" + 0.057*"\'restaurant\'"'),
(2,
'0.112*"\'pitch\'" + 0.067*"\'cafe\'" + 0.065*"\'pub\'" + 0.059*"\'school\'" + 0.059*"\'park\'"'),
(3,
'0.126*"\'restaurant\'" + 0.095*"\'cafe\'" + 0.084*"\'fast_food\'" + 0.071*"\'hairdresser\'" + 0.054*"\'pub\'"'),
(4,
'0.073*"\'park\'" + 0.071*"\'restaurant\'" + 0.068*"\'school\'" + 0.064*"\'pub\'" + 0.061*"\'fast_food\'"'),
(5,
'0.237*"\'pitch\'" + 0.062*"\'school\'" + 0.058*"\'convenience\'" + 0.049*"\'hairdresser\'" + 0.042*"\'park\'"')]
Next, we can check how each document (region) are represented using the topic:
count = 0
for i in lda_bristol[doc_term_matrix]:
print("doc: ", count, i)
count+=1
doc: 0 [(0, 0.83217597), (1, 0.033423353), (2, 0.03352079), (3, 0.03337131), (4, 0.033680912), (5, 0.033827636)]
doc: 1 [(1, 0.9699462)]
doc: 2 [(0, 0.94747126), (1, 0.010519896), (2, 0.010488585), (3, 0.010489068), (4, 0.010525895), (5, 0.01050526)]
doc: 3 [(0, 0.014079193), (1, 0.29682362), (2, 0.014034042), (3, 0.013978785), (4, 0.014052106), (5, 0.6470323)]
doc: 4 [(2, 0.7453384), (5, 0.21913613)]
doc: 5 [(0, 0.5495233), (1, 0.40251347), (2, 0.011982679), (3, 0.011978997), (4, 0.011991423), (5, 0.012010168)]
doc: 6 [(0, 0.015247152), (1, 0.92353535), (2, 0.01531361), (3, 0.015313374), (4, 0.015321066), (5, 0.01526944)]
doc: 7 [(3, 0.9931071)]
doc: 8 [(0, 0.0105504235), (1, 0.9473252), (2, 0.010540981), (3, 0.010473925), (4, 0.010549425), (5, 0.01056005)]
doc: 9 [(0, 0.5802037), (1, 0.01679723), (2, 0.01682109), (3, 0.016754672), (4, 0.016824938), (5, 0.35259834)]
doc: 10 [(1, 0.9634004)]
doc: 11 [(1, 0.15240093), (3, 0.48479685), (4, 0.35438988)]
doc: 12 [(0, 0.19156682), (1, 0.47174674), (3, 0.33012822)]
doc: 13 [(0, 0.041998807), (1, 0.04183528), (2, 0.042015024), (3, 0.04176866), (4, 0.042039167), (5, 0.79034305)]
doc: 14 [(3, 0.43310913), (4, 0.54282767)]
doc: 15 [(0, 0.021173846), (1, 0.020979095), (2, 0.021020494), (3, 0.020898085), (4, 0.021064946), (5, 0.89486355)]
doc: 16 [(0, 0.05592313), (1, 0.056297045), (2, 0.056242563), (3, 0.055985115), (4, 0.71929425), (5, 0.05625785)]
doc: 17 [(4, 0.7117077), (5, 0.26787984)]
doc: 18 [(1, 0.97662306)]
doc: 19 [(0, 0.20986126), (1, 0.48722824), (4, 0.2948858)]
doc: 20 [(0, 0.015258109), (1, 0.9235579), (2, 0.015270039), (3, 0.015304093), (4, 0.015313634), (5, 0.015296179)]
doc: 21 [(0, 0.021061739), (1, 0.021058284), (2, 0.021092903), (3, 0.021000056), (4, 0.8945658), (5, 0.02122121)]
doc: 22 [(0, 0.0112183215), (1, 0.94395834), (2, 0.011209111), (3, 0.0111712), (4, 0.011196391), (5, 0.0112466235)]
doc: 23 [(0, 0.0419544), (1, 0.79038066), (2, 0.04186972), (3, 0.041971896), (4, 0.04197297), (5, 0.04185034)]
doc: 24 [(0, 0.3966683), (1, 0.56587833)]
doc: 25 [(0, 0.10900296), (2, 0.8616712)]
doc: 26 [(0, 0.9505633)]
doc: 27 [(0, 0.6397539), (4, 0.32659358)]
doc: 28 [(0, 0.9234856), (1, 0.015348032), (2, 0.015269217), (3, 0.015277718), (4, 0.015320138), (5, 0.015299315)]
doc: 29 [(0, 0.9234167), (1, 0.015268485), (2, 0.015328541), (3, 0.015233515), (4, 0.015328568), (5, 0.015424265)]
doc: 30 [(1, 0.10323222), (2, 0.75720674), (3, 0.13234371)]
doc: 31 [(5, 0.96138394)]
doc: 32 [(0, 0.75392085), (5, 0.21686251)]
doc: 33 [(0, 0.9064717), (1, 0.018733587), (2, 0.0187056), (3, 0.018651485), (4, 0.018765299), (5, 0.018672269)]
doc: 34 [(0, 0.42057648), (2, 0.1394032), (3, 0.42513642)]
doc: 35 [(0, 0.47034827), (2, 0.4942027)]
doc: 36 [(0, 0.37939805), (1, 0.5592075), (2, 0.015399452), (3, 0.0152483545), (4, 0.015329889), (5, 0.015416824)]
doc: 37 [(1, 0.82384545), (3, 0.15888834)]
doc: 38 [(0, 0.58102053), (1, 0.083692044), (2, 0.083904475), (3, 0.0835684), (4, 0.08408199), (5, 0.08373257)]
doc: 39 [(0, 0.016821997), (1, 0.36672425), (2, 0.016779823), (3, 0.016748136), (4, 0.016805448), (5, 0.5661203)]
doc: 40 [(0, 0.33030936), (5, 0.6322819)]
doc: 41 [(0, 0.7179962), (1, 0.05653706), (2, 0.056529474), (3, 0.05626602), (4, 0.056618016), (5, 0.05605324)]
doc: 42 [(5, 0.9533654)]
doc: 43 [(0, 0.010577291), (1, 0.010562004), (2, 0.01050544), (3, 0.010504081), (4, 0.010525369), (5, 0.9473258)]
doc: 44 [(3, 0.59086114), (4, 0.38508675)]
doc: 45 [(1, 0.6228953), (5, 0.34167024)]
doc: 46 [(0, 0.18574491), (1, 0.5077382), (5, 0.28909826)]
doc: 47 [(0, 0.01527985), (1, 0.0153401345), (2, 0.015229504), (3, 0.0152205005), (4, 0.015241117), (5, 0.9236889)]
doc: 48 [(4, 0.95048773)]
doc: 49 [(0, 0.018689334), (1, 0.9065497), (2, 0.0186589), (3, 0.01868378), (4, 0.018718937), (5, 0.018699413)]
doc: 50 [(0, 0.01288401), (1, 0.012881098), (2, 0.012910637), (3, 0.012841318), (4, 0.012920735), (5, 0.93556225)]
doc: 51 [(0, 0.033626616), (1, 0.83221865), (2, 0.033518806), (3, 0.033577878), (4, 0.03354657), (5, 0.033511527)]
doc: 52 [(4, 0.9772423)]
doc: 53 [(0, 0.08334324), (1, 0.08334228), (2, 0.083343625), (3, 0.5832848), (4, 0.08334296), (5, 0.083343126)]
doc: 54 [(0, 0.90625477), (1, 0.018725852), (2, 0.018812835), (3, 0.018616742), (4, 0.01874055), (5, 0.018849256)]
doc: 55 [(0, 0.021073613), (1, 0.02100332), (2, 0.021019064), (3, 0.020926744), (4, 0.02105007), (5, 0.89492714)]
doc: 56 [(0, 0.0421637), (1, 0.04201479), (2, 0.042009104), (3, 0.041954547), (4, 0.04212894), (5, 0.78972894)]
doc: 57 [(0, 0.01867386), (1, 0.018603817), (2, 0.018683227), (3, 0.018581433), (4, 0.018658018), (5, 0.9067997)]
doc: 58 [(0, 0.24652027), (1, 0.011210666), (2, 0.011287659), (3, 0.011193128), (4, 0.70847946), (5, 0.011308781)]
doc: 59 [(1, 0.06334552), (4, 0.922001)]
doc: 60 [(0, 0.3892188), (1, 0.5989765)]
doc: 61 [(0, 0.9063315), (1, 0.0187126), (2, 0.018739639), (3, 0.018624835), (4, 0.01870965), (5, 0.018881759)]
doc: 62 [(0, 0.016811784), (1, 0.016865281), (2, 0.016831316), (3, 0.016815526), (4, 0.9158751), (5, 0.016801054)]
doc: 63 [(1, 0.9663285)]
doc: 64 [(0, 0.35368788), (1, 0.62138885)]
doc: 65 [(0, 0.32114798), (5, 0.6467774)]
doc: 66 [(0, 0.012014329), (1, 0.011995733), (2, 0.012052908), (3, 0.011980748), (4, 0.0120557435), (5, 0.9399006)]
doc: 67 [(4, 0.9709791)]
doc: 68 [(5, 0.97948265)]
doc: 69 [(0, 0.6576848), (5, 0.3068854)]
doc: 70 [(1, 0.06028744), (5, 0.9022441)]
doc: 71 [(3, 0.9617652)]
doc: 72 [(0, 0.010494314), (1, 0.94755286), (2, 0.0104817515), (3, 0.010475555), (4, 0.010497967), (5, 0.010497536)]
doc: 73 [(0, 0.041996334), (1, 0.042027324), (2, 0.78932816), (3, 0.042121816), (4, 0.04223653), (5, 0.042289846)]
doc: 74 [(0, 0.4964167), (1, 0.010490433), (2, 0.010525827), (3, 0.010451756), (4, 0.0105173355), (5, 0.461598)]
doc: 75 [(0, 0.021169052), (1, 0.02093616), (2, 0.021022046), (3, 0.020891258), (4, 0.021063143), (5, 0.8949183)]
doc: 76 [(0, 0.018785741), (1, 0.018716522), (2, 0.018691264), (3, 0.018651832), (4, 0.018748464), (5, 0.90640616)]
doc: 77 [(0, 0.024107426), (1, 0.024092905), (2, 0.024069294), (3, 0.024112498), (4, 0.8795775), (5, 0.024040336)]
doc: 78 [(0, 0.02396677), (1, 0.023943976), (2, 0.023985198), (3, 0.023893578), (4, 0.88016886), (5, 0.024041627)]
doc: 79 [(0, 0.4640192), (5, 0.5022988)]
doc: 80 [(0, 0.0211191), (1, 0.021120423), (2, 0.021026827), (3, 0.021018997), (4, 0.894659), (5, 0.021055674)]
doc: 81 [(0, 0.024260448), (1, 0.8793815), (2, 0.024108082), (3, 0.023943907), (4, 0.02415126), (5, 0.024154807)]
doc: 82 [(0, 0.28182244), (5, 0.687575)]
doc: 83 [(0, 0.013948811), (1, 0.013964614), (2, 0.013984908), (3, 0.013937109), (4, 0.013980689), (5, 0.9301838)]
doc: 84 [(0, 0.95795405)]
doc: 85 [(0, 0.014008018), (1, 0.014062392), (2, 0.014014296), (3, 0.0139524825), (4, 0.014005432), (5, 0.9299574)]
doc: 86 [(0, 0.01867866), (1, 0.018671244), (2, 0.01868873), (3, 0.018631762), (4, 0.018708404), (5, 0.9066212)]
doc: 87 [(0, 0.021064535), (1, 0.020948656), (2, 0.021076178), (3, 0.020881927), (4, 0.89483035), (5, 0.021198368)]
doc: 88 [(0, 0.08879313), (1, 0.5423661), (3, 0.36012414)]
doc: 89 [(0, 0.014018333), (1, 0.92989856), (2, 0.013996465), (3, 0.014062178), (4, 0.014035775), (5, 0.013988684)]
doc: 90 [(0, 0.024046516), (1, 0.024001658), (2, 0.024101526), (3, 0.02392894), (4, 0.024063664), (5, 0.8798577)]
doc: 91 [(0, 0.011223428), (1, 0.01124866), (2, 0.011226683), (3, 0.943884), (4, 0.011204827), (5, 0.011212417)]
doc: 92 [(0, 0.041950006), (1, 0.041874222), (2, 0.04209734), (3, 0.04174705), (4, 0.042265154), (5, 0.79006624)]
doc: 93 [(1, 0.9759825)]
doc: 94 [(0, 0.011213961), (1, 0.011174938), (2, 0.011207387), (3, 0.011138739), (4, 0.011252615), (5, 0.94401234)]
doc: 95 [(0, 0.7896238), (1, 0.041925862), (2, 0.04218879), (3, 0.04181452), (4, 0.042247158), (5, 0.042199828)]
doc: 96 [(0, 0.25610042), (1, 0.014067966), (2, 0.21105102), (3, 0.014058795), (4, 0.014074898), (5, 0.49064693)]
doc: 97 [(0, 0.015248661), (1, 0.0152765), (2, 0.015233273), (3, 0.015176626), (4, 0.015232912), (5, 0.923832)]
doc: 98 [(1, 0.7172456), (4, 0.26102555)]
doc: 99 [(0, 0.012026619), (1, 0.01197395), (2, 0.012021277), (3, 0.011938577), (4, 0.0120150475), (5, 0.94002455)]
doc: 100 [(0, 0.26893362), (1, 0.011203375), (2, 0.6862145), (3, 0.011181182), (4, 0.011210649), (5, 0.0112566585)]
doc: 101 [(1, 0.57775193), (5, 0.40118617)]
doc: 102 [(0, 0.02401939), (1, 0.024124885), (2, 0.02402478), (3, 0.023985105), (4, 0.8798038), (5, 0.024042035)]
doc: 103 [(0, 0.010511529), (1, 0.94743043), (2, 0.010506749), (3, 0.010501352), (4, 0.010532884), (5, 0.010517088)]
doc: 104 [(0, 0.012037567), (1, 0.9398515), (2, 0.0120121585), (3, 0.011993215), (4, 0.0120630255), (5, 0.012042528)]
doc: 105 [(0, 0.028054547), (1, 0.028047942), (2, 0.028201913), (3, 0.027930774), (4, 0.85939765), (5, 0.028367158)]
doc: 106 [(1, 0.9835291)]
doc: 107 [(0, 0.0153382635), (1, 0.015288379), (2, 0.015297467), (3, 0.015275782), (4, 0.9234789), (5, 0.015321237)]
doc: 108 [(0, 0.29619956), (3, 0.69059914)]
doc: 109 [(4, 0.42108235), (5, 0.54835486)]
doc: 110 [(0, 0.05575243), (1, 0.055796694), (2, 0.05610549), (3, 0.055654056), (4, 0.056028377), (5, 0.72066295)]
doc: 111 [(0, 0.8800459), (1, 0.0239903), (2, 0.02399281), (3, 0.023915006), (4, 0.024053225), (5, 0.024002785)]
doc: 112 [(0, 0.19042303), (1, 0.3799925), (5, 0.41558063)]
doc: 113 [(0, 0.016708503), (1, 0.016716396), (2, 0.016793879), (3, 0.016681613), (4, 0.016742788), (5, 0.9163568)]
doc: 114 [(0, 0.028290857), (1, 0.028185256), (2, 0.028139958), (3, 0.028093625), (4, 0.028050905), (5, 0.8592394)]
doc: 115 [(0, 0.015283785), (1, 0.015258944), (2, 0.015261443), (3, 0.015212201), (4, 0.015226945), (5, 0.92375666)]
doc: 116 [(1, 0.9759489)]
doc: 117 [(0, 0.028060684), (1, 0.8601383), (2, 0.027938543), (3, 0.027931118), (4, 0.02794689), (5, 0.027984433)]
doc: 118 [(0, 0.02402835), (1, 0.8803231), (2, 0.023921782), (3, 0.023892265), (4, 0.023880765), (5, 0.02395373)]
doc: 119 [(1, 0.059910275), (4, 0.8052879), (5, 0.12806891)]
doc: 120 [(0, 0.01200248), (1, 0.0120085385), (2, 0.012029742), (3, 0.011995779), (4, 0.93996805), (5, 0.011995415)]
doc: 121 [(1, 0.96185166)]
doc: 122 [(1, 0.6876246), (2, 0.12054685), (3, 0.18381487)]
doc: 123 [(0, 0.86380005), (5, 0.110335164)]
doc: 124 [(1, 0.95060456)]
doc: 125 [(1, 0.8214463), (3, 0.16751465)]
doc: 126 [(0, 0.35699138), (1, 0.011997002), (2, 0.012054889), (3, 0.011997836), (4, 0.012003806), (5, 0.594955)]
doc: 127 [(0, 0.83103865), (1, 0.033561483), (2, 0.033882987), (3, 0.033418506), (4, 0.03364355), (5, 0.034454804)]
doc: 128 [(0, 0.8464992), (1, 0.010536389), (2, 0.11134751), (3, 0.010504723), (4, 0.010544508), (5, 0.01056767)]
doc: 129 [(0, 0.015286526), (1, 0.015262246), (2, 0.015305827), (3, 0.01522302), (4, 0.01532258), (5, 0.9235998)]
doc: 130 [(0, 0.5510388), (1, 0.0209471), (2, 0.021063846), (3, 0.020881444), (4, 0.020984478), (5, 0.36508435)]
doc: 131 [(0, 0.5810676), (1, 0.08369055), (2, 0.08389685), (3, 0.08356772), (4, 0.084046334), (5, 0.083730936)]
doc: 132 [(5, 0.96003604)]
doc: 133 [(1, 0.98131394)]
doc: 134 [(4, 0.67479074), (5, 0.28981197)]
doc: 135 [(2, 0.9533968)]
doc: 136 [(1, 0.4275891), (4, 0.33731458), (5, 0.21768194)]
doc: 137 [(0, 0.01867153), (1, 0.018701145), (2, 0.018775448), (3, 0.018685106), (4, 0.018796815), (5, 0.90637)]
doc: 138 [(0, 0.018706484), (1, 0.18930066), (2, 0.01877248), (3, 0.018737), (4, 0.018764945), (5, 0.7357185)]
doc: 139 [(0, 0.028080426), (1, 0.85968137), (2, 0.0280047), (3, 0.027928285), (4, 0.028143838), (5, 0.028161345)]
doc: 140 [(0, 0.97711384)]
doc: 141 [(0, 0.015302059), (1, 0.015236695), (2, 0.015397071), (3, 0.015252001), (4, 0.015293278), (5, 0.9235189)]
doc: 142 [(0, 0.018572135), (1, 0.01857683), (2, 0.01863579), (3, 0.018534226), (4, 0.018591097), (5, 0.90708995)]
doc: 143 [(0, 0.16197324), (1, 0.79601276), (2, 0.010481149), (3, 0.010480452), (4, 0.010534917), (5, 0.0105174845)]
doc: 144 [(0, 0.024107154), (1, 0.024034746), (2, 0.02406335), (3, 0.02393932), (4, 0.024137026), (5, 0.8797184)]
doc: 145 [(0, 0.02106492), (1, 0.02094805), (2, 0.021056509), (3, 0.020885939), (4, 0.02113255), (5, 0.89491206)]
doc: 146 [(0, 0.27551916), (5, 0.70343757)]
doc: 147 [(0, 0.018706273), (1, 0.4126156), (2, 0.018774), (3, 0.018622406), (4, 0.5124376), (5, 0.018844126)]
doc: 148 [(0, 0.0153514035), (1, 0.015369818), (2, 0.015340448), (3, 0.015421078), (4, 0.015352685), (5, 0.92316455)]
doc: 149 [(0, 0.8309773), (1, 0.03378097), (2, 0.033857252), (3, 0.033577), (4, 0.03391653), (5, 0.03389095)]
doc: 150 [(1, 0.78416556), (4, 0.19901197)]
doc: 151 [(3, 0.701907), (4, 0.2777058)]
doc: 152 [(0, 0.010501946), (1, 0.0105079785), (2, 0.010543501), (3, 0.010479749), (4, 0.010541955), (5, 0.9474249)]
doc: 153 [(0, 0.012959775), (1, 0.012907731), (2, 0.01296291), (3, 0.012869094), (4, 0.012959091), (5, 0.9353414)]
doc: 154 [(1, 0.56801945), (3, 0.41556138)]
doc: 155 [(1, 0.2870055), (3, 0.6983647)]
doc: 156 [(0, 0.011259781), (1, 0.011238356), (2, 0.011234002), (3, 0.011207973), (4, 0.94381624), (5, 0.011243668)]
doc: 157 [(0, 0.64620626), (1, 0.016760072), (2, 0.016809879), (3, 0.016729662), (4, 0.016842192), (5, 0.2866519)]
doc: 158 [(0, 0.1933009), (4, 0.47110146), (5, 0.31372866)]
doc: 159 [(0, 0.8950403), (1, 0.020948214), (2, 0.021013888), (3, 0.020887008), (4, 0.0210785), (5, 0.02103209)]
doc: 160 [(0, 0.011223569), (1, 0.94383186), (2, 0.011235257), (3, 0.011201873), (4, 0.011266462), (5, 0.011241021)]
doc: 161 [(1, 0.2953749), (3, 0.6776541)]
doc: 162 [(5, 0.9579438)]
doc: 163 [(5, 0.9581312)]
doc: 164 [(5, 0.97905815)]
doc: 165 [(4, 0.30445224), (5, 0.6675276)]
doc: 166 [(1, 0.98723495)]
doc: 167 [(5, 0.9664245)]
doc: 168 [(0, 0.97786355)]
doc: 169 [(0, 0.015311497), (1, 0.9234009), (2, 0.015318546), (3, 0.0152720995), (4, 0.015353984), (5, 0.015343044)]
doc: 170 [(3, 0.99197656)]
doc: 171 [(0, 0.18164146), (1, 0.01677703), (2, 0.016821057), (3, 0.016738677), (4, 0.016840683), (5, 0.7511811)]
doc: 172 [(0, 0.018680187), (1, 0.018625213), (2, 0.018698605), (3, 0.018585872), (4, 0.018722741), (5, 0.90668744)]
doc: 173 [(2, 0.8826539), (5, 0.08688013)]
doc: 174 [(1, 0.96339864)]
doc: 175 [(0, 0.05650424), (1, 0.05591067), (2, 0.05618685), (3, 0.05575784), (4, 0.71952575), (5, 0.056114662)]
doc: 176 [(0, 0.02823976), (1, 0.028096152), (2, 0.028028497), (3, 0.027948752), (4, 0.028124185), (5, 0.8595627)]
doc: 177 [(0, 0.015355163), (1, 0.015292865), (2, 0.0153141385), (3, 0.015261212), (4, 0.015350086), (5, 0.9234265)]
doc: 178 [(1, 0.91966945), (5, 0.06658911)]
doc: 179 [(0, 0.83250344), (1, 0.033452716), (2, 0.03342657), (3, 0.033354163), (4, 0.033547405), (5, 0.03371571)]
doc: 180 [(0, 0.44102895), (1, 0.020992612), (2, 0.021067202), (3, 0.020925498), (4, 0.021070508), (5, 0.47491524)]
doc: 181 [(1, 0.8386378), (5, 0.1440833)]
doc: 182 [(0, 0.016844327), (1, 0.64743215), (2, 0.01688067), (3, 0.28516817), (4, 0.01688017), (5, 0.016794577)]
doc: 183 [(1, 0.52020913), (2, 0.46384728)]
doc: 184 [(0, 0.012977248), (1, 0.0129985465), (2, 0.7874361), (3, 0.012978637), (4, 0.012982994), (5, 0.16062646)]
doc: 185 [(3, 0.9977314)]
doc: 186 [(0, 0.01120842), (1, 0.94395125), (2, 0.011198129), (3, 0.011230915), (4, 0.011197283), (5, 0.011214016)]
doc: 187 [(4, 0.9720186)]
doc: 188 [(0, 0.0558775), (1, 0.05613777), (2, 0.056297168), (3, 0.055941246), (4, 0.71965826), (5, 0.056088034)]
doc: 189 [(0, 0.9235985), (1, 0.015317026), (2, 0.015257439), (3, 0.015270723), (4, 0.015296273), (5, 0.015259994)]
doc: 190 [(0, 0.02409235), (1, 0.8795594), (2, 0.024106232), (3, 0.02405906), (4, 0.024115033), (5, 0.024067923)]
doc: 191 [(0, 0.9765978)]
doc: 192 [(0, 0.013009444), (1, 0.012972249), (2, 0.012952881), (3, 0.012925314), (4, 0.01296726), (5, 0.93517286)]
doc: 193 [(0, 0.9473278), (1, 0.010571014), (2, 0.010540338), (3, 0.010513432), (4, 0.010520001), (5, 0.01052745)]
doc: 194 [(0, 0.09718031), (3, 0.21432409), (4, 0.67586386)]
doc: 195 [(0, 0.055904035), (1, 0.0558215), (2, 0.056077056), (3, 0.05567088), (4, 0.7204084), (5, 0.056118093)]
doc: 196 [(0, 0.02809516), (1, 0.027895587), (2, 0.028011872), (3, 0.027835006), (4, 0.028051134), (5, 0.8601112)]
doc: 197 [(3, 0.9960314)]
doc: 198 [(0, 0.015230137), (1, 0.015287745), (2, 0.015246233), (3, 0.015206956), (4, 0.015223267), (5, 0.92380565)]
doc: 199 [(0, 0.01684851), (1, 0.016735895), (2, 0.016802067), (3, 0.016708305), (4, 0.016766094), (5, 0.9161392)]
doc: 200 [(2, 0.4629934), (5, 0.52578515)]
doc: 201 [(0, 0.018740565), (1, 0.01869888), (2, 0.01887236), (3, 0.018668018), (4, 0.90628844), (5, 0.018731741)]
doc: 202 [(0, 0.8797133), (1, 0.023961745), (2, 0.02410926), (3, 0.023883542), (4, 0.024200346), (5, 0.024131848)]
doc: 203 [(0, 0.0129685905), (1, 0.93532705), (2, 0.012926101), (3, 0.012883998), (4, 0.012948405), (5, 0.012945857)]
doc: 204 [(0, 0.75317216), (1, 0.17962399), (2, 0.016860185), (3, 0.01680023), (4, 0.01677871), (5, 0.01676468)]
doc: 205 [(0, 0.041867595), (1, 0.04190062), (2, 0.04213959), (3, 0.041778196), (4, 0.79015476), (5, 0.042159267)]
doc: 206 [(5, 0.9648826)]
doc: 207 [(1, 0.9558221)]
doc: 208 [(4, 0.82121944), (5, 0.15188536)]
doc: 209 [(1, 0.4191583), (4, 0.15517172), (5, 0.40622997)]
doc: 210 [(0, 0.8318273), (1, 0.033572998), (2, 0.03368024), (3, 0.03348718), (4, 0.03376578), (5, 0.033666488)]
doc: 211 [(1, 0.748365), (3, 0.24276981)]
doc: 212 [(0, 0.018639365), (1, 0.018604225), (2, 0.01865638), (3, 0.01857608), (4, 0.018629313), (5, 0.90689456)]
doc: 213 [(0, 0.2203332), (2, 0.5528093), (5, 0.20023774)]
doc: 214 [(0, 0.041982964), (1, 0.04222485), (2, 0.04222132), (3, 0.042046346), (4, 0.78910863), (5, 0.042415857)]
doc: 215 [(0, 0.015327006), (1, 0.9234784), (2, 0.015290041), (3, 0.015302332), (4, 0.015321929), (5, 0.015280278)]
doc: 216 [(1, 0.39675272), (5, 0.56785536)]
doc: 217 [(0, 0.012972654), (1, 0.0129037965), (2, 0.012911041), (3, 0.012885509), (4, 0.012982537), (5, 0.93534446)]
doc: 218 [(0, 0.9531834)]
doc: 219 [(1, 0.7279432), (4, 0.24275658)]
doc: 220 [(5, 0.9579276)]
doc: 221 [(0, 0.016729262), (1, 0.016727533), (2, 0.0168045), (3, 0.016689641), (4, 0.016797308), (5, 0.9162517)]
doc: 222 [(0, 0.91591686), (1, 0.016781492), (2, 0.016831987), (3, 0.016727418), (4, 0.01690011), (5, 0.016842175)]
doc: 223 [(1, 0.759018), (2, 0.21772704)]
doc: 224 [(0, 0.859697), (1, 0.028139682), (2, 0.027977502), (3, 0.028165363), (4, 0.028058406), (5, 0.027962102)]
doc: 225 [(0, 0.95796764)]
doc: 226 [(1, 0.66438895), (3, 0.32439238)]
doc: 227 [(0, 0.011157525), (1, 0.01118593), (2, 0.011238155), (3, 0.011209889), (4, 0.011254137), (5, 0.94395435)]
doc: 228 [(0, 0.83235157), (1, 0.03345184), (2, 0.033545084), (3, 0.033471122), (4, 0.033519406), (5, 0.033660926)]
doc: 229 [(0, 0.9237297), (1, 0.015296649), (2, 0.015214852), (3, 0.015254307), (4, 0.015263571), (5, 0.015240881)]
doc: 230 [(0, 0.92382663), (1, 0.015248046), (2, 0.015208541), (3, 0.015199595), (4, 0.015268072), (5, 0.015249144)]
doc: 231 [(0, 0.027966868), (1, 0.028079312), (2, 0.028103307), (3, 0.02793328), (4, 0.02813502), (5, 0.8597822)]
doc: 232 [(0, 0.86022294), (1, 0.028016564), (2, 0.027922949), (3, 0.027899949), (4, 0.028024103), (5, 0.027913528)]
doc: 233 [(0, 0.7448505), (1, 0.2196914)]
doc: 234 [(1, 0.9728189)]
doc: 235 [(1, 0.010119875), (4, 0.9501005)]
doc: 236 [(0, 0.48126006), (1, 0.012976307), (2, 0.012937998), (3, 0.01291139), (4, 0.46689728), (5, 0.013016945)]
doc: 237 [(0, 0.021024542), (1, 0.8949018), (2, 0.020991182), (3, 0.021095019), (4, 0.021017252), (5, 0.020970223)]
doc: 238 [(2, 0.39788073), (4, 0.5803694)]
doc: 239 [(2, 0.32005742), (3, 0.6642747)]
doc: 240 [(0, 0.11335334), (1, 0.6432129), (2, 0.012958464), (3, 0.012915699), (4, 0.012960928), (5, 0.20459871)]
doc: 241 [(0, 0.58403116), (3, 0.41068012)]
doc: 242 [(3, 0.6146098), (4, 0.37577277)]
doc: 243 [(0, 0.014007957), (1, 0.013999304), (2, 0.014036859), (3, 0.013946033), (4, 0.014057007), (5, 0.9299528)]
doc: 244 [(0, 0.4134391), (3, 0.5633509)]
doc: 245 [(0, 0.02402512), (1, 0.023970915), (2, 0.024024196), (3, 0.023925848), (4, 0.88001055), (5, 0.02404337)]
doc: 246 [(0, 0.010517221), (1, 0.0105254), (2, 0.01052896), (3, 0.010490651), (4, 0.010553944), (5, 0.9473838)]
doc: 247 [(1, 0.973687)]
doc: 248 [(0, 0.018665476), (1, 0.018711038), (2, 0.018699141), (3, 0.018616669), (4, 0.90644395), (5, 0.01886376)]
doc: 249 [(0, 0.01680894), (1, 0.016771415), (2, 0.016831433), (3, 0.016709587), (4, 0.91593224), (5, 0.016946342)]
doc: 250 [(0, 0.04217169), (1, 0.041880984), (2, 0.042052593), (3, 0.04177904), (4, 0.79009795), (5, 0.04201774)]
doc: 251 [(5, 0.9557194)]
doc: 252 [(0, 0.021046203), (1, 0.021063492), (2, 0.021002732), (3, 0.89483), (4, 0.021042898), (5, 0.02101467)]
doc: 253 [(0, 0.58099735), (1, 0.08369305), (2, 0.0839063), (3, 0.08356877), (4, 0.08409945), (5, 0.0837351)]
doc: 254 [(2, 0.715579), (3, 0.18125984), (5, 0.09084106)]
doc: 255 [(2, 0.52996373), (5, 0.43044698)]
doc: 256 [(0, 0.043813977), (1, 0.38615268), (4, 0.5611813)]
doc: 257 [(4, 0.9504558)]
doc: 258 [(4, 0.959994)]
doc: 259 [(1, 0.27219608), (3, 0.7192935)]
doc: 260 [(4, 0.9709992)]
doc: 261 [(0, 0.78919107), (1, 0.042261735), (2, 0.04214416), (3, 0.04203494), (4, 0.04230868), (5, 0.042059466)]
doc: 262 [(0, 0.021091476), (1, 0.02100511), (2, 0.020968145), (3, 0.020935662), (4, 0.021000907), (5, 0.89499867)]
From the result, we see that each document now is represented as a distribution of topics. For example, for doc: 0
, it is represented using topic 0, 1, 2, 3, 4, and 5, and their probabilities are 0.83217597, 0.033423353, 0.03352079, 0.03337131, 0.033680912, and 0.033827636, respectively. Since topic 1 dominates the distribution (with a probability of 0.83217597), we can then say doc: 0
(region indexed as 0) is more about “fast food” and “park” (this is from the topic distribution you get from the last step).
Since regions are also represented as, or can be converted to, numeric vectors. We can use the same semantic simiarity we implemented before to check the relevance between regions. Can you try it yourself?
Finally, to have a more straigtforward view of the model, we can use pyLDAvis
library, and the code is provided as below:
pyLDAvis.enable_notebook()
vis = gensimvis.prepare(lda_bristol, doc_term_matrix, dict_)
vis
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/_utils.py:38: DeprecationWarning: ast.Num is deprecated and will be removed in Python 3.14; use ast.Constant instead
if isinstance(node, ast.Num): # <number>
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/_utils.py:38: DeprecationWarning: ast.Num is deprecated and will be removed in Python 3.14; use ast.Constant instead
if isinstance(node, ast.Num): # <number>
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/_utils.py:38: DeprecationWarning: ast.Num is deprecated and will be removed in Python 3.14; use ast.Constant instead
if isinstance(node, ast.Num): # <number>
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/_utils.py:39: DeprecationWarning: Attribute n is deprecated and will be removed in Python 3.14; use value instead
return node.n
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/_utils.py:38: DeprecationWarning: ast.Num is deprecated and will be removed in Python 3.14; use ast.Constant instead
if isinstance(node, ast.Num): # <number>
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/_utils.py:39: DeprecationWarning: Attribute n is deprecated and will be removed in Python 3.14; use value instead
return node.n
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/joblib/externals/loky/backend/fork_exec.py:38: DeprecationWarning: This process (pid=61864) is multi-threaded, use of fork() may lead to deadlocks in the child.
pid = os.fork()
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/dateutil/tz/tz.py:37: DeprecationWarning: datetime.datetime.utcfromtimestamp() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.fromtimestamp(timestamp, datetime.UTC).
EPOCH = datetime.datetime.utcfromtimestamp(0)
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/dateutil/tz/tz.py:37: DeprecationWarning: datetime.datetime.utcfromtimestamp() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.fromtimestamp(timestamp, datetime.UTC).
EPOCH = datetime.datetime.utcfromtimestamp(0)
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/dateutil/tz/tz.py:37: DeprecationWarning: datetime.datetime.utcfromtimestamp() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.fromtimestamp(timestamp, datetime.UTC).
EPOCH = datetime.datetime.utcfromtimestamp(0)
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/dateutil/tz/tz.py:37: DeprecationWarning: datetime.datetime.utcfromtimestamp() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.fromtimestamp(timestamp, datetime.UTC).
EPOCH = datetime.datetime.utcfromtimestamp(0)
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/dateutil/tz/tz.py:37: DeprecationWarning: datetime.datetime.utcfromtimestamp() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.fromtimestamp(timestamp, datetime.UTC).
EPOCH = datetime.datetime.utcfromtimestamp(0)
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/dateutil/tz/tz.py:37: DeprecationWarning: datetime.datetime.utcfromtimestamp() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.fromtimestamp(timestamp, datetime.UTC).
EPOCH = datetime.datetime.utcfromtimestamp(0)
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/dateutil/tz/tz.py:37: DeprecationWarning: datetime.datetime.utcfromtimestamp() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.fromtimestamp(timestamp, datetime.UTC).
EPOCH = datetime.datetime.utcfromtimestamp(0)
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/dateutil/tz/tz.py:37: DeprecationWarning: datetime.datetime.utcfromtimestamp() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.fromtimestamp(timestamp, datetime.UTC).
EPOCH = datetime.datetime.utcfromtimestamp(0)
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/pyLDAvis/_prepare.py:9: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/pyLDAvis/_prepare.py:9: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/pyLDAvis/_prepare.py:9: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/pyLDAvis/_prepare.py:9: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/pyLDAvis/_prepare.py:9: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/pyLDAvis/_prepare.py:9: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/pyLDAvis/_prepare.py:9: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
/Users/gy22808/opt/anaconda3/envs/ox/lib/python3.12/site-packages/pyLDAvis/_prepare.py:9: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
This is an interactive visualization. On the left, each bubble represents a topic. The larger the bubble, the more prevalent or dominant the topic is. Good topic model will be fairly big topics scattered in different quadrants rather than being clustered on one quadrant. On the right, it shows the distribution of words of that topic. The red bar indicates the estimated frequency of the word in the topic; the blue bar indicates the overall term frequency (i.e., will not change over topics).
One key challenge of using LDA is to determine the parameter num_topics
, which can largely impact your model’s performance. There are some guidlines on selecting the optimal num_topics
:
To build many LDA models with different values of number of topics and pick the one that gives highest coherence score
coherence score is to measure the degree of semantic similarity between high scoring words in the topic. So if it is high, it means the top terms in the topic are semantically similar, which can further indicates that it is easier to interprete the topic
another evaluation metric is perplexity, which reflects how successfully a trained topic model predicts new data.
to get these two metrics, you can use codes as shown below.
If the same keywords have repeated in multiple topics, it’s probably a sign that the ‘k’ is too large.
If you are intersted in LDA for Place Semantics, check this paper: Gao, S., Janowicz, K., & Couclelis, H. (2017). Extracting urban functional regions from points of interest and human activities on location‐based social networks. Transactions in GIS, 21(3), 446-467. (if the link does not work, search it in UoB library!)
# Compute Perplexity
print('\nPerplexity: ', lda_bristol.log_perplexity(doc_term_matrix))
# a measure of how good the model is. lower the better.
# Compute Coherence Score
coherence_model_lda = CoherenceModel(model=lda_bristol, texts=corpus, dictionary=dict_, coherence='c_v')
coherence_lda = coherence_model_lda.get_coherence()
print('\nCoherence Score: ', coherence_lda)
Perplexity: -3.8607396997846375
Coherence Score: 0.37440290856540687